Loops, which repeat code, are crucial to programming.
Loops can be complicated to handle, especially when errors or unexpected behaviors arise.
This blog post will cover Python loop restarting. Step-by-step instructions, practical examples, and theoretical explanations will help you understand the subject and enhance your programming skills.
We are confident that this article helps programmers of all levels.
Let’s go!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Preliminary Consideration: What is a Loop?
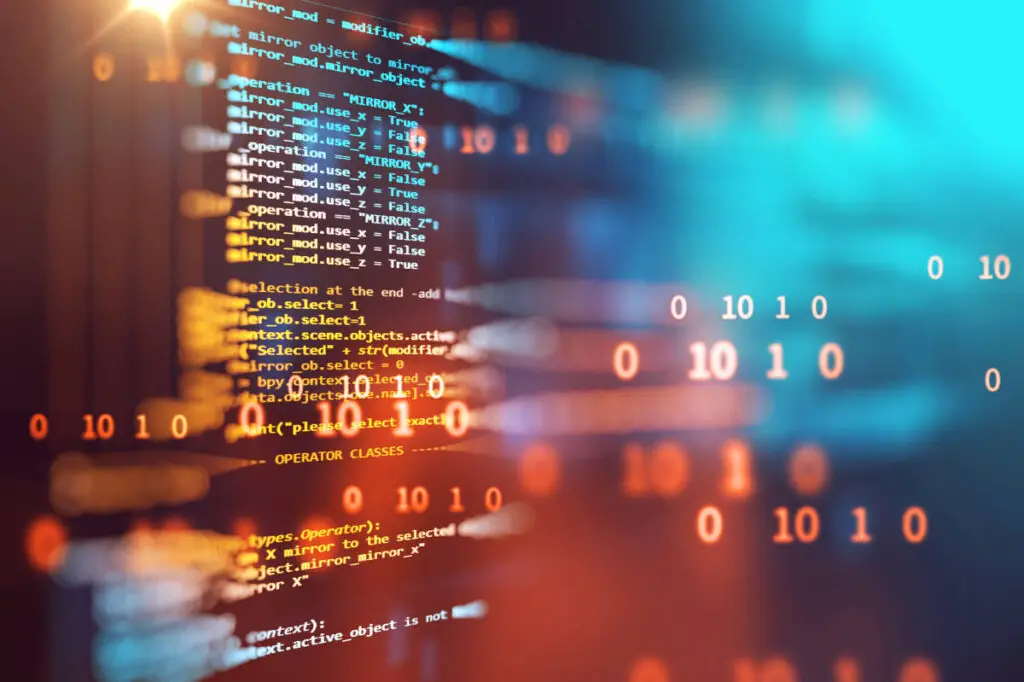
To learn how to restart a loop in Python, we must first define the term. Simply speaking, restarting a loop means restarting it from the beginning.
This is useful when you wish to restart a loop with the same initial conditions numerous times.
Depending on the type of loop, there are numerous ways to restart it in Python. In this post, we’ll go over these methods in depth and show you how to use them in your code using real examples.
Solutions and Examples
The most effective approach to utilize will depend on the kind of loop and the particular issue being addressed.
There are several practical ways to restart a loop in Python. Examine several popular techniques and the circumstances in which they are advised.
#1: Restarting a While Loop
When starting the loop over from the beginning and skipping the current iteration, this statement is advised.
When a condition needs to be tested repeatedly and you need to start over if the condition is not met, it can be helpful.
Here is an illustration:
while True: # do some code here if some_condition: continue # restart the loop
As long as the condition is True, this code will keep executing the loop. Once the condition has been determined to be False, the loop will end and the program will resume running.
#2: Resuming a For Loop
A for loop in Python can be restarted by using the “break” statement. When you wish to stop the loop and start it over, using this statement is advised.
It can be helpful in circumstances when you need to loop through a set number of things repeatedly and start again if a condition is not satisfied.
Example:
for i in range(10): # do some code here if some_condition: break # exit the loop and start again
As soon as the condition is satisfied, this code will continue executing the loop until it is finished, at which point it will stop and restart the loop again.
#3: The Restart of a Loop Using a Function
When you need to restart a loop numerous times, defining a function that houses the loop and invoking that function to restart the loop is a good technique.
When you need to run the loop repeatedly under the same initial circumstances, this way is advised.
Example:
def my_loop(): while True: # do some code here if some_condition: return # exit the loop and return to main code # call the function to start the loop my_loop()
The loop is specified in this example within a method called “my loop()”. When the condition is satisfied, the loop will be broken and the function will return to the main program.
As necessary, the loop can be restarted by repeatedly calling the function.
Tips & Best Practices
There are a few guidelines and best practices to follow while restarting a loop:
- To make the code more clear and understandable, give your variables names that are descriptive.
- To prevent mistakes and unexpected behavior, keep the loop code as straightforward as you can.
- To make sure the code functions as intended and satisfies the criteria, fully test it.
- Select the approach that best satisfies the demands while keeping in mind the unique problem that needs to be solved.
You can successfully restart loops in Python and prevent probable issues or unexpected behavior by keeping these pointers and potential difficulties in mind.
Possible Problems When Restarting a Loop in Python
In Python programming, restarting a loop can be a beneficial method, but if done incorrectly, there may be problems. Here are some frequent problems to be aware of and some advice on how to prevent them.
Infinite Loops
Creating an infinite loop could be an issue when restarting a loop.
When the loop’s condition is never satisfied, this happens and the loop runs endlessly.
Make sure the loop condition can be satisfied at some point to prevent this, or add a break statement to end the loop after a predetermined amount of iterations.
Unexpected Behavior
Unexpected behavior may also arise while restarting a loop.
This can happen if the loop code is very complicated or if the loop is not correctly constructed to handle every scenario that might arise.
Keep the loop code as straightforward as you can and test it extensively before adding it to your application to prevent unexpected behavior.
Comprehensive Code Review
It is crucial to carefully check the code before putting it into practice to prevent any problems while continuing a loop.
By doing so, you’ll be able to spot possible issues early on and make the required adjustments to steer clear of mistakes and unexpected behavior.
Furthermore, if difficulties do develop, utilizing descriptive variable names and comments helps make the code simpler to comprehend and debug.
Use of Loops in Practice
Programming uses loops frequently. Loops display dynamic information, repeat arrays, and do other recurring operations in web development. Loops calculate huge datasets in data analysis.
Loop usage examples:
- Loops display user profiles, postings, and comments on a web page in web development. Loops can display blog entries on a homepage.
- Data analysis loops handle huge datasets and calculate. Looping through each day’s temperature values can generate a month’s average temperature.
- Loops update and handle player input in game development.
Programming loops are useful and adaptable. Programmers can improve code by using loops efficiently.
Conclusion
Python loop restarting is essential. Real-world solutions and examples can help you restart programming loops and solve problems faster.
Web, data, and game development all use loops. Loop proficiency helps programmers create more dynamic and successful applications.
This post should have helped you understand Python loops. If you practice and master new loop approaches, you’ll become an excellent programmer.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.