Any programming language, even Python, needs to have a printing feature. When you need to format data in a certain way, being able to print results horizontally can be useful. This article will explain how to print horizontally in Python and give you the resources and information you need to accomplish it well.
Don’t worry: we know that printing horizontally in Python can seem like a difficult undertaking. We will deconstruct the subject into simple steps and offer applicable examples along the way.
Regardless of your level of programming knowledge, this blog article will provide insightful tips and tricks that will help you increase your coding abilities.
You will be prepared with the knowledge and confidence necessary to print horizontally in Python by the end of this blog post. Now let’s get started and enhance your coding skills!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
In a nutshell: How to Print Horizontally in Python
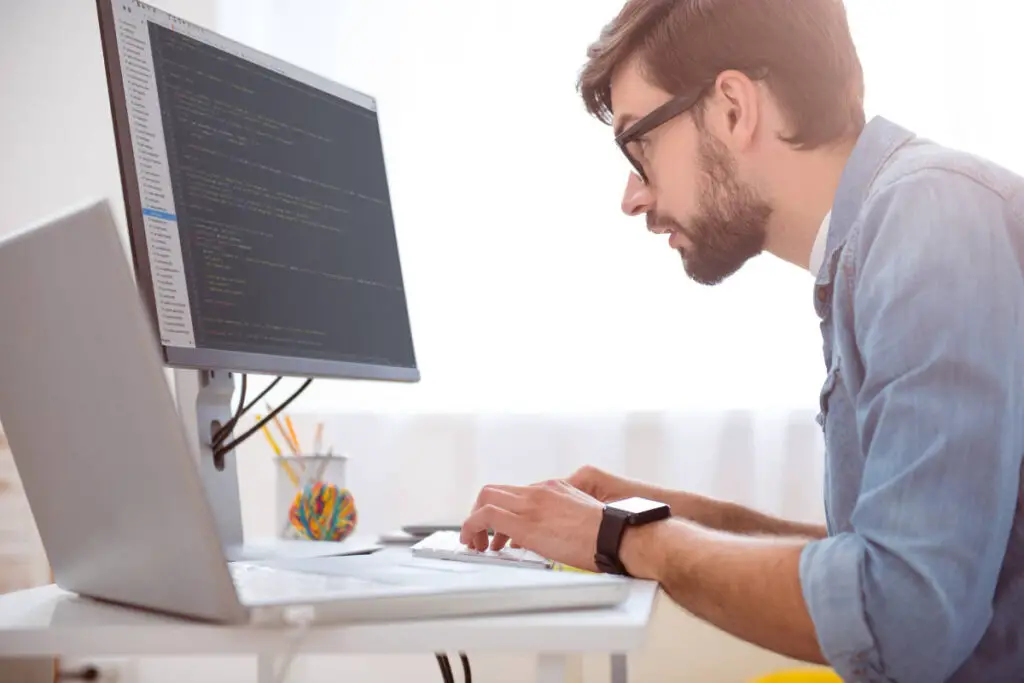
Use the “end” parameter with the print() function in Python to print horizontally. The end argument is set by default to “\n,” which writes the output on a new line. The result can, however, be printed horizontally by changing the value of the end parameter to anything you choose, such as an empty string or a space character. In addition, you can horizontally print concatenated strings by using the join() method. With these methods, printing horizontally in Python is simple, and your output may be formatted however you like.
Effective advice for printing horizontally in Python
Here are some helpful hints for horizontal Python printing:
Using the print() function’s “end” parameter
Using the “end” parameter with the print() method is a simple way to print horizontally in Python. The “end” parameter’s default value of “n” prints the result on a new line.
Nevertheless, you can modify the value of the “end” option to anything you choose, even an empty string or a space character, to print the output horizontally.
# Example 1: Print horizontally with space print("Hello", end=" ") print("World") # Output: Hello World # Example 2: Print horizontally without space print("Hello", end="") print("World") # Output: HelloWorld
Making use of the join() technique
Another excellent method for horizontally printing concatenated strings is the join() method.
The join() method can be used to print a list of strings on a single line. For example, if you have a list of words, you can use the join() method to print them horizontally like this: “.join(words)” is printed.
# Example 1: Join a list of words with space and print horizontally words = ["Hello", "World"] print(" ".join(words)) # Output: Hello World # Example 2: Join a list of words without space and print horizontally words = ["Hello", "World"] print("".join(words)) # Output: HelloWorld
Loops
To print output horizontally, you can use loops like for and while loops. For instance, you could print each item in a list horizontally using a loop.
A row of asterisks or dashes, for example, can be printed horizontally using loops.
# Example 1: Print a list of numbers horizontally using a for loop numbers = [1, 2, 3, 4, 5] for num in numbers: print(num, end=" ") # Output: 1 2 3 4 5 # Example 2: Print a row of asterisks horizontally using a while loop count = 0 while count < 5: print("*", end="") count += 1 # Output: *****
Using formatting options
To print output horizontally, Python provides a variety of formatting choices. For instance, you can format a string with variables and output them horizontally using the format() method.
The f-strings (formatted strings) can also be used to print output horizontally in a cleaner way.
# Example 1: Format a string with variables and print horizontally name = "Alice" age = 25 print(f"My name is {name} and I'm {age} years old.") # Output: My name is Alice and I'm 25 years old. # Example 2: Format a string with a specific width and print horizontally number = 42 print(f"Number: {number:05}") # Output: Number: 00042
Consider breaking up the process of horizontal printing in Python into smaller phases to make it even simpler.
Choose the best method to print something horizontally after first identifying the output you want to print. As you are proficient with each technique, experiment with combining them to produce more complicated results.
Solving typical problems
Although Python allows for horizontal printing, there are a few frequent issues that can occur. Listed below are a few potential problems and how to fix them:
- If you use the print() function and fail to specify the value for the “end” parameter, extra space may appear at the end of the line. Make sure you set the value of “end” to an empty string to resolve this problem. For example: print(“Hello”, end=”); print(“World”).
- Improper item spacing can happen if the join() technique is used with the incorrect delimiter. Use the correct delimiter for your output. For instance, use “, ” as the delimiter to divide items with a comma and a space.
- Too much output: If your output is too long to fit on one line, you might need to divide it up into several lines. The “\n” character, which creates a line break, can be used to accomplish this. Consider this: For readability, this sentence needs to be broken up into many lines, (“This is a really long sentence that needs to be split\nup into multiple lines for readability.”)
- When utilizing f-strings or the format() technique, formatting issues can happen. Be sure you use the appropriate formatting and syntax. For example, if you want to print a float with two decimal places, use “{:.2f}”.format(my_float) or f”{my_float:.2f}”.
You can make sure that your output is correctly formatted and printed horizontally by resolving these typical issues.
To identify the best solution for your unique use case, always test your code and try out multiple approaches.
Conclusion
Congratulations! You’ve mastered Python’s horizontal printing technique. This crucial tactic will enable you to format your output and improve its readability.
We’ve covered a lot of territory in this article, from the fundamentals, like using the print() function’s “end” parameter, to the more complex ones, like loops and formatting options. You may easily print output horizontally in Python using these methods.
There could be some difficulties along the road, though, just like with any skill. Don’t worry if you encounter typical issues like additional spaces or wrong item spacing. Also, we’ve offered troubleshooting advice to assist you in resolving these problems.
Practice is the key to becoming an expert at horizontal printing in Python. Don’t rush things; take your time, try out various methods, and don’t be scared to mess up. You’ll quickly master the procedure by segmenting it into smaller steps and concentrating on one approach at a time.
We sincerely hope that this tutorial has been useful to you as you work to understand how to print horizontally in Python. Happy coding and keep up the practice!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.