Do you wish to shield unwanted users and prying eyes from your Python code? Do you want to stop anyone from stealing your ideas or reversing engineering your code?
If so, you should become familiar with the potent method of code obfuscation. Obfuscation is the process of keeping functionality but making your code more difficult to read and understand.
Any programmer who wishes to keep their work secure and confidential must possess this ability. This blog post will walk you through the most effective techniques for hiding your Python code, including the usage of external tools.
You will have the knowledge, skills, and confidence to use them on your projects at the end of this post to keep your code safe and secure. So let’s get started and discover how to disguise Python code expertly!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Why you should obfuscate your Python code…
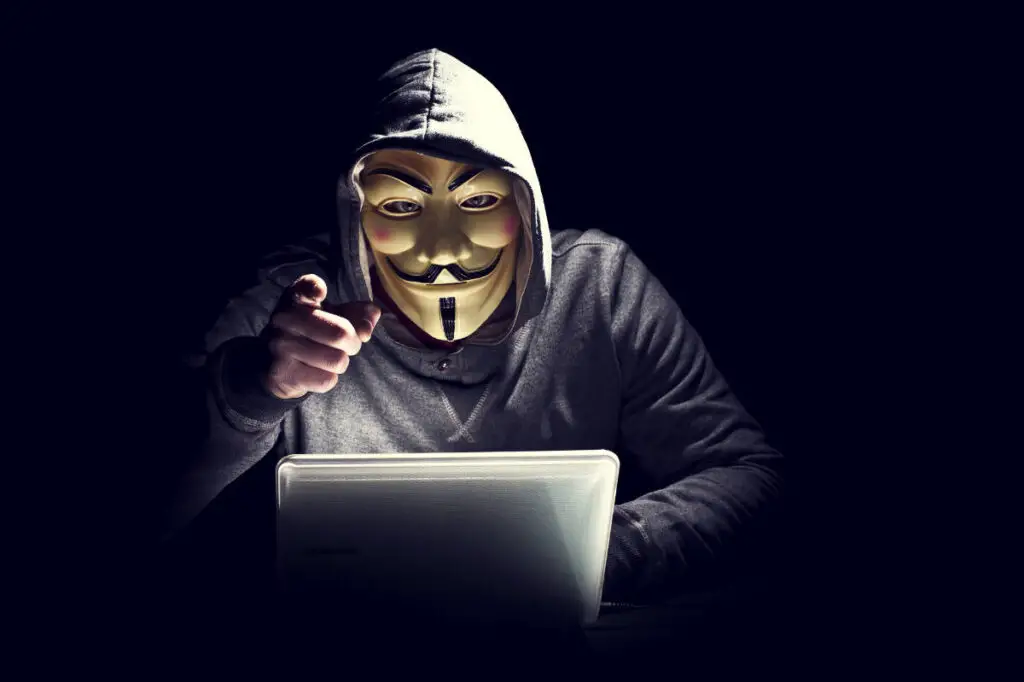
Obfuscating code is a crucial method that all programmers should be familiar with.
The explanation is straightforward: anyone with access to your code may read and understand it if it is not obfuscated.
This includes possible competitors and hackers who might use your code to steal your intellectual property, obtain illegal access to confidential data, or reverse-engineer your products.
Obfuscation, however, has uses beyond security. You may enhance the readability and comprehension of your code, while also increasing its efficiency. This is because obfuscation can assist your code become smaller, more effective, and more quickly executable.
For applications that demand high performance or have constrained resources, this can be particularly crucial.
Code obfuscation is a crucial ability for any programmer who wants to safeguard their code and make sure it runs as efficiently as possible. We’ll look at some top methods for obscuring Python code in the following section.
Now let’s get started and learn how to safeguard and protect your code 😎!
Python Code Obfuscation: 4 Methods
There are various methods for obscuring Python code that you might employ to make it more challenging to read and comprehend. Let’s examine each of these strategies in greater detail and see how to use them in practice:
#1: Name Mangling
Name mangling is the process of renaming variables and functions in your code to make them more illegible. You can use underscores before or after the names of your variables and functions to use this strategy. For example:
class MyClass: def __init__(self): self.__my_private_var = "Hello World" def get_private_var(self): return self.__my_private_var my_instance = MyClass() print(my_instance.get_private_var())
In this illustration, the variable my private var’s name has been changed.
This makes it more difficult for others to determine the variable’s function and the class to which it belongs.
#2: Restructuring the Code
Rearranging your code to make it more challenging to read and comprehend is known as code restructuring. Using intricate control structures, like as nested loops and conditionals, is one frequent way to implement this strategy.
For instance:
def complex_function(a, b): for i in range(a): for j in range(b): if i % 2 == 0: print("Hello") else: print("World")
In this example, we’ve used code restructuring to build a function that prints alternating strings using nested loops and conditionals.
As a result, the logic of the function is concealed behind intricate control structures, making the code more challenging to read and understand.
#3: Bytecode Manipulation
Modifying the generated code that Python creates from your source code is known as bytecode manipulation.
This method has the potential to be quite effective, but it necessitates thorough knowledge of the Python virtual machine and how it executes bytecode.
Replace code blocks with comparable but obfuscated blocks, insert dummy code blocks to make the code more illegible, and change the control flow of the code are a few examples of typical bytecode modification techniques.
For instance:
def obfuscated_function(a, b): if a < b: return a + b else: return a - b
The function in this example tests to see if an is less than b via bytecode modification.
The function returns the sum of a and b if it is. The function returns the difference between a and b if it is not.
We can obscure the control flow of the code and make it more challenging to comprehend and follow by employing bytecode manipulation.
#4: Third-Party Tools
Python code can be obscured using a variety of third-party tools. These tools offer a variety of approaches to make your code more challenging to comprehend and implement, as well as the ability to automate the obfuscation process. Let’s examine some of the most well-liked tools in more detail:
PyArmor
PyArmor is a potent obfuscation tool that hides your Python code using a variety of methods. It offers capabilities including name mangling, code reorganization, and bytecode encryption.
Additionally, PyArmor has a license system that enables you to manage who can use your code that has been obfuscated.
from pyarmor.pyarmor import obfuscate_file # Obfuscate a file and generate a new obfuscated file obfuscate_file("my_script.py", "my_obfuscated_script.py", "my_license.lic", mode="eval")
Python-Minifier
Python-Minifier is a simple program that aims to reduce the size of your code by eliminating comments and extra whitespace.
To make your code more difficult to read, it can also rename variables and functions.
Python-Minifier is a decent option if you just want to shrink the size of your code, even though it doesn’t offer the same amount of obfuscation as other tools.
from python_minifier import minify # Minify a file and print the obfuscated code to the console obfuscated_code = minify("my_script.py", rename_globals=True) print(obfuscated_code)
Pyminifier
Another simple tool you may use to obfuscate your Python code is Pyminifier. It offers functions like variable renaming, comment removal, and whitespace removal.
Pyminifier is helpful for bigger projects because it can manage several files at once.
Ultimately, using third-party tools to obfuscate your Python code can be practical. These tools offer a variety of strategies and functions to make your code more challenging to read and comprehend.
It’s crucial to carefully analyze your alternatives and pick the solution that best suits your needs, whether you decide to use PyArmor, Python-Minifier, Pyminifier, or another program.
from pyminifier import minify # Minify a file and write the obfuscated code to a new file with open("my_obfuscated_script.py", "w") as f: f.write(minify("my_script.py", remove_comments=True, rename_locals=True))
Python Code Obfuscation Best Practices
Python code obfuscation is a practical method for safeguarding your intellectual property and preventing code reverse engineering.
To retain the effectiveness and maintainability of your obfuscated code, it’s crucial to adhere to a few recommended practices. The following are some recommendations to remember:
- Employ a variety of methods: Using a variety of obfuscation methods in tandem can help your code be as effective as possible. To make your code more challenging to comprehend and follow, you can, for instance, utilize name mangling, code restructure, and bytecode manipulation.
- Test your obfuscated code: Before deploying your obfuscated code, make sure it functions as intended by giving it a full test run. Checking to make sure your code still functions properly after obfuscation is critical since obfuscation can occasionally bring flaws or unexpected behavior.
- Consider the tradeoffs: Obfuscation might make your code more challenging to read and comprehend, but it can also make it more challenging to maintain and update. It’s crucial to thoroughly weigh the tradeoffs between maintainability and obfuscation before obfuscating your code.
- Employ third-party tools: Using third-party tools to obfuscate your code is a convenient approach to make it harder to read and understand (see the three solutions in the chapter before). They frequently offer a variety of features and techniques to do this. Nonetheless, it’s crucial to pick your tool wisely and be aware of its constraints.
- Maintain a clean copy: You should keep a copy of your code that hasn’t been obfuscated as a backup in case something goes wrong with the obfuscation process or if you need to update your code in the future.
These best practices can help you safeguard the effectiveness and maintainability of your obfuscated code, while also safeguarding your intellectual property and preventing others from deciphering your code.
Conclusion
Protect your intellectual property by obfuscating your Python code.
You can make your code harder to comprehend and follow by employing name mangling, code restructure, bytecode modification, and third-party tools. Unfortunately, obfuscation is not failsafe, and determined attackers may still be able to reverse-engineer your code.
Obfuscated code can be harder to maintain and update. Thus, before using obfuscation in your applications, consider its pros and cons.
Obfuscation can effectively safeguard Python code. You may choose the appropriate obfuscation method for your needs and use case by learning the various methods and tools.
Best practices include testing your obfuscated code and retaining a backup copy of your unobfuscated code. By adopting these recommended practices, you can secure your intellectual property and keep your obfuscated code functional and maintainable.
Happy coding 😎.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.