You might have run into the error message “TypeError: cannot concatenate ‘str’ and ‘int’ objects” if you’re new to Python programming. This error happens when you attempt to combine a string with an integer using Python’s forbidden + operator.
We’ll discuss the significance of this error message, the causes behind it, and how to resolve it in this blog post.
Each solution will come with detailed instructions and code samples. You’ll understand how to prevent this mistake and create Python code that correctly concatenates strings and integers by the end of this essay.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Recognizing the Mistake
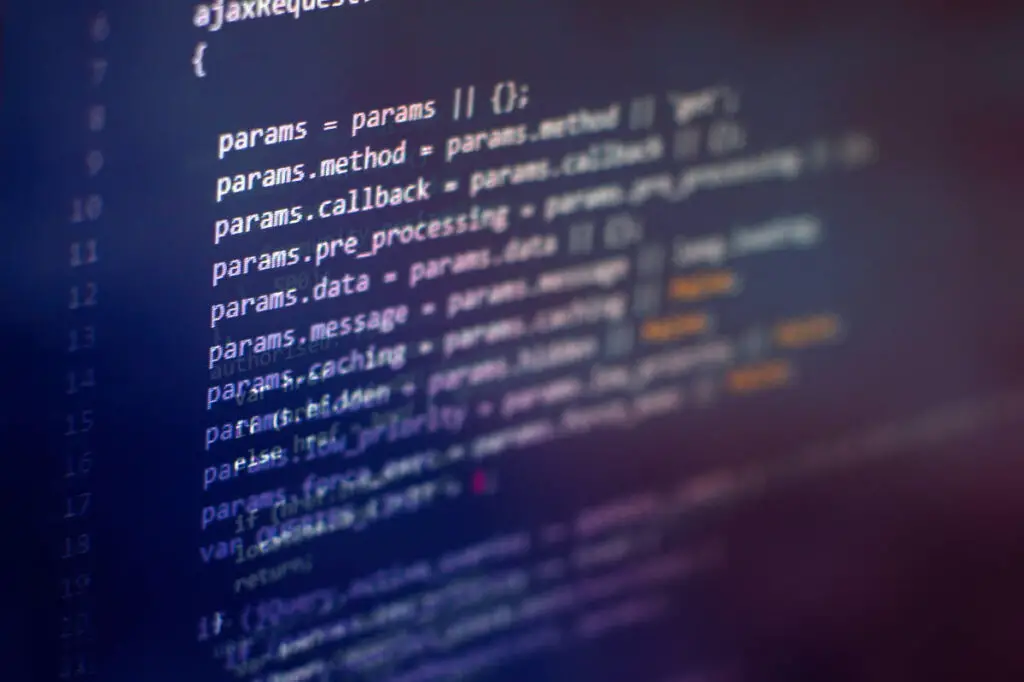
Let’s first examine the causes and implications of this error before moving on to the solutions.
Python returns a TypeError when you attempt to concatenate a string and an integer using the + operator because it is unable to combine two different data types. Strings and integers cannot be directly concatenated in Python since they are different data types.
You must first turn the number into a string before joining it to the string in order to correct this problem. There are various approaches to doing this, which we’ll discuss in the section below.
Before: Using Python, the following code would result in the error “TypeError: cannot concatenate’str’ and ‘int’ objects”:
age = 30 print("I am " + age + " years old.")
In this code, the + operator is being used to concatenate the string “I am” with an integer age. Python raises a TypeError because the age variable is an integer and not a string.
When joining the integer to the string, the integer must be converted to a string to resolve this issue. In the following part, we’ll go over numerous approaches to doing this.
3 possible solutions: “TypeError: cannot concatenate’str’ and ‘int’ objects”
Solution 1: Converting an integer to a string using the str() function
The str() function can be used to convert an integer to a string in order to resolve the “cannot concatenate’str’ and ‘int’ objects” problem. When an object is passed as an argument, the str() function returns a string representation of that object.
Here’s how to turn an integer into a string using the str() function:
age = 30 print("I am " + str(age) + " years old.")
In this code, the age variable is being concatenated with the string “I am” after being converted from an integer to a string using the str() method.
We can get the desired result by avoiding the TypeError and using the str() function instead: I am thirty years old.
Solution 2: Using f-Strings to Concatenate Strings and Integers
Using f-strings is another method for resolving the “cannot concatenate’str’ and ‘int’ objects” problem.
In Python, F-strings are a more recent method of formatting strings that make it simple to embed variables.
Here’s how to combine a string with an integer using f-strings:
age = 30 print(f"I am {age} years old.")
In this code, the string “I am age years old” contains an f-string that contains the age variable’s value. The variable value should be placed into the string at the location indicated by the curly brackets.
We may prevent the TypeError and generate the desired result by using f-strings: I am thirty years old.
Using the format() method is option three
Using the format() function is a third approach to resolving the “cannot concatenate’str’ and ‘int’ objects” problem.
By setting placeholders in the string and then filling them with variables via the format() method, you can concatenate strings and variables.
Here’s how to combine a string with an integer using the format() method:
age = 30 print("I am {} years old.".format(age))
In this code, the string “I am years old.” has the age variable’s value inserted using the format() method. The variable value should be placed into the space between the curly brackets.
We may get the desired output and avoid the TypeError by employing the format() method: I am thirty years old.
Comparison of Fixes for the Issue
After discussing three possible ways to resolve the “cannot concatenate’str’ and ‘int’ objects” problem, let’s contrast the benefits and drawbacks of each approach.
- Although it is simple to turn an integer into a string by using the str() method, adding str() to every integer in your code might be tiresome.
- Although it’s only compatible with Python versions 3.6 and later, using f-strings offers a clear and legible approach to embed variables in a string.
- It is possible to concatenate strings and variables using the format() technique, but it can be more difficult to read and needs more typing than f-strings.
f-strings are the most condensed and readable option, so if you’re running Python 3.6 or later, we generally advise using them. The format() function is a nice option if you’re using an older version of Python or if you prefer a more conventional approach.
Looking for a step-by-step guide to learn coding?
Conclusion
This blog entry explains the meaning of the issue “cannot concatenate’str’ and ‘int’ objects,” why it occurs, and three ways to remedy it using the str() function, f-strings, and the format() method.
You can develop Python code that appropriately concatenates strings and integers by knowing the distinctions between strings and integers in the language and applying one of these fixes.
We advise getting comfortable with these solutions on your code if you are new to Python. You are welcome to post any questions or comments in the section below as well.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.