If you use python, you probably know just how much you can do with it. However, getting to grips with it can be a challenge and a half. In fact, getting accustomed to Python could be seen as an equal challenge as trying to become an Adobe Lightroom pro.
It’s not easy, but don’t worry, if you are trying to figure it out, we have got you covered.
Today we will teach you how you can split a list in Python using Python Programming.
Lists are basically just mutable data types which are able to store items. We will go over the many ways in which you can split a list, from splitting it in half, into sublists and even into chunks.
So, are you ready? Put your thinking caps on, take some notes, and let’s go!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Splitting A List In Python
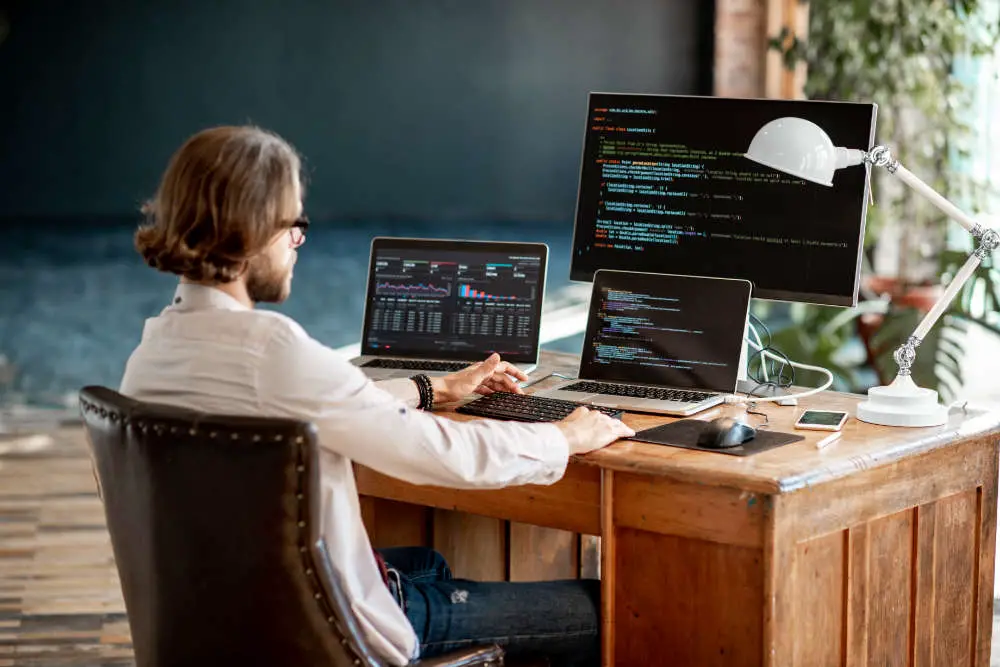
Splitting a list in python is not that tricky overall, there are various ways in which you can do this. You could split it in half, into sublists, or into chunks, it is not too tricky, you just need a little overall know how of the whole system and the code needed, as well as features of the system.
Splitting In Half
Okay so, when you have an input list, and you want to split it into two halves you can do this, depending on if it is symmetrical or asymmetrical you can do this one of two ways.
If you wish to split it when the list is symmetrical it may be something like this;
Input: 1, 7, 5, 3, 2, 8, 9, 4
And the output would end up looking like this; 1, 7, 5, 3 and then 2, 8, 9, 4.
However, if the list is asymmetrical then it might be something such as;
Input: 12, 6, 2, 4, 4, 81, 9, 32, 5, 7, 11.
And then the output would likely end up looking something like this; 13, 6, 2, 4, 4 and then 81, 9, 32, 5, 7, 11.
When you have halves, they could be equal in size, or not, it depends on the list length. Either is possible. You can then use the slicing technique to split the list.
Simply do the following;
- Gather the length of a list using the function len()
- If the length of these is not give then you could also divide the list length in half by using the floor operator to get the middle of the index of the list instead.
- Finally, slice it in half using these [:middle_index] followed by [middle_index:].
Splitting Into Sublists Or Parts
You can also split into sublists, which in some cases is not just relevant but also a way to make data neat.
So, you may have a list that looks like
Input: 5, 3, 2, 1, 3, 24, 5, 41, 35, 4, 6.
And when you split the list, it will look like this; 5, 3, 2, and, 1, 3, 24, and 5, 41, 25, and, 4, 6.
Python has a cool feature known as NumPy, which has the ability to ‘array_split()’, it is able to divide your list into multiple parts. It takes your array as well as the number of splits as an argument, then gives you back a list of subarrays.
It sounds complex, we know, but there is much more to it. So, let’s have a look at how you would expect this to function.
First up, an example
Import numpy as np.
#creating a list
list1 = [1, 2, 4, 7. 8, 3. 5. 6, 9]
Sub_lists = np.array_split(list1, 3)
count=1
For i in sub_lists:
print (“List”, count “: “,list(i))
count+=1
This ends up looking something like
List 1: 1, 2, 4.
List 2: 7, 8, 3.
List 3: 5, 6, 9.
Doing this you use the NumPy library that takes your list and how many splits you want, returning to you the parts of your list.
Splitting Into Chunks
Okay, but what if you want to split your list into a series of chunks? Well you can do this as well using Python.
Here’s an example of what to expect.
If you split it into chunks it will be like so;
Input: 24, 54, 9, 76, 32, 42, 9, 64, 3, 1, 98, 22, 5
This means that the output might look like this;
24, 54, 9, and 76, 32, 42, and 9, 64, 3, and 1, 98, 22, and finally, 5.
So, when you are splitting your list into chunks, be aware of the list given and the length of the chunks, if you are needing to split it, then you can do so by slicing the operator.
So, how do you do this?
Well, here is an example of the code.
Def split_list(input_list, n):
For i in range (0, len(input_list), n):
Yield Inpit_list [i:i + n]
If__ name __==”_main_”:
# create and empty list
List1 = [ ]
# Take number of elements as input from user
Length = int(input(“Enter number of elements : “ ))
list1.append(item)
#take the size of chunks as an input from user
N = int(input(“Enter the size of chunks : “))
X=split_list(list1, n)
print(list(X))
To Conclude
Of course this shows that there are various different ways in which you can split a list in Python. For beginners to python it will take some practice, but it is easy enough to practice and learn.
There are many ways you can split your list and make sure that it looks exactly how you want and need it to look.
If you are new to python, to code and its operations, it is well worth getting in some practice an djust playing around with it for a while to get used to all its quirks, features, and the way that the system works overall.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.