Have you ever needed to ignore the first line while working with files in Python?
You might not have wanted to include certain headers or metadata in your analysis because they were present in the first line. You’re in the correct place, then!
This article will demonstrate several ways to skip the first line of a file in Python. It’s a typical activity in data processing and analysis, but if you’re not familiar with Python, it might be challenging.
So don’t worry, we have you covered. Let’s go!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
How to Skip the First Line of a File in Python: Practical Methods and Solutions
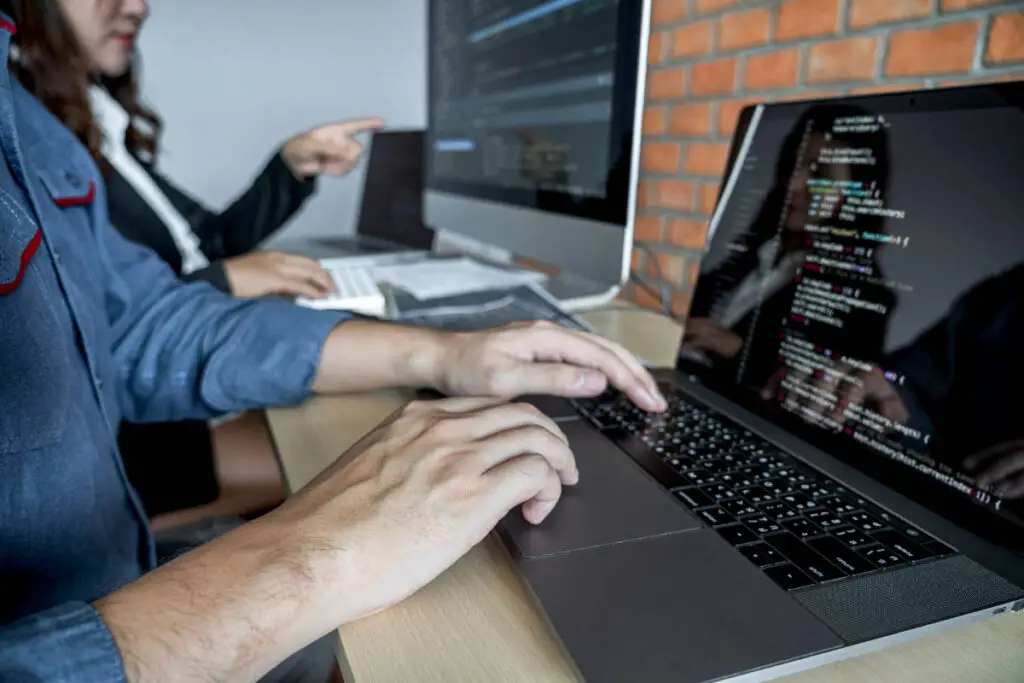
There are numerous approaches available when it comes to skipping the first line of a file in Python.
In this chapter, we’ll go over some of the most popular strategies, their advantages and disadvantages, as well as some sample code.
Using the next() Function
The next() function is a straightforward way to skip the first line of a file.
An illustration of some code is shown below:
with open('myfile.txt') as f: next(f) # skip the first line for line in f: # process the remaining lines ...
The simplicity and readability of this approach are its key advantages. However, it makes the assumption that the file is in a readable format and that the first line only has headers or metadata. The file must also be read in order.
Using the readlines() Method
Using the readlines() method to read every line of a file into a list and then slicing the list to remove the first line is another technique to skip the first line.
Example:
with open('myfile.txt') as f: lines = f.readlines() for line in lines[1:]: # process the remaining lines ...
By enabling you to skip any number of lines, not just the first one, this function is more adaptable than next(). Because it reads the full file into memory at once, it might not be appropriate for huge files.
Using the CSV Module
The CSV module lets you skip the first line when working with CSV files.
See here:
import csv with open('myfile.csv') as f: reader = csv.reader(f) next(reader) # skip the first line for row in reader: # process the remaining rows ...
This approach, which was created especially for CSV files, can also handle more complicated formats such files with multiple delimiters or quotes.
For straightforward files, it might not be as effective as the other approaches.
Using the islice() Function
The initial line of a file can also be skipped using the itertools module’s islice() method.
As an illustration, consider the following:
from itertools import islice with open('myfile.txt') as f: for line in islice(f, 1, None): # process the remaining lines ...
This approach is comparable to using readlines(), but it reads the file line by line, requiring less RAM. Unfortunately, it necessitates the import of a new module and could be harder for beginners to understand.
Different File Formats That May Require Skipping the First Line
In this chapter, we’ll look more closely at several file formats that may call for various strategies for skipping the first line.
Do you have any experience with CSV or TSV files?
These file formats have a particular structure that we need to consider and are frequently employed in data analysis. For instance, in CSV files, the headers, which explain the data in each column, are often found on the first line.
Similar to CSV files, TSV files also feature headers in the first line and utilize tabs rather than commas to divide the values.
Using Python’s csv module, we may skip the first line of a CSV or TSV file. It offers a reader object that, by default, skips the first line.
Here’s an illustration:
import csv with open('myfile.csv') as f: reader = csv.reader(f) for row in reader: # process the remaining rows ...
Working with a TSV file only requires that the delimiter be specified as a tab:
import csv with open('myfile.tsv') as f: reader = csv.reader(f, delimiter='\t') for row in reader: # process the remaining rows ...
Some file formats, however, can call for a different strategy. In some files, the headers might not appear on the first line, or they might be formatted differently than in CSV or TSV files.
If so, you might have to read the file line by line and manually exclude the first line by employing one of the strategies we discussed in the chapter before.
If there is a specialist library or module for interacting with the particular file format, you may also use that as an alternative.
To guarantee that your code performs as intended, it’s crucial to use the right approach for the file type you’re working with. Don’t forget to test your code extensively to make sure it can handle the file format.
It’s time to put your knowledge of how to skip the first line of various file formats into action.
Check to see if you can successfully skip the first line using the right technique by experimenting with various file types. You can handle any file type with a little practice, though!
Possible Issues and Solutions
Although skipping a file’s initial line in Python is a simple procedure, there are some potential problems and dangers that you may run into. This chapter will cover some typical issues you could experience, as well as troubleshooting tips and potential remedies.
Issue: File Not Found Error
You can frequently experience a “File not found” error when attempting to open a file. A misspelled file name, a lack of a file extension, or the file being in a different directory could all be to blame for this.
Solution:
Ensure that the file path and name are accurate by checking them twice. Provide the whole path to the file if it is located in a separate directory. The OS module can also be used to create file paths that are independent of the platform.
Difficulties with encoding
You can run into encoding issues when reading or writing files if you’re working with non-ASCII characters. As a result, the file may process incorrectly or with distorted text.
When opening the file, choose the appropriate encoding. For instance, to open a file encoded in UTF-8, use the syntax open(“myfile.txt”, encoding=”utf-8″). A file’s encoding can also be automatically determined using the chardet module.
Error with File Permissions
A “Permission denied” error could appear if you don’t have the correct permissions to read or write a file.
Ensure that you have read or write access to the file by checking the file permissions. An administrator or superuser might also need to run your Python script.
Issue: Wrong Header Format
Your code could not function as expected if the headers in your file are formatted differently than you anticipated. The headers might be in a different case or contain more spaces, for instance.
Solution:
Verify the header formats in your file and make the necessary code modifications. Before processing the file, you can also normalize the headers using string manipulation techniques like lower() and strip().
Conclusion
We’ve gone over many approaches and solutions for skipping the first line of a file, such as using the next() function, readlines() method, csv and itertools modules. We’ve also highlighted probable concerns and remedies to help troubleshoot frequent situations.
It’s now time to put your knowledge to use! Try with various strategies and approaches to see what works best for your particular use case. Ensure to thoroughly test your code and address edge circumstances to verify that it works as planned.
Take a look at the official Python documentation for more resources and information, or join Python community forums and discussion groups to network with other developers.
Thank you for taking the time to read this, and happy coding!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.