Division is a simple math operation that you may need to do from time to time. When it comes to coding, there are many occasions that you might need to divide.
Thankfully, almost all programming languages have some form of Arithmetic Operators – this is basically a long-winded way of saying that you can use C++ to add, subtract, multiply or divide.
If you’re trying to work out how to divide in C++, then you’ve come to the right place! In this article, we’re going to be taking you through everything to do with C++ Arithmetic Operators.
We’ll give you a few examples of how you can do this within your coding sessions.
Let’s get started!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Arithmetic Operators
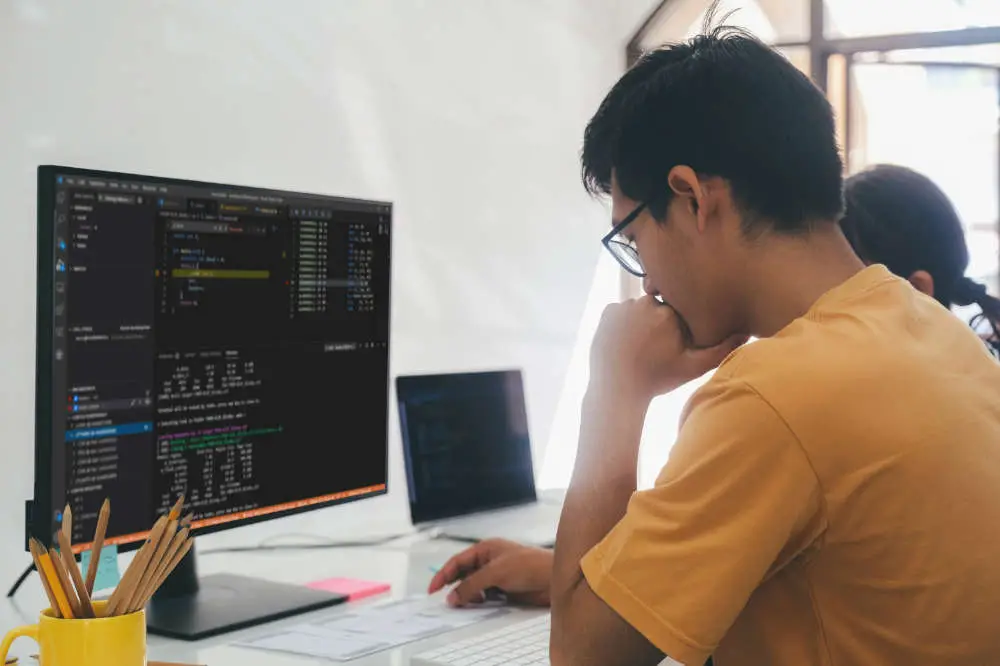
If you’re new to C++, then you might want to experiment and see what kind of cool things you can do. Arithmetic Operators are a great way for you to learn what C++ is capable of, and a great tool on your belt for you to use in the future.
Operators: within C++, operators are common symbols that can perform certain operations involving values and variables.
To put this very simply the ‘-’ symbol is used for basic subtraction and – you guessed it – the ‘+’ symbol can in turn be used for addition.
There are different forms of operators including Assignment Operators, Arithmetic Operators (which we are going to be looking at for the purpose of this article), Relational Operators, C++ Logical Operators, and finally Bitwise Operators.
If you’re looking to seriously learn C++ it’s a good idea to get familiar with all of these, but the best place to start is probably Arithmetic Operators.
Defining Operators And Operation
So within this classification, we can break down each symbol into its corresponding operation.
- The Operator for + is Addition.
- The Operator for – is Subtraction.
- The Operator for * is Multiplication.
- The Operator For / is Division.
There is also the % operator, but we will save that for another time to keep things simple. An Arithmetic Operator works by using two values (referred to as operands), that will be used to calculate an answer. This works the same way that any math problem might be solved, for example:
1 + 2 = 3.
Simple enough, right? Well to get this operation to work within a program, there is some additional code that you’ll need to include. First, you’ll need to define the integers that you’ll want to include. This will look like this:
int main() {
int a, b;
a = 1;
b = 2;
What we’re doing here is stating that there are two integers: a which equals the value 1, and b which equals the value 2. To add these together, we need to add another line of code.
// printing the sum of a and b
cout << “a + b = “ << (a + b) << endl;
Let’s break this down a little. ‘Printing’ here refers to what will show on your screen once you run the program.
So this program is going to print the sum of a and b (in our case, 1 and 2). “cout” is a combination of the terms Character (abbreviated to C) and Output, which when used alongside the insertion operator (written here as <<) will display a stream of specific characters.
Finally, Endl, is what is called a manipulator. To make this simple, it’s basically a command. This is the end of a sentence, so to speak – a signifier to the operating system that a new line is about to start. In totality, our code will look something like this:
#include <iostream>
using namespace std;
int main() {
int a, b;
a = 1;
b = 2;
// printing the sum of a and b
cout << “a + b = ” << (a + b) << endl;
return 0;
}
When we run this code – providing everything has gone right – you’re likely to see this printed in the output:
a + b = 3
If you see this, you know that your program has worked and can successfully add two numbers together!
Division In C++
So now that we’ve gone over the basics of Arithmetic Operators, we can move on to how you can use what we just learned in order to perform division. Thankfully, it’s not much different to what we have just written, only you’ll need to change the operator symbol from a + sign to a /.
You might be wondering why we’re not using the actual mathematical symbol for division here – well, most keyboards don’t feature this, so instead C++ just uses /. This is how your program is going to look if we want to divide two numbers.
#include <iostream>
using namespace std;
int main() {
int a, b;
a = 6;
b = 2;
This time we’ve used the integers 6 and 2 to make things more simple for our first division. Now let’s add that first part on to our longer code.
#include <iostream>
using namespace std;
int main() {
int a, b;
a = 6;
b = 2;
// printing the sum of a and b
cout << “a / b = ” << (a / b) << endl;
return 0;
}
Notice here we have changed the + symbol we used before for the operator for division. So it’s a / b =. When you run your program, this is what is going to be output:
a + b = 3.
Final Thoughts
So there you have it! Arithmetic Operators are a simple part of coding in C++ that is important for you to learn.
From, here, you should be able to take the concept and play around with it. It’s worth noting that you can chain these different operators together to have the integers be added, subtracted, multiplied, and divided at the same time.
If you want to further understand Arithmetic Operators, you can learn about the % operation (Modulo Operation), which is most commonly used in low-level programming, but worth learning about!
We hope that this article has broken the concept down to you in a simple way and that you’re now one step closer to becoming a C++ pro!
If you have any problems with your program, make sure you check over everything or else research further. Usually, the solution is just one search away!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.