Functions are crucial to interacting with and controlling data in the Python coding language. Functions operate as blocks of code that are put into action when you, the user, ask them to.
Functions can be packed full of any data you want, and when they eventually run, they will result in new data being generated as a result. Thus, you can use functions to help you to analyze data.
However, as useful as functions already are within Python, they can actually be even more useful, and can also be made to return multiple values!
But how do you get a function to do this? What does it involve, and can you do it yourself? Read on below to find out now!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
How Do You Return Multiple Values In Python?
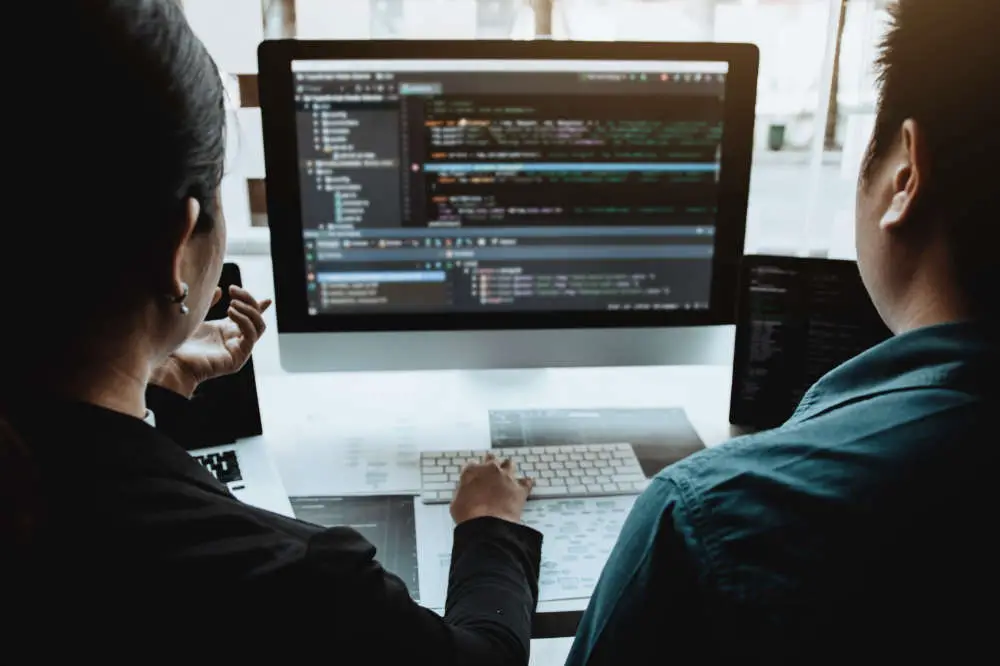
Luckily, unlike with other coding languages, it is very easy to return multiple values within the Python language.
In order to get multiple values from one function, you simply need to use a return command, and, directly after it, separated by commas, list out all of the values within the function.
Once you press “Enter” after doing this, you should find that you are met with multiple values.
It is important to mention that, within Python, values that are separated by commas are referred to as “Tuples”. Tuples refer to variables that are used to store more than one value at once.
They are one of many different types of storage methods within Python. This is why you need to make use of them if you are planning on generating multiple values.
Each time a value is returned by your function, you will find that they are all stored as tuples.
Are There Any Other Methods To Return Multiple Values In Python?
Yes. If you find that the tuples method does not suit you, then there are plenty of other methods that you can try out. Let’s take a look at some of them now!
Use A List
Python allows users to easily create lists using their data which can help to make data much easier to organize in a sensible manner. Lists, within the context of Python, refer to stratified structures that organize elements into a logical order.
They can be very useful, as they can help to organize your data for you so that it stays today and readable.
This method utilizes the effectiveness of lists in Python. Since lists, very similar to tuples, are collections of organized items, you can use them to return multiple values. In order to do it, use this code:
“Def multiple():
Operation = “Sum”
Total = 5+10
Return [operation, total];
values = multiple()
print(values)”
This will result in the function yielding multiple values in one go. Once this has happened, you will need to quite painstakingly search through all of the results in order to find exactly what you are looking for.
Use A Dictionary
Dictionaries are another form of value storage that can be used in Python, and thus they can be utilized in order to return multiple values from one function.
In order to make use of a dictionary for such results, you will need to type in the following code:
“def multiple() :
Values = dict() ;
values [‘operation’] = “Sum”
Values[‘total’] = 5+10
Return values;
Values = multiple()
print(values)”
All of the values that are returned in this method are much easier to sift through with this method than using lists, as the values will be logically organized, and made much easier to understand.
Use a Python Data Class
Finally, if you find that none of the other methods have worked out for you, you could also try out this method that makes use of Python’s data classes.
Data classes are used to add methods to a class that has been defined by the user. In order to use this method, simply type the following code into your terminal:
“From dataclasses import dataclass
@dataclass
Class multiplevalues():
Operation: str
Num1: int = 0
Num2: int = 0
def values(self) -> float:
Return self.num1 + self.num2
All_values = multiplevalues( “Addition”, 5, 10)
V = all_values.values()
print(v)
print(all_values)”
Though this code may appear lengthy and cumbersome, once it has been typed in, it will yield multiple results from a function that are very easy to utilize.
However, it must be noted that this is the method that presents the most difficulty, and as such, it should generally be depended on by those with plenty of existing experience with the Python coding language.
Frequently Asked Questions
What is Python Return?
The “Return” statement used in Python is one that causes a function to result in data being returned to the user after the function has been completed.
This is why returns are so useful to Python users that are using the platform to organize or analyze data!
How Do You Print A Return Value In Python?
Once you have utilized a return function, and you want to see the results of that function represented visually, you are going to need to print the return value.
In order to do this, all you need to do is head to your terminal and enter the “print()” function into it. This will cause the values of your function to be displayed.
Is Python OK For Beginners?
Compared to other coding languages, Python is actually surprisingly beginner-friendly. This is thanks to the fact that the Python coding language has a syntax that is not too far off from the ordinary syntax used in the English language.
Thus much of it makes sense even to the layperson!
To Conclude
Hopefully, now, you feel ready to alter your functions to help them to yield multiple values every time you use them in the Python program.
The methods explored above all work in their own unique ways, and offer their own stable of benefits that can help to optimize your experience when organizing data in Python.
However, some methods are definitely easier than others, such as the dictionary method, which makes it much easier to understand the multiple values once they have been created.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.