Coding makes up a huge part of our daily lives, even if we don’t think about it. For those people who love coding and have taken it upon themselves to learn everything about it, Python plays a huge role!
If you’re one of those people who want to learn everything there is to learn about coding, or Python in particular, you’re in the right place!
Here, we’re going to be taking a look at what is mutable in Python. To be specific, we will be looking at whether lists are mutable in Python or not (spoiler, they are).
To start off, though, we’ll be learning what “mutable” actually means in terms of python, after that, we will learn how to do it.
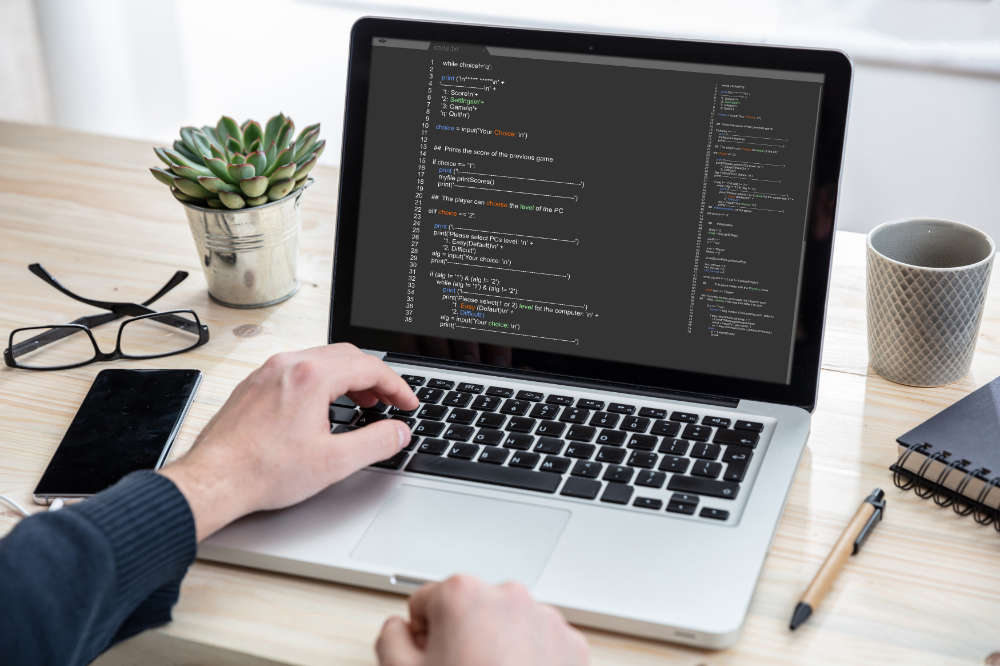
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What Does “Mutable” Mean in Python?
The term “mutable” is simply a fancy way of stating that an object’s internal state has been either mutated or changed. While we may think of “mutable” in terms of volume, like we might mute a TV, the term means something completely different in coding.
An object that is mutable in coding can have its internal state changed (mutated). This is a very important thing to understand when it comes to understanding Python structure as a whole.
The term will also be used to refer to an object that has the ability to be changed, or mutated. The mutation or change does not need to have happened yet for an object to be considered mutable. Mutable objects are typically the various kinds of objects that store a collection of data.
What Does it Mean if Something is “Immutable” in Python?
By contrast, something is “immutable” in Python, it is something that cannot be changed or mutated. No changes are allowed to happen to an immutable object after it has been created. Even over time, there is no possibility of any changes occurring.
You might hear that the “value” of an object is immutable.
Are Lists Mutable in Python?
Now that we know what mutable and immutable means in Python, it should be fairly easy to figure out that lists are mutable objects. In other words, lists are able to change or “mutate” in Python.
Understanding What an Object is in Python
Before we continue, it’s important to understand exactly what we mean by “objects” in Python. Let’s go through it here.
Everything in Python is treated as an object, and all objects will have three attributes. These attributes are the following:
- Value – the value refers to the value that the object is storing. An example of this would be List-[1,2,3]. This list holds the numbers 1, 2, and 3, hence the value.
- Type – the type refers to what kind (or type) of objects that have been created. Some examples of types would be things like integer, string, list, etc.
- Identity – the identity refers to the location or address on a computer’s memory where an object is referred to.
Which Objects Are Mutable and Immutable in Python?
Built-in type objects in Python that are mutable include the following:
- Dictionaries
- Sets
- User-Defined Classes (this depends on the user to define all the characteristics)
- Lists
Build-in type objects that are immutable, or cannot be mutated in Python, include the following:
- Tuples
- Strings
- Numbers (Booleans, Complex, Decimal, Float, Rational, and Integer numbers)
- User-Defined Classes (this depends on the user to define all the characteristics)
- Frozen Sets
How to Mutate Lists in Python – An Example
Below, we will take a look at an example of mutation in Python.
To check out the original site, just click here. This example should hopefully help you understand a little more about mutability in action.
foo = [‘hi’]
print(foo)
# Output: [‘hi’]
bar = foo
bar += [‘bye’]
print(foo)
# Output: [‘hi’, ‘bye’]
As you can see, the output is something that we did not expect to see. Instead, we expected to see something like this:
foo = [‘hi’]
print(foo)
# Output: [‘hi’]
bar = foo
bar += [‘bye’]
print(foo)
# Expected Output: [‘hi’]
# Output: [‘hi’, ‘bye’]
print(bar)
# Output: [‘hi’, ‘bye’]
When we look at mutability in lists, we have the following coding in Python as an example:
def add_to(num, target=[]):
target.append(num)
return target
add_to(1)
# Output: [1]
add_to(2)
# Output: [1, 2]
add_to(3)
# Output: [1, 2, 3]
The expected result may have been something more along the lines of this:
def add_to(num, target=[]):
target.append(num)
return target
add_to(1)
# Output: [1]
add_to(2)
# Output: [2]
add_to(3)
# Output: [3]
Because of the mutability, it can be extremely confusing and difficult to understand why the result is different to our expectation.
In Python, default programs are only evaluated once, when the function is initially defined. It does not evaluate the function every time that the function is called.
Because of this, you shouldn’t ever define default programs if they are mutable unless you have a clear idea of exactly what you are doing.
Instead, something like this should be done:
def add_to(element, target=None):
if target is None:
target = []
target.append(element)
return target
When you do this and call the function from now on without the target argument, you will create a new list. To understand this better, check out the following code below:
add_to(42)
# Output: [42]
add_to(42)
# Output: [42]
add_to(42)
# Output: [42]
Final Thoughts
Python can be very confusing, especially if you are brand new to it. There are a number of concepts that require time and patience to understand fully.
When it comes to understanding the mutability of objects, the concept can be equally confusing.
However, once you have a basic understanding of mutability and immutability, you will be able to better understand Python as a whole.
To sum things up, lists, along with sets, dictionaries, and some user-defined classes, are mutable objects. That means that they can be altered or “mutated”.
If you are looking for objects that are immutable in Python, those would be frozen sets, strings, numbers, tuples, and some user-defined classes.
Hopefully this post has been helpful, and you know a little more about lists in Python and mutability. Good luck!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.