Looking for a solution to print a string multiple times in Python?
Then you are in the right place!
We have taken a closer look at three possibilities, examined their advantages and disadvantages, and identified possible problem areas. Let’s go!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Python Quick Answer: How to Print a String Multiple Times
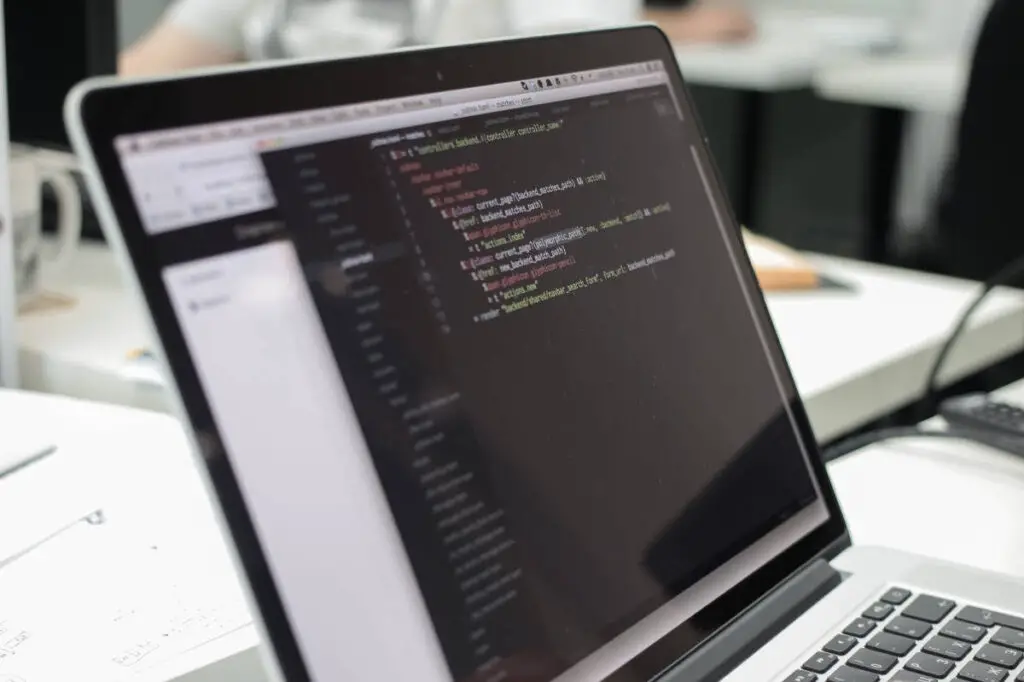
First, let’s answer the basic question of how to print a string several times in Python.
Python has two ways to repeat a string:
- Looping and printing the string.
- Multiplying the string with “*” and outputting the result.
Both techniques are simple!
Let’s examine each method’s pros and cons.
3 Common Ways: Printing Several Strings in Python
Looping
Python loops are used to repeat string printing. This method loops and prints the string.
Code sample:
string = "Hello, world!" n = 3 for i in range(n): print(string)
In this example, we define string as “Hello, world!” and n as 3.
This code yields:
Hello, world! Hello, world! Hello, world!
Python loops can print a string several times for small to medium n values. This method is sluggish and inefficient for higher n. It also requires more code than other approaches we’ll examine in later chapters.
Using “*”
The “*” operator is used to multiply the string and print the result.
Code sample:
string = "Hello, world!" n = 3 print(string * n)
In this example, we define string as “Hello, world!” and n as 3. Then, we multiply string by n and print the result.
This code produces the same output as before:
Hello, world! Hello, world! Hello, world!
Python’s “*” operator prints a string numerous times for any n. It’s less readable than a loop, especially for larger n. If you need to alter the output string or add content, this method may be less versatile.
Join Method
“Join” is an advanced Python method for printing a string numerous times. This method creates a list of the desired number of strings, joins them using “join,” and prints the result.
Code sample:
string = "Hello, world!" n = 3 output = "".join([string for _ in range(n)]) print(output)
In this example, we define string as “Hello, world!” and n as 3. Using a list comprehension, we generate a list of n string copies and link them using the join method with an empty separator. Then we print output.
This code produces the same results as before:
Hello, world! Hello, world! Hello, world!
Python’s “join” technique lets you customize the output format and add content when printing a string many times. It involves more code than prior methods and may be less intuitive for beginners.
Common Challenges for Printing a String Multiple Times in Python
It’s critical to comprehend the issue and the various circumstances in which this work could be needed to efficiently print a string several times in Python.
Creating a string of repeated characters, such a line of dashes or asterisks, is one of the most popular uses for printing a string more than once.
When formatting a table or a report, this activity may also be necessary because it may take many copies of a string to get the correct arrangement.
Another frequent use case is to repeatedly repeat a message or prompt in a program or script.
While it would seem like a straightforward process to repeatedly print a message, there are various difficulties that programmers might run into.
Performance is one such issue, since numerous approaches could perform better than others, depending on the length and frequency of printing of the string. It’s crucial to pick a strategy that can handle the necessary workload without making the software slower.
Another difficulty is formatting.
If the output needs to be formatted in a specific way, it can be difficult to guarantee that the string is printed precisely the required number of times with the necessary spacing and alignment.
Furthermore, it’s crucial to check and sanitize user input if the number of times the string needs to be printed is dictated by user input to prevent mistakes or security problems.
Conclusion
In Python, printing a string repeatedly can be done in several methods, such as with a loop, the “*” operator, or the “join” method. Programmers should select the appropriate method based on their demands because each method has pros and cons of its own.
It’s crucial to comprehend the typical situations and difficulties that arise when printing a string many times, such as performance issues, formatting issues, and user input. Programmers can strengthen their skills in addressing a variety of programming issues by understanding the strategies described in this blog post.
We hope that this blog article has been useful in providing an answer to the topic of how to print a string several times in Python, as well as insightful information about the various methods and difficulties.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.