CSV files are commonly used to store and exchange data between different systems.
In Python, CSV files are handled using various libraries and modules, making it easier for developers to work with CSV data. However, sometimes we need to add a new column to an existing CSV file in Python.
This task may seem straightforward, but there are certain challenges that we may face, especially when working with large CSV files.
In this guide, we’ll explore how to add a column to a CSV file in Python. We’ll start by understanding CSV files and the basics of reading and writing them in Python. We’ll also discuss the problem statement of adding a new column to an existing CSV file and the common use cases for doing so.
Let’s dive in and explore how to add a column to a CSV file in Python!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding CSV files in Python
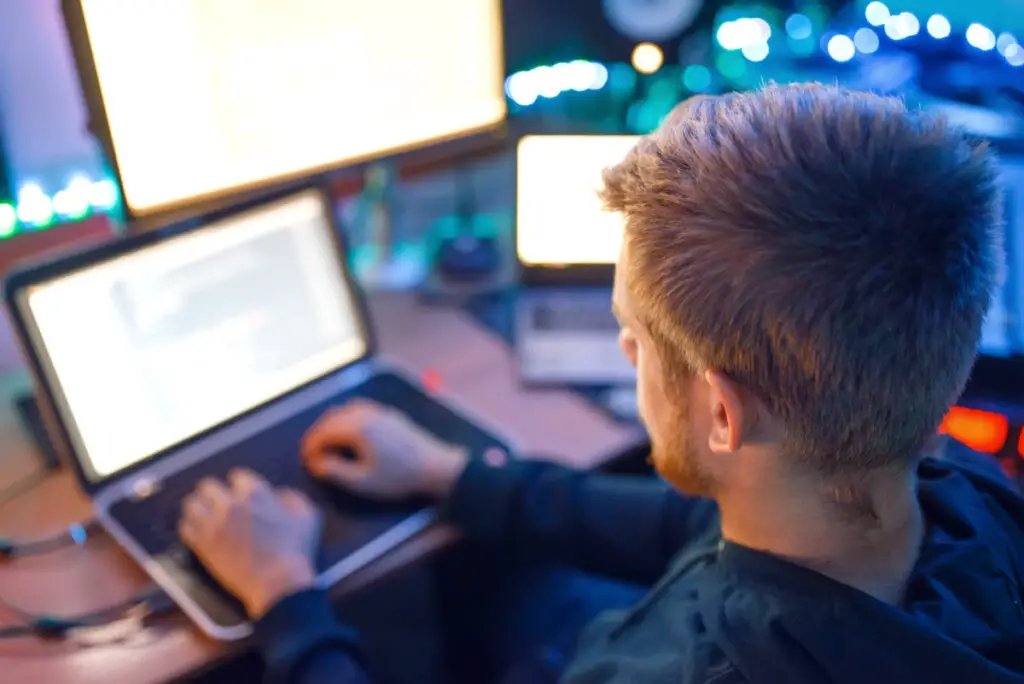
CSV (Comma Separated Values) is a plain text file format that is used to store and exchange data between different systems.
In a CSV file, each line represents a row of data, and each value is separated by a comma. CSV files are widely used in data analysis and data science because of their simplicity and compatibility with most programming languages.
Reading and writing CSV files in Python
Python provides various libraries and modules for working with CSV files, including the built-in csv module and the popular pandas library.
The csv module in Python provides functionality to read and write CSV files in a simple and efficient way.
The pandas library, on the other hand, provides more advanced features for working with CSV data, such as data filtering, manipulation, and visualization.
Working with CSV data in Python
To work with CSV data in Python, we first need to import the required libraries and modules.
We can then use the built-in functions and methods to read and write CSV files, or use the advanced features provided by the pandas library. Once we have loaded the CSV data into our program, we can perform various operations on it, such as filtering, sorting, and transforming the data.
In the next section, we’ll discuss the problem statement of adding a new column to a CSV file in Python.
Problem statement: Adding a column to CSV in Python
Occasionally, we need to add a new column to an existing CSV file in Python.
This can be useful when we want to append new data to an existing CSV file, or when we would like to calculate some new values based on the existing data.
Common use cases of adding a column
There are several common use cases for adding a new column to a CSV file, such as:
- Adding a new column with calculated values based on existing data, such as computing the total price of an order from the unit price and quantity.
- Adding a new column with metadata or additional information, such as the date the data was last updated.
- Adding a new column with external data, such as looking up additional information from another data source based on a key value.
Challenges faced while adding a column to CSV
While adding a new column to a CSV file may seem like a simple task, there are certain challenges that we may encounter, especially when working with large CSV files.
Some challenges are:
- Keeping the order of the existing columns intact while adding the new column.
- Ensuring that the new column is added to all rows of the CSV file.
- Handling errors and exceptions that may occur during the process.
- Maintaining the performance and memory usage of the program, especially when working with large CSV files.
In the next section, we’ll explore various solutions for adding a column to a CSV file in Python, including using the popular pandas library and the csv module.
Solutions for adding a column to CSV in Python
Using pandas
- Installing pandas
Before we can use pandas to add a column to a CSV file, we need to install the library. We can do this using the pip package manager in Python:
Copy codepip install pandas
- Adding a column to CSV using pandas
To add a column to a CSV file using pandas, we first need to load the CSV data into a pandas DataFrame object.
We can then use the df['column_name']
syntax to create a new column in the DataFrame and assign values to it. Finally, we can use the to_csv()
method to write the updated DataFrame back to a CSV file.
Here’s an example code snippet that demonstrates how to add a new column to a CSV file using pandas:
bashCopy codeimport pandas as pd # Load the CSV data into a DataFrame df = pd.read_csv('input.csv') # Create a new column with calculated values df['total_price'] = df['unit_price'] * df['quantity'] # Write the updated DataFrame back to a CSV file df.to_csv('output.csv', index=False)
Using csv module
- Reading and writing CSV using csv module
To work with CSV files using the csv module, we first need to import the module and create a csv.reader
or csv.writer
object. We can then use the methods provided by the reader or writer object to read or write the CSV data.
Here’s an example code snippet that demonstrates how to read and write CSV data using the csv module:
sqlCopy codeimport csv # Read the CSV data into a list of rows with open('input.csv', 'r') as f: reader = csv.reader(f) rows = list(reader) # Add a new column to each row for row in rows: row.append('new value') # Write the updated rows back to a CSV file with open('output.csv', 'w', newline='') as f: writer = csv.writer(f) writer.writerows(rows)
- Adding a column to CSV using csv module
To add a column to a CSV file using the csv module, we can follow a similar approach as above, but with some additional steps.
We first need to create a new list of rows, with the new column added to each row. We can then use the csv.writer
object to write the updated rows back to a CSV file.
Here’s an example code snippet that demonstrates how to add a new column to a CSV file using the csv module:
sqlCopy codeimport csv # Read the CSV data into a list of rows with open('input.csv', 'r') as f: reader = csv.reader(f) rows = list(reader) # Add a new column to each row for row in rows: row.append('new value') # Write the updated rows back to a CSV file with open('output.csv', 'w', newline='') as f: writer = csv.writer(f) writer.writerows(rows)
In the next section, we’ll summarize the key points of the guide and discuss the significance of this topic in programming.
Conclusion
In this guide, we explored how to add a column to a CSV file in Python.
Adding a column to a CSV file in Python is a common task in data analysis and data science. By following the solutions provided in this guide, you can efficiently and easily add a new column to your CSV file, and make the most out of your data.
We hope that you have learned how to add a column to a CSV file in Python, and we have provided you with the necessary knowledge and tools to handle CSV files effectively in your programming projects.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.