As a programmer, you know how frustrating it can be to spend hours debugging code, only to find that the problem was caused by a simple mistake, such as a repeated string.
Repeated strings can have a significant impact on program performance and memory usage, and finding them quickly and efficiently is essential for any programmer.
In this blog post, we’ll explore how Python can be used to find repeated strings step by step. Whether you’re a beginner or an experienced programmer, this guide will help you improve your coding efficiency and avoid common mistakes.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What are repeated strings?
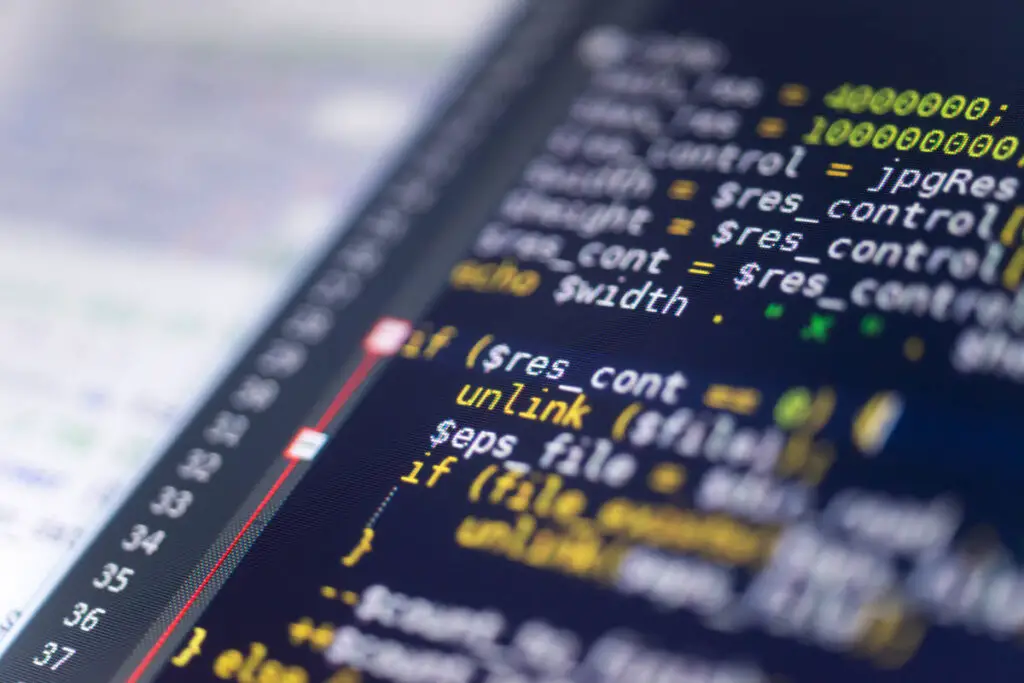
Repeated strings are those that turn up multiple times in a dataset. They can appear in text, images, and audio, among other sorts of data.
When processing through a text file and the same word or phrase appears more than once, this is a typical example of a repeated string.
Another illustration is when two datasets are compared to identify common elements; repeating strings can signify matches between the databases.
Why is it important to find repeated strings?
Identifying repeated strings in your data is crucial for several reasons.
First, it can have a significant impact on program performance. When working with large datasets, repeated strings can slow down your code and increase memory usage, leading to longer processing times and potential errors. By identifying and removing repeated strings, you can streamline your code and improve its overall efficiency.
Second, finding repeated strings can help improve the accuracy of your code. In some cases, repeated strings may indicate errors or duplicates in your data, leading to inaccurate results.
By identifying and removing repeated strings, you can ensure that your code is working with accurate data and producing reliable results.
Overall, finding and removing repeated strings is an essential step in any data analysis or programming project. In the next section, we’ll explore how you can use Python to find repeated strings step by step.
How to find repeated strings in Python in 5 steps
Python has a number of built-in modules and functions that can be used to find recurrent strings in your data.
Here is a step-by-step tutorial for using Python to find repeated strings:
Step 1
Put your information in a list or string object that Python can read as a string format.
Python needs string objects to execute string-specific operations like searching and counting. Thus, this step is required. You must first convert your data into a string format if it is in another format, such as a database table or a numerical array.
Step 2
Create an empty dictionary object to keep track of the number of strings. A dictionary in Python is a group of key-value pairs, where each key denotes a distinct element in the group and the matching value denotes the element’s count.
In this instance, we’ll utilize the dictionary to keep track of how many times each string appears in our data.
Step 3
Add each string to the dictionary using a loop through your data, increasing the count for each occurrence.
The current count of each element in your data can be obtained by using the dictionary’s get() method while iterating through each element in your data using a for loop.
The get() method will return a default value of zero if the element is not yet included in the dictionary. The count can then be increased by one and stored in the dictionary with the element serving as the key.
Step 4
Make a fresh dictionary object that only contains repeated strings with a count greater than 1 in it.
To achieve this, iterate through each key-value pair in the original dictionary using a different for loop, and determine whether the count is larger than 1.
If this is the case, you can add the key-value pair to a brand-new dictionary object that just contains the repeated strings.
Step 5
Print the repeated strings together with their number. To output the repeated strings and their associated count, use the print() statement.
This phase is crucial to make sure your code is operating properly and to find any potential mistakes or problems.
Here is an example of Python code that implements these steps:
# example data data = ['apple', 'orange', 'banana', 'apple', 'orange', 'grape'] # create an empty dictionary to store string counts string_count = {} # loop through the data and add each string to the dictionary for string in data: count = string_count.get(string, 0) string_count[string] = count + 1 # create a new dictionary to store only the repeated strings repeated_strings = {} for key, value in string_count.items(): if value > 1: repeated_strings[key] = value # output the repeated strings print("Repeated strings:") for key, value in repeated_strings.items(): print(f"{key}: {value}")
Practical applications of finding repeated strings
Discovering repeated strings can be used in a variety of real-world programming and data analysis scenarios. Here are a few illustrations:
- Text analysis: Recurring strings can point to frequently occurring phrases or words in a collection of texts. You can learn more about the most prevalent subjects or themes in the text by spotting these recurring phrases, and you can then utilize this knowledge to enhance search algorithms or predictive models.
- Recurring strings can also be found in picture databases, where they may point to recurring patterns or shapes. You can enhance picture recognition algorithms or spot anomalies in the collection by locating these repeated strings.
- Audio analysis: Repeating strings in audio files can be utilized to find musical or spoken patterns. These repeated strings can be used to enhance voice recognition software or find recurring musical themes in songs.
Generally, the process of identifying repeated strings is essential to many programming and data processing tasks.
You may enhance the precision and effectiveness of your code and discover important information about your data by using Python to rapidly and effectively identify these strings.
The key ideas of this manual are summarized in the next part, along with some recommendations for readers’ next moves.
Conclusion
We’ve covered Python’s repeated string search in this article. We’ve highlighted the necessity of recognizing repeated strings in your data and offered a full Python approach to do so.
We’ve also addressed practical applications for identifying repeated strings in text, pictures, and audio.
The good news are: Python makes it easy to find repeated strings in your data. .
Explore Python packages and tools that ease identifying repeated strings to expand your skills. Counter, pandas, and nltk are common choices.
Programmers and data analysts need to find repetitive strings. This guide will help you find and remove repetitive strings in your data to enhance programming productivity and accuracy.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.