Writing interactive Python programs, or programs that pause to receive input from the user and then perform some processing based on the user’s input, will become necessary as you progress with Python.
In this article, find out how to take input until enter is pressed in Python and find the answers to some of the most asked questions online about similar topics.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Steps On How To Take Input Until Enter Is Pressed In Python
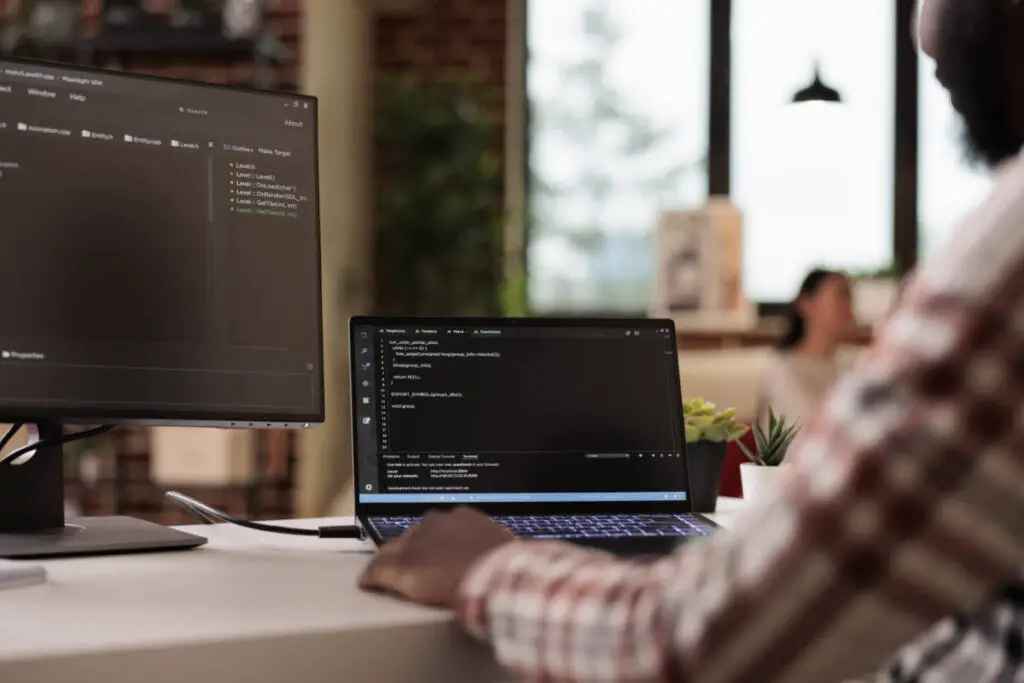
According to bobbyhadz blog, to accept input until the Enter key is pressed, you have to do the following steps.
- Declare a variable that keeps an empty list.
- Use a “while” loop to iterate as many times as necessary.
- Add each value entered by the user to the list.
- The “while” loop should be broken when the user clicks Enter.
# ✅ When taking strings as input my_list = [] while True: user_input = input('Enter a string: ') if user_input == '': print('User pressed enter') break my_list.append(user_input) print(my_list) # --------------------------------------------- # ✅ When taking integers as input my_list = [] while True: user_input = input('Enter a number: ') if user_input == '': print('User pressed enter') break try: my_list.append(int(user_input)) except ValueError: print('Invalid number.') continue print(my_list)
While iterating indefinitely, he utilized a while loop to accept user input.
A break statement or raising an exception is the only way to exit a while True loop.
my_list = [] while True: user_input = input('Enter a string: ') if user_input == '': print('User pressed enter') break my_list.append(user_input) print(my_list)
We exit the while loop and record an empty string in the user input variable if the user pushes Enter.
The innermost for or while loop is broken by the break statement.
If not, the input value is added to the list.
The item is added to the end of the list using the list.append() function.
Here is an example that accepts any quantity of integer-type user input values until the user clicks Enter.
my_list = [] while True: user_input = input('Enter a number: ') if user_input == '': print('User pressed enter') break try: my_list.append(int(user_input)) except ValueError: print('Invalid number.') continue print(my_list)
The input function accepts an optional prompt parameter and does not add a terminating newline when writing to standard output.
Following that, the function reads the line from the input, creates a string, and returns the outcome.
The input() method will always return a string even if the user provides a number.
Each string was changed into an integer using the int() class.
The ValueError triggered whenever an incorrect integer is provided to the int() class is handled with the try/except statement.
Frequently Asked Questions
Here we will, as usually, answer the questions about Python and related topics. Keep reading to find out the answers to some of the most asked questions online!
In Python, How Do You Wait For Enter?
To do this, you may utilize the input() method.
The software will wait endlessly for the user input in this scenario.
When a user enters data and pushes the enter key, the software begins to run the subsequent statements.
How Do You Make Python Wait For Input Before Continuing?
By including a sleep() call in Python with time.
The sleep() method in the time module allows you to pause the caller thread’s execution for whatever length you choose.
You should wait before entering a new statement into the REPL if you execute this code in your console.
Does Python Have A Wait Until Function?
The wait() function is frequently used to wait for anything to happen in a process.
It will wait until the function is called with the appropriate conditions or modes supplied in a true or false manner.
The wait() method is defined in Python in two different modules.
How Does Python Postpone An Action?
The sleep() function is used between the two statements between which the delay is desired to add a temporal delay during execution.supplying an integer or float value for the argument in the sleep() function.
Run the application. Take note of the execution time delay.
How Is Delay () Implemented?
You may halt your Arduino program’s execution for a specific time using the delay() method.
The method asks you to provide a full integer that tells it how many milliseconds to wait for it to happen.
In Python, How Do You Wait For 10 Seconds?
You may use a straightforward function like this one: “time”, in a Python application, to make it wait.
In there, the x is the duration in seconds that you want your application to wait. Use it like this: sleep(x).
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.