A global variable in Python is defined outside the function or in the global scope.
This indicates that a global variable may be accessed inside or without the function. But, we will talk more about the definition in the article.
In this article, find out what a global variable is, how to clear out the variables, and how to reset the global variables differently.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is a Global Variable?
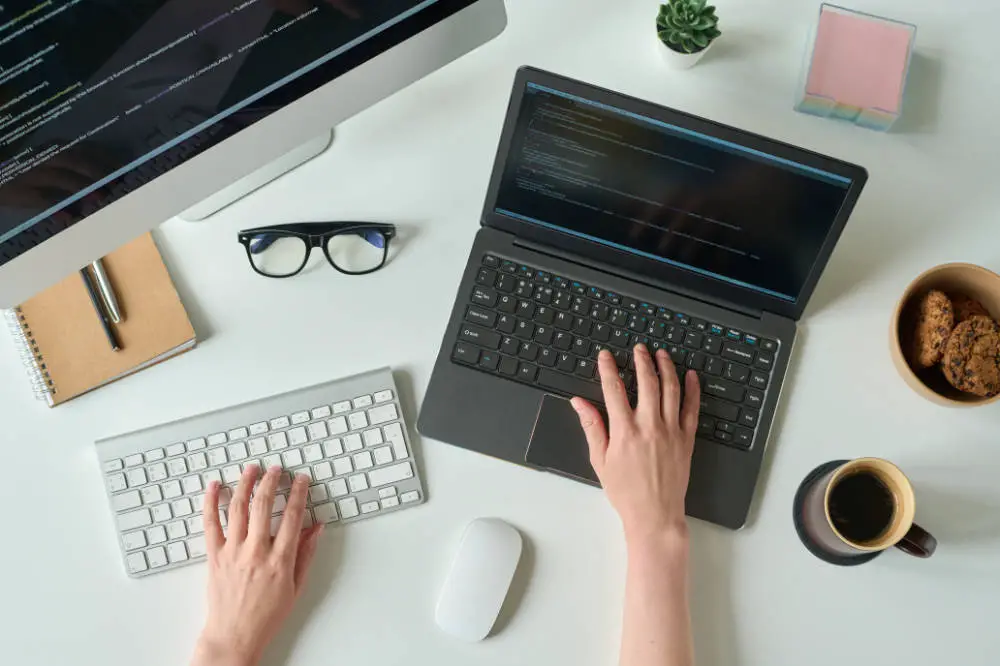
A global variable is a programming language concept.
It is a variable declared outside any function and available to all functions throughout the program.
Because they describe many characteristics of a program or the environment while the program runs, a collection of global variables is referred to as a global state or global environment.
Most programmers consider global variables risky since they can be mistakenly altered, leading to problems during program execution; hence they are often declared on top of all functions and kept to a minimum.
Can You Change a Global Variable in Python?
You can access a global variable inside the function and get an output. But, there are scenarios where you have to get inside the function and change it from the inside.
If you do that, you will encounter an error. It often looks something like this:
UnboundLocalError: local variable ‘?’ referenced before assignment
That is because you can access it but cannot change it from the inside. You can use the global keyword for this. Then you will have the needed output.
How To Reset Variables in Python?
There are several methods how for clearing the variables in Python.
You can use the del option, the %reset-f, or the %reset.
Let’s explain them quickly.
Clearing the Variables: Del
Deleting a variable in Python is as simple as typing del.
You may clear variables quickly and easily using the del function in Python.
To remove variable1, utilize it as follows:
del variable1
Use it as follows if you wish to remove many variables:
del variable1, variable2
As a result, you can eliminate the particular variable you do not want.
Clearing the Variables: %reset-f
Same as above, there is a way of clearing the variables using the reset-f option.
Simply use the code:
%reset-f
On the other hand, the %reset option is similar to the one above.
Global Variables: 3 Ways on How to Reset Them
In this section, you will see three representations of how to reset the global variable in Python.
Use these methods on your computer, and let us know how it went!
Reset Global Variable #1 (h3)
When reading a variable, Python searches the full scope chain for it. This implies:
GLOB_VAR = "Some thing"
def some_dance():
print GLOB_VAR
You must explicitly inform Python that you are referring to an existing global variable if you want to write to your global. It would be best if you employed the global keyword to do this. Thus, the following.
GLOB_VAR = "Some thing"
def some_dance():
global GLOB_VAR
GLOB_VAR = "Some other thing"
some_dance()
print GLOB_VAR
When you attempt to read from a global first before writing to it, the behavior is the same (but slightly more unexpected). These things:
GLOB_VAR = False
def some_dance():
if GLOB_VAR:
GLOB_VAR = False
some_dance()
Next, this will arise:
Traceback (most recent call last):
File "t.py", line 7, in <module>
some_dance()
File "t.py", line 4, in some_dance
if GLOB_VAR:
UnboundLocalError: local variable 'GLOB_VAR' referenced before assignment
The interpreter must be informed that you are referring to a global variable:
def some_dance:
global SOME_VARIABLE
...
SOME_VARIABLE = []
If you no longer require the SOME VARIABLE, you might use:
del SOME_VARIABLE
To get an empty list:
del SOME_VARIABLE[: ]
Since SOME_VARIABLE is a global variable, rebinding it will not work unless you use global. However, modify it as necessary since it’s a changeable object.
Reset Global Variable #2
Now let’s explain the second example.
The RESET GLOBAL command removes the names and values of variables an administrator or user generates.
These names do not start with “DSQ” and are global variables.
To remove all previously specified names and values for global variables made by an administrator or user: GLOBAL ALL RESET, Only the DEPT, and LOCATION variables’ names and values should be removed: GLOBAL RESET (DEPT LOCATION.
You can enter the names of the variables you wish to remove on the question window that appears when you perform RESET GLOBAL?
RESET global variables
. - + -- - + -- -- -- -- -- --. |
'-,-' |
V |
>>
-RESet--GLOBAL-- + -(-- -- - name-- - + - + -- - + - + -- -- -- -- -- -- - > <
|
'-)-' |
'-ALL--------------------------'
RESET GLOBAL ALL
RESET GLOBAL(DEPT LOCATION
Reset Global Variable #3
Variables that are only used inside of functions are inherently global in Python.
Anywhere in the function’s body where a variable is given a value unless specified explicitly as global, it is presumed to be local.
This explicit declaration is necessary to remind you that you are genuinely changing the value of the variable in the outer scope rather than the comparable scenario with class and instance variables: Guido van Rossum advises storing all code within functions rather than using any instances of from <module> import.
Global and class variables should only be initialized using built-in functions or constants.
This indicates that everything from an imported module is called <module>. <name>.
Programming practice is recommended to avoid using mutable objects as default values due to this property.
Use None as the default value instead, and if the argument is None inside the function, construct a new list, dictionary, or whatever if it is. Don’t write, for instance:
>>> x = 10 >>>
def bar():
...print(x) >>>
bar()
10
>>> x = 10 >>>
def foo():
...print(x)
...x += 1
>>> foo()
Traceback(most recent call last):
...
UnboundLocalError: local variable 'x'
referenced before assignment
>>> x = 10 >>>
def foobar():
...global x
...print(x)
...x += 1 >>>
foobar()
10
>>> print(x)
11
>>> def foo():
...x = 10
...def bar():
...nonlocal x
...print(x)
...x += 1
...bar()
...print(x) >>>
foo()
10
11
Conclusion
There you go! Now you know how to reset a global variable in Python; how exciting!
Let us know how it goes, and share your own tips&tricks!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.