Are you sick of employing the same old technique to alphabetize lists in Python?
This blog post will examine workable alternatives to the sort() function for sorting lists alphabetically in Python. We’ll delve deeply into some simple-to-implement strategies that will help you develop your coding abilities.
Let’s dive in.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
How do I sort a list and numbers without the sort method in Python?
Without using the default sort() function, you can sort a list in Python using other techniques like the sorted() function, lambda functions, and custom sorting algorithms. These techniques are simple to use and allow for customizing sorting criteria.
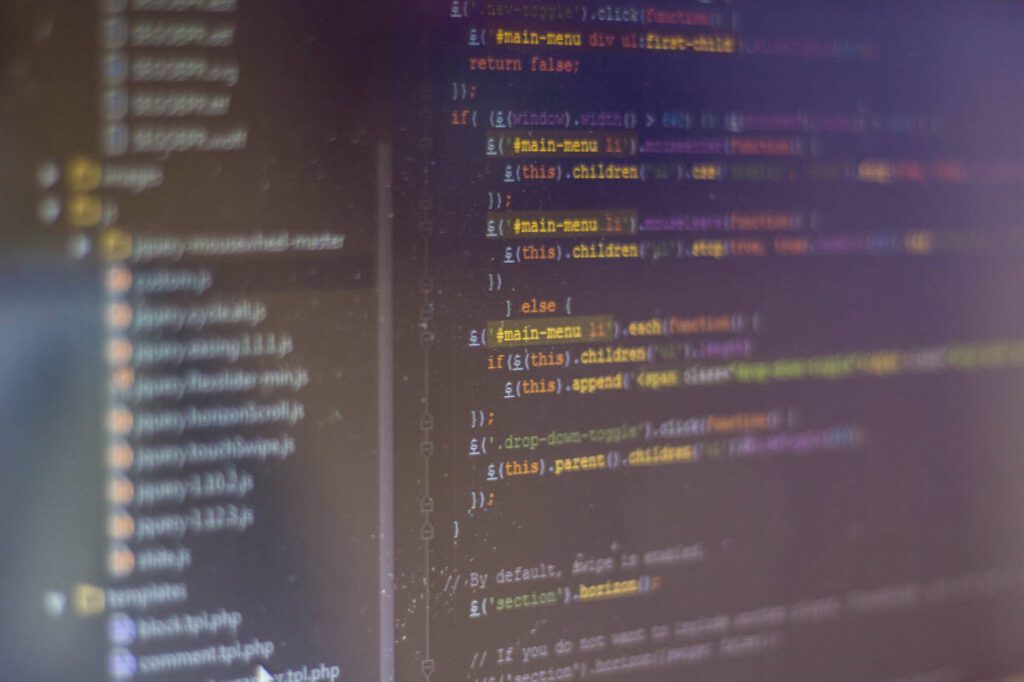
Furthermore, hypothetical challenges like sorting lists containing both integers and texts or sorting in reverse order can be overcome with easy solutions. You can expand your coding knowledge and develop as a better Python programmer by investigating these alternate approaches.
3 Ways to sort a list alphabetically in Python without sort function
#1: Using the sorted() function
One way to sort a list alphabetically in Python without using the built-in sort() function is by using the sorted() function.
An iterable object, such as a list, is passed to the sorted() function, which creates a new sorted list based on the elements of the original list.
By using this technique, you can maintain the original list’s format while obtaining a sorted version of it. If you want a quick and simple way to sort the original list and don’t need to change it, this is a suitable option.
# Original unsorted list unsorted_list = ['orange', 'apple', 'banana', 'pear'] # Use sorted() function to create a new sorted list sorted_list = sorted(unsorted_list) # Print the sorted list print(sorted_list) # Output: ['apple', 'banana', 'orange', 'pear']
#2: Using lambda function
Using lambda functions instead of the sort() method is another way to sort a list in Python.
Lambda functions are small, anonymous functions that can be defined in one line.
To alter the sorting criteria, the sorted() function accepts lambda functions as a key parameter.
# Original unsorted list unsorted_list = ['orange', 'apple', 'banana', 'pear'] # Use sorted() function with lambda function as key parameter to create a new sorted list sorted_list = sorted(unsorted_list, key=lambda x: x[1]) # Print the sorted list print(sorted_list) # Output: ['apple', 'pear', 'banana', 'orange']
This technique gives more customization possibilities compared to the sorted() function, allowing you to sort the list based on a specific element or property of the list items.
#3: Using bubble sort
A list can be sorted using the straightforward sorting algorithm known as bubble sort in either ascending or descending order. If nearby items are in the wrong sequence, it repeatedly switches them.
Despite not being the most effective sorting algorithm, bubble sort is simple to use and comprehend. If you need to sort a short list and want to learn the fundamentals of sorting algorithms, try this method.
# Original unsorted list unsorted_list = [5, 2, 8, 3, 1] # Bubble sort implementation for i in range(len(unsorted_list)-1): for j in range(len(unsorted_list)-i-1): if unsorted_list[j] > unsorted_list[j+1]: temp = unsorted_list[j] unsorted_list[j] = unsorted_list[j+1] unsorted_list[j+1] = temp # Print the sorted list print(unsorted_list) # Output: [1, 2, 3, 5, 8]
By investigating these practical options, you might identify the strategy that works best for your individual use case. These techniques can be used to arrange a list in alphabetical order, or you can alter the sorting criteria to arrange it in a particular order.
Possible problems and solutions
There are possible problems that you might run into when sorting a list alphabetically in Python without using the built-in sort() function. We’ll look at a few of these issues in this chapter and offer remedies for each one.
Sorting lists that include both strings and integers
Sorting a list that includes both strings and numbers is a typical issue.
Due to Python’s prohibition on comparisons across several data types, the sorted() function might not behave as expected in this situation.
To solve this issue, you can convert all elements in the list to the same data type before sorting. This ensures that all items may be compared effectively.
Sorting in reverse order
Another challenge you may find is sorting a list in reverse order. By default, the sorted() function sorts a list in ascending order. But, in rare circumstances, you may need to sort a list in descending order.
You can accomplish this by either using the lambda function to alter the sorting order or by passing the reverse=True parameter to the sorted() function.
Handling both capital and small-cap characters
Furthermore, managing uppercase and lowercase characters might also be a difficulty when sorting a list of strings.
Python distinguishes between uppercase and lowercase characters when sorting alphabetically. Before sorting the list, you can resolve this issue by changing the case of all the elements. This guarantees that all factors are evaluated similarly.
By being aware of these potential problems and solutions, you can avoid common mistakes and sort your list correctly using alternative methods.
Conclusion
In this blog post, we discussed how to sort a list alphabetically in Python without utilizing the built-in sort() function.
The use of the sorted() function, lambda functions, and bubble sort algorithm were some of the practical solutions that were covered in the discussion. We also looked at a few potential difficulties that can occur when employing alternate approaches and offered fixes for each one.
You can arrange your list in alphabetical order or according to particular criteria by employing the strategies and fixes covered in this article. Knowing the potential issues that might occur while utilizing alternate approaches is crucial, as is being able to resolve them.
In conclusion, anyone beginning to write in Python would benefit from learning how to sort a list alphabetically in Python without using the sort() function. It allows you to have more control over the sorting process and adjust the sorting criteria.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.