You’ll probably need to extract the initial letter of a string at some point as a Python programmer. Yet, for individuals who are new to string manipulation in Python, this seemingly easy activity might be complex.
String manipulation is an important notion in Python programming since it allows you to parse text input and execute a variety of actions on it. It can, however, be a source of confusion and annoyance for newcomers.
In this blog post, we’ll look at various Python solutions to the problem of extracting the initial letter of a string. As a beginner or intermediate-level programmer, we appreciate that the tremendous amount of material accessible on this topic may be overwhelming.
As a result, we strive to give beginner-friendly solutions and simple step-by-step instructions.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is a String in Python and why is it Important?
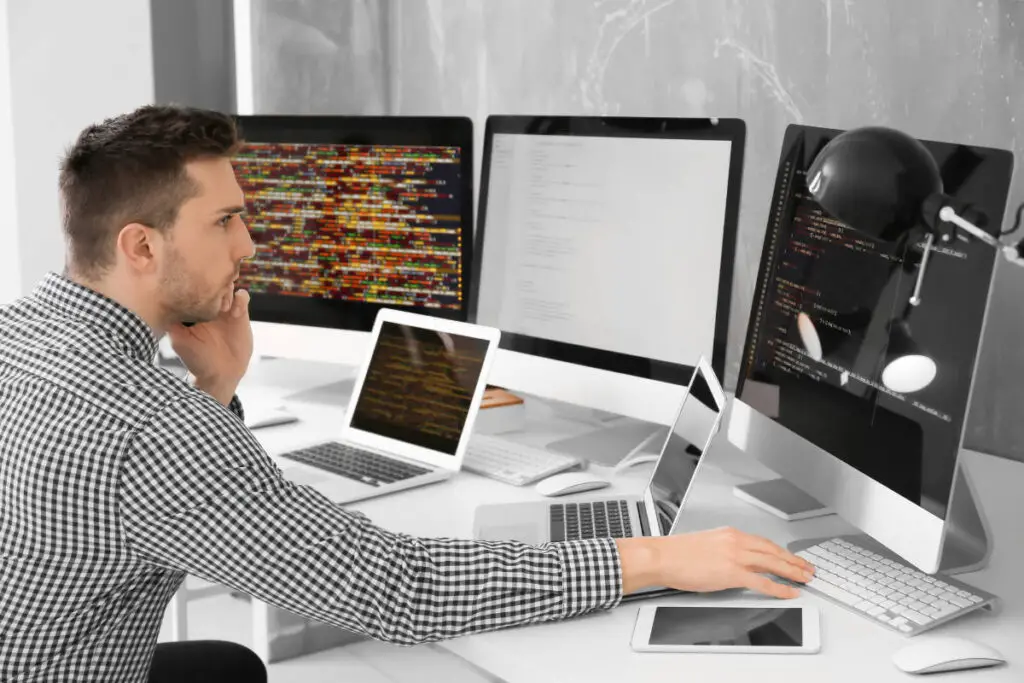
It’s essential to comprehend what a string is and why it’s critical to extract its initial letter to properly extract the first letter of a string in Python.
A string in Python is a group of characters that stands for text data. Python programmers frequently employ strings for a variety of tasks, including processing user inputs, displaying text on the screen, and data manipulation.
It’s frequently required to extract the first letter of a string while working with strings. For instance, you might need to extract the first letter of a user’s name while processing user inputs to address the user properly.
When working with acronyms or abbreviations, extracting the first letter of a string is also beneficial.
It can be difficult for some programmers who are new to Python string manipulation, despite the significance of extracting the initial letter of a string.
Typical difficulties include:
- Misunderstanding Python’s string indexing system
- Failing to consider strings with no characters or only one character
- Difficulty in distinguishing between characters and strings
3 Methods to extract the first letter of a string in Python
There are several methods to extract the first letter of a string in Python. In this section, we’ll explore three commonly used methods and provide practical examples to demonstrate their usage.
Using the index operator
One way to extract the first letter of a string in Python is to use the index operator, which is represented by square brackets. The index operator retrieves a single character from a string at the specified position.
Here’s an example code snippet:
text = "hello" first_letter = text[0] print(first_letter)
In this example, the text
variable contains the string “hello”. We use the index operator to retrieve the first character of the string and store it in the first_letter
variable. The print
statement outputs the value of first_letter
, which is “h”.
Using the string slicing operator
Another method to extract the first letter of a string in Python is to use the string slicing operator. The slicing operator retrieves a range of characters from a string at the specified position.
Here’s an example code snippet:
text = "hello" first_letter = text[0:1] print(first_letter)
In this example, we use the string slicing operator to retrieve the first character of the string and store it in the first_letter
variable. The print
statement outputs the value of first_letter
, which is “h”.
Using the str()
and list()
functions
You can also use the str()
and list()
functions to extract the first letter of a string in Python. The str()
function converts any data type into a string, and the list()
function converts a string into a list of characters.
Here’s an example code snippet:
text = "hello" first_letter = list(str(text))[0] print(first_letter)
In this example, we first convert the string text
into a list of characters using the list()
function. Then, we use the index operator to retrieve the first character of the list, which is the first letter of the string.
The print
statement outputs the value of first_letter
, which is “h”.
Python’s best methods for locating the initial letter of a string
It’s crucial to adhere to best practices when extracting the initial letter of a string in Python to make sure your code is effective, legible, and maintainable.
The best strategies for locating the initial letter of a string in Python will be covered in this section.
Give your variables meaningful names
It’s critical to give variables in Python meaningful names that fully define their function.
By doing this, you can increase the readability of your code and make it simpler for others to understand.
Deal with mistakes and edge cases
To prevent unexpected outcomes when working with strings, it’s crucial to handle mistakes and edge cases.
For instance, the initial letter cannot be retrieved if the string is empty, so you should manage this situation by displaying the proper error messages.
Describe your code in the comments
Making use of comments in your code is a wonderful method to clarify your reasoning and make it simpler to grasp.
The purpose of extracting the first letter from a string should be explained in the comments, along with any further information that may be required to comprehend your code.
Think about performance
Your code’s performance may suffer if you work with lengthy strings. As a result, it’s crucial to think about how your code may affect performance and optimize it as needed.
Looking for a step-by-step guide to learn coding?
Conclusion
In this article, we looked at the significance of extracting the initial letter of a string in Python, as well as the difficulties that programmers could run into when trying to do so.
We’ve covered three typical techniques for obtaining the initial letter of a string and given real-world applications to show how to use them.
Furthermore, we’ve offered some best practices for handling mistakes and edge circumstances, handling performance issues, and utilizing appropriate variable names when extracting the first letter of a string in Python.
You may efficiently and successfully extract the first letter of a string in Python by using the tips and tactics in this article. You can then use these ideas to complete other string manipulation jobs in your projects.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.