Although tuples are a fundamental data structure in Python, breaking them out into individual values can be difficult.
We’ll walk you through splitting a tuple in Python in this blog post. We’ll go through useful strategies and fixes, sophisticated methods, actual use cases, and frequent problems that could come up while splitting tuples.
You’ll have a firm grasp of the best practices for splitting tuples in Python by the end of this blog post. Hence, whether you’re a new or seasoned Python coder, read on to learn Python tuple splitting.
Let’s go!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
3 Practical Ways to Split Tuples in Python
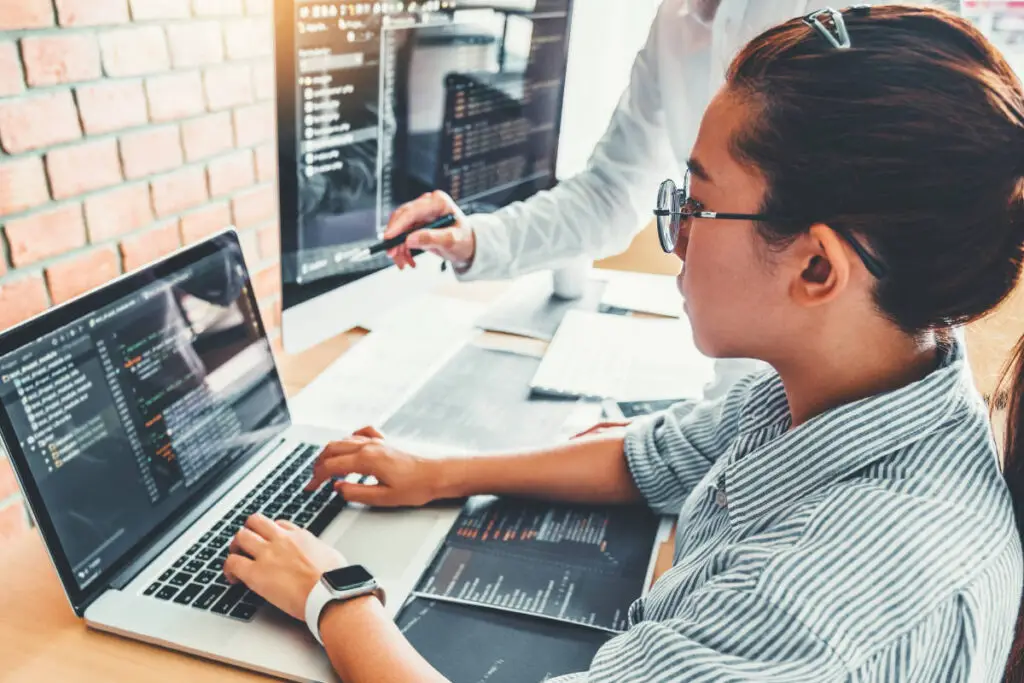
It’s possible that you’ll need to divide tuples into different values when working with them in Python. Fortunately, there are several useful approaches you can use.
Three of the most popular techniques will be covered in this chapter.
#1: Unpacking Operator: The Simplest Method
Using the unpacking operator is the most straightforward method of splitting a tuple. These values of the tuple, which Python automatically unpacks, can have variables attached to them thanks to this operator.
An illustration would be if you had a tuple with three values (1, 2, 3). The following formula can be used to divide this tuple into three different variables: a, b, and c = (1, 2, 3).
# Example tuple my_tuple = (1, 2, 3) # Unpack the tuple into separate variables a, b, c = my_tuple # Print the values of a, b, and c print(a) # Output: 1 print(b) # Output: 2 print(c) # Output: 3
Viola!
Currently, ´a´ is 1, b is 2, and c is 3.
#2: Extract a Certain Range with the Slice Operator
Using the slice operator is another method of splitting a tuple.
You can use this tool to find out the number of people who have viewed your profile.
How it functions is as follows: Suppose you have a tuple with five values (1, 2, 3, 4, 5). Use the slice operator with the following syntax to only retrieve the values from the second to the fourth index: my tuple[1:4].
Your new tuple with the values will be produced as a result (2, 3, 4).
# Example tuple my_tuple = (1, 2, 3, 4, 5) # Extract a specific range of values using the slice operator new_tuple = my_tuple[1:4] # Print the values of new_tuple print(new_tuple) # Output: (2, 3, 4)
#3: Nested Tuples: A Little Trickier
Tuples that contain other tuples are known as nested tuples, and you may occasionally need to separate them. Although a little more challenging, do not worry!
We can still use the strategies we discussed earlier.
Here is an illustration: Suppose you have a tuple that has the values (1, 2) and (3, 4) in two nested tuples. Using the unpacking operation twice, you can split this tuple as follows: a, b = ((1, 2), (3, 4)). As of right now, ´a´ is the tuple (1, 2) and b is the tuple (3, 4).
# Example tuple with nested tuples my_tuple = ((1, 2), (3, 4)) # Unpack the nested tuples into separate variables a, b = my_tuple # Print the values of a and b print(a) # Output: (1, 2) print(b) # Output: (3, 4)
You can extract particular values from your tuples and manipulate them as required by utilizing these useful methods for splitting tuples in Python.
2 Advanced Tuple Splitting Methods for Pros
If you’ve been using tuples in Python for some time, you may already be familiar with the fundamental methods for dividing them into distinct values.
But did you know that there are also some more sophisticated techniques out there?
This chapter will examine the zip() function and list comprehensions as two effective methods for tuple splitting.
Separating Tuples with the zip() Function
A versatile built-in Python method called zip() can be used for many things, including splitting tuples. By combining zip() with the unpacking operator, you can assign values to numerous variables at once, making your code more concise and legible.
Here is an illustration of how to split tuples using the zip() function:
# Example tuples names = ('Alice', 'Bob', 'Charlie') ages = (25, 30, 35) # Use zip() to split the tuples into separate variables for name, age in zip(names, ages): print(f"{name} is {age} years old.")
The names
and ages
tuples in this example each have three values.
We may separate the tuples into distinct variables for name
and age
by using the unpacking operator and the zip() method. Then, using f-strings, for loop prints out a text after iterating over each pair of variables.
Simple and Effective List Comprehension Techniques
Python’s list comprehensions are a quick and effective technique to build lists. By applying an expression to each element in an existing list or iterable, you can use them to generate a new list.
But did you know that dividing tuples may also be accomplished with list comprehensions?
You can take a tuple and turn it into a new list of values by using a list comprehension and the unpacking operator.
Here is an illustration of how to split tuples using list comprehensions:
# Example tuples names = ('Alice', 'Bob', 'Charlie') ages = (25, 30, 35) # Use zip() to split the tuples into separate variables for name, age in zip(names, ages): print(f"{name} is {age} years old.")
The tuple my_tuple
in this illustration has five values. We can make a new list new_list
with the same values as the initial tuple by using a list comprehension and the unpacking operator. Each value in the tuple is simply returned by the expression x.
The updated list is then printed using an f-string.
You may make your code more succinct, readable, and potent by utilizing these sophisticated tuple splitting in Python strategies.
So feel free to give them a shot!
Common Errors and How to Prevent Them
Even seasoned developers might make some typical errors while splitting tuples in Python, despite the fact that it can be a straightforward process. We’ll discuss a few of the most typical errors in this chapter, along with suggestions for how to avoid them.
Forgetting the Unpacking Operator
Forgetting to utilize the unpacking operator is one of the most typical errors made while dividing tuples. Python will try to assign the entire tuple to a single variable if the unpacking operator is not present, which could provide unexpected results.
Take a tuple with two values as an illustration (1, 2). Without using the unpacking operator, if you try to assign this tuple to a single variable using the syntax my var = (1, 2), the result will be a tuple with two values.
But if you apply the unpacking operator, as in the formula a, b = (1, 2), a and b will both be 1.
Always remember to separate tuples with the unpacking operator to prevent making this common error.
Choosing the Incorrect Index or Slice
When dividing tuples, using the incorrect index or slice is a typical error.
When working with large or complex tuples, you can experience this if you forget which values you’re attempting to access.
Let’s imagine you have a tuple with five values, for instance (1, 2, 3, 4, 5). Since there are only five entries in the tuple, an IndexError will be returned if you attempt to access the value at index 6, as in my_tuple[6].
Before utilizing your indices and slices to retrieve tuple values, double-check them to prevent making this common error.
Not Taking Nested Tuples Into Consideration
When working with tuples that contain other tuples, or nested tuples, it can be simple to overlook the hierarchical structure while dividing them.
Let’s take the example of a tuple containing two nested tuples, ((1, 2), (3, 4)). Without considering the hierarchical structure, you can get surprising results if you try to split this tuple.
Use the unpacking operator for each level of nesting in your tuples to prevent making this common error.
You may improve your Python tuple splitting skills by being aware of these common errors and taking precautions to prevent them.
Use Cases in the Real World for Python Tuple Splitting
Let’s examine some practical use scenarios where tuple splitting might be extremely helpful after covering the fundamentals and some advanced methods for splitting tuples in Python.
Tuple splitting can make it easier for you to work with your data more effectively and efficiently, from data analysis to string manipulation.
Data Analysis and Manipulation
Tuples are frequently used in data analysis and manipulation to represent rows of data in a table or database. You can do calculations and data manipulations on particular values by dividing these tuples into independent variables without impacting the rest of the data.
An illustration of how to divide tuples in a list of data is given here:
# Example data my_data = [ ('Alice', 25, 'F'), ('Bob', 30, 'M'), ('Charlie', 35, 'M'), ] # Split the data into separate variables for name, age, gender in my_data: print(f"{name} is {age} years old and identifies as {gender}.")
In this illustration, each tuple in the list my data stands for a row of data. We may assign each value to a different variable and then print out a line about each person by utilizing tuple splitting along with the unpacking operator.
String Manipulation
Tuples can be used to represent smaller strings within a bigger string when manipulating strings. These tuples can be divided into several variables so that you can interact with the string’s individual characters more easily.
An illustration of how to partition a string into tuples and then those tuples into different variables is shown below:
# Example string my_string = "Hello, World!" # Split the string into tuples of (letter, index) my_tuples = [(letter, index) for index, letter in enumerate(my_string)] # Split the tuples into separate variables for letter, index in my_tuples: print(f"{letter} is at index {index}.")
In this example, we break the string into tuples of (letter, index), where letter
is a single letter from the string and index
is its location in the string, first using a list comprehension.
After assigning each value to a different variable using tuple splitting and the unpacking operator, we print out a sentence for each letter.
Object-Oriented Programming
Tuples can be used in object-oriented programming to represent an object’s state. These tuples can be divided into many variables so that you can work with the object’s specific attributes more easily.
Here is an illustration of how to partition a tuple expressing the state of an object into several variables:
# Example class class Person: def __init__(self, name, age, gender): self.name = name self.age = age self.gender = gender def get_state(self): return (self.name, self.age, self.gender) # Create a new Person object p = Person("Alice", 25, "F") # Get the object's state as a tuple and split it into separate variables name, age, gender = p.get_state() # Print out the object's attributes print(f"{name} is {age} years old and identifies as {gender}.")
The Person class in this example has attributes for name, age, and gender, and its get state() method delivers these data as a tuple.
The next step is to construct a new Person object, p, and assign each value to a different variable using tuple splitting and the unpacking operator. Lastly, a statement describing the object is printed.
Common Questions
What is tuple in Python?
A tuple is an immutable, ordered sequence of values in Python. Although they are similar to lists, multiples cannot be changed once they have been formed. When storing related values collectively, such as a pair of coordinates or a date and time, tuples are frequently employed.
Can you slice a tuple in Python?
In Python, the slice operator can be used to slice a tuple. A tuple can be divided into smaller pieces, each of which includes a specific set of values from the larger tuple.
Can tuples be split using split()?
No, Python’s split() function does not support tuples. A string can be divided into a list of substrings using the split() function, which does so based on a delimiter that is given. The split() method does not exist for tuples, which are immutable.
How do you slice a tuple in a list in Python?
In Python, list indexing and tuple slicing can be combined to slice a tuple within a list. You can use the following code, for instance, to extract the second value from the first tuple if you have a list of tuples called my list. my_list[0][1:].
Conclusion
In this article, we’ve examined both the fundamentals and some more sophisticated methods for dividing tuples in Python, such as the use of nested tuples and the unpacking and slice operators. Along with tuple splitting’s practical applications in data analysis, string manipulation, and object-oriented programming, we’ve talked about some typical pitfalls to avoid while working with tuples.
You may interact with your data more effectively and efficiently while also making your code simpler to read and maintain if you learn Python’s tuple splitting technique. It’s a good idea to have a backup plan, just in case.
Like with any programming subject, there is always more to discover. For this reason, we urge you to keep researching tuple splitting in Python and attempting novel approaches and use cases. You can split tuples like a pro with a little effort and experimentation.
Happy coding 😎.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.