When you are programming, regardless of what system you use you will often find yourself having to do string comparisons.
It’s a very common scenario, however you will also find that a majority of the algorithms for string comparison will craft the strings through character.
They will do this character by character, individually.
When we use something like C++ this gives us some built-in operation such as != or even == in order to compare the strings as well as the use of functions such as ‘compare’ or strcmp.
So, how can we learn how to compare strings using this?
Today we will find out.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
How Do You Compare Strings In C++?
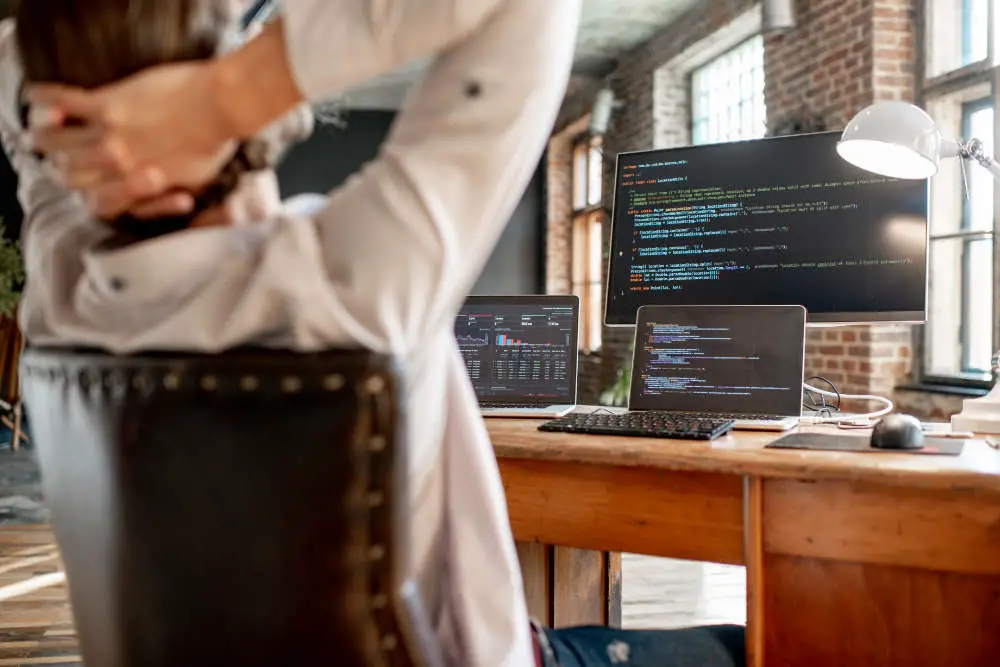
Today we intend on teaching you the various three methods that you can use to properly compare strings in C++.
You may have to consider a situation where perhaps you would need to enter a name and password into a website in order to log in.
In these kinds of cases, which are all too common, there is a backend which needs to be built up with functions in the script that are able to compare and check the login details (otherwise known as input string), with the string that is stored inside the sites’ database.
This is why it is necessary to know how to compare strings in C++, There are luckily three ways in which you could compare strings in C++ and today we will look through all of these.
In C++ you can compare strings by using strcmp(), in-built compare() functions, or via C++ relational operators as well. So, we will look at each of these individually for you to choose which may be best for you.
Using the ‘strcmp()’ Function
When you use C++ String you will find that it actually has some built-in features that will allow you to manage, deal with and manipulate data that is formed in string types.
So, if you wish to compare two strings in C++ then you can use the function enabled by String known as the ‘strcmp()’ function.
This function is basically a function in the C library which is used to compare two strings in a way that is lexicographical.
The syntax you will want to use for this would likely look something like this;
Int strcmp (const char * str1, const char * str2 );
Using this function should return as 0 if both of your strings are the same or equate to being equal. Do note that your input string also needs to be a char array that is a string of C-style.
Also, be aware that using the strcmp() function will compare your strings in a form which is case-sensitive as well.
Using The ‘in-built compare()’ Function
Not only does C++ have the strcmp function, but it also has other functions that are already in its system as well. You can have the C++ in-build function to ‘compare()’ as well. This will also compare your two strings as well.
Using this function to compare() you will be comparing two strings, and it will return values according to the match cases. This means that it will return 0 if both of the strings are identical.
It will return <0 if the character of the first string is smaller in value in comparison to the value of the second string character’s input.
Your results will also be higher than 0 if your second string is more when compared to the first.
You will be wanting the syntax for this which will look something like this;
Int compare (const string& string-name) const;
Note that if both of your strings which you are comparing are lexicographically the same then you will get a return of 0.
Using C++ Related Operators
Finally, you can also use relational operators when you want to compare strings in C++. These are operators such as != and ==. These can be very handy to know and be knowledgeable about when you are looking to compare strings.
In fact, using these two can be very useful in comparing strings easily.
The syntax for doing this would be
String1 == string 2
However, it could also be string1 != string2
Note that == means ‘equals to’ while != means does not equal to.
This is basically the vanilla way of comparing 2 separate strings.
When we use == in the C++, this operator is simply used to compare two different entities to see if they are valued equally, or if not. It is probably one of absolutely the easiest ways that you can actually compare two strings in C++.
It is basically just like simple math.
Let’s look at an example.
Say your code looked something like this;
“
String firstString = “Scaled”;
Sting secondString = Scaler
If (firstString != secondString) {
Cout << String 1 is NOT EQUAL to String 2” << ‘\n’;
} else {
Cout << “String 1 is EQUAL to String 2” << ‘\n’;
} “
In this instance String 1 is NOT EQUAL to String 2. While in some cases the outputs might remain without change, the Scaler and Scaled strings are unequal. And the way of writing the conditions in the code changes.
To Conclude
How can you compare strings in C++? Well, the overall answer is that there are multiple ways you can do this, and the good news is that for the most part they are already there for you to make use of.
There are 3 main ways you can do this, and there is no saying if one is better than the others, just personal preference really. Although we might say that using operators is probably the easiest as it is the most straightforward way to do it.
Note that there are differences between each of these, even if they may seem to be very similar. While relational operators and the compare() function seem similar they are not.
So, pick your favorite way and run with it, it is actually very easy!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.