Everyone is aware of Python’s renown for having robust standard libraries. However, in most other programming languages, we will need to implement numerous functionality piecemeal.
The majority of the fancy features, on the other hand, are already implemented in Python and are available for usage right out of the box.
In this article, find out how to create a list in Python and what are some of the wrapping techniques that you can find in Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
How To Create A List In Python?
You may make an empty list by using two square brackets:
>>> empty_list = [] >>> type(empty_list) <class 'list'> >>> len(empty_list) 0
A comma-separated list of components can be converted into a list by enclosing it in square brackets.
Although lists can contain components of several sorts (heterogeneous), they typically have only one type (homogeneous):
>>> homogeneous_list = [1, 1, 2, 3, 5, 8] >>> type(homogeneous_list) <class 'list'> >>> print(homogeneous_list) [1, 1, 2, 3, 5, 8] >>> len(homogeneous_list) 6 >>> heterogeneous_list = [1, "Hello Campers!"] >>> print(heterogeneous_list) [1, "Hello Campers!"] >>> len(heterogeneous_list) 2
A list can also be made using the list constructor:
>>> empty_list = list() # Creates an empty list >>> print(empty_list) [] >>> list_from_iterable = list("Hello campers!") # Creates a list from an iterable. >>> print(list_from_iterable) ['H', 'e', 'l', 'l', 'o', ' ', 'c', 'a', 'm', 'p', 'e', 'r', 's', '!']
Wrapping Techniques You Can Find In Python
Now we are onto the good part!! Let’s learn how to use some techniques that you already have in Python!
You will learn about two wrapping methods. Let’s go!
Wrapping In Python: Basics
Nothing needs to be installed. Instead, a simple import of the library is required.
import text-wrap as tr
my_str = "Python is an interpreted, high-level and general-purpose programming language."
My str is a lengthy sentence. The text needs to be shown in a specific width on your website or in the user interface of your software.
The text must be divided into numerous lines if it is wider than tall.
Of course, we don’t want to divide it perfectly into segments of length and width because that could result in some words being partially at the end of one line and the beginning of the next.
From the user’s point of view, reading this is not enjoyable.
lines = tr.wrap(my_str, width=30)

The sentence is divided into three parts, as seen. This is because we set the width to 30, which ensures that the text won’t “overflow” in the UI before it reaches 30. The lengths of the three parts are 25, 30, and 21 when we count them.
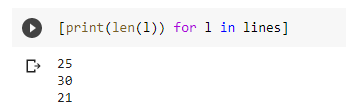
Because of this, Python TextWrapper is intelligent enough to recognize that the following word will cause the current line to overflow and will thus automatically move it to the next line. We have 25 characters in the first line because of this.
Wrapping In Python: Ready To Print
This still isn’t convenient enough because we have to loop the list in order to print the wrapped strings line by line.
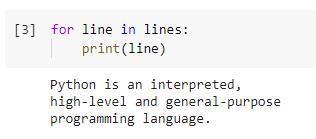
Yes, Python specifically included the fill() method to make things more convenient.
Instead of using tr.wrap() on the exact string, we can use tr.fill() to obtain a single wrapped string with n within that is “print-ready.”
tr.fill(my_str, width=30)
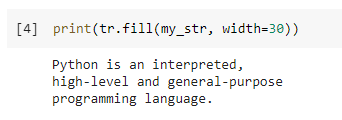
There are more wrapping techniques you can try, here are just some of them.
If you want to see the answers to some of the most asked questions below, make sure to keep reading!
FYI – all the pictures are taken from an author Christopher Tao.
Frequently Asked Questions
Here are the most asked questions online on a topic of wrapping around a list in Python.
How Can I Wrap Text In A List In Python?
Use Python’s textwrap module from the standard library to wrap or truncate strings at a specified width (= number of characters).
In Python, Are List () and [] The Same?
Practically, there is no distinction.
Given that [] does not require a global lookup followed by a function call, we would say for [] to be faster.
It remains the same except for that.
A Tuple Vs. List: What Is The Difference?
The main distinction between tuples and lists is that although lists are mutable, tuples are immutable objects.
This indicates that while lists can be updated, tuples cannot. Additionally, multiples use memory more effectively than lists do.
Hopefully, you now know how to wrap around a text in Python! Yay!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.