Asyncio and threading are two widely used techniques in Python for concurrent programming.
In this blog post, we will contrast Python threading versus asyncio in great detail. We’ll look at how these approaches differ from one another, their benefits and drawbacks, and instances from the real-world showing when to employ each approach instead of the others.
Building efficient and productive applications requires an awareness of the distinctions between asyncio and threading, regardless of your programming experience with Python.
You’ll have thorough knowledge of these strategies by the end of this blog post, along with advice on how to select the one that most closely matches your programming requirements. So let’s start now!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Asyncio vs. Threading: The Short Answer
Asyncio and threading are two approaches for concurrent programming in Python. Asyncio is ideal for I/O-bound tasks, while threading is better suited for CPU-bound tasks. The choice between them depends on the specific requirements of your project and the type of task you need to perform.
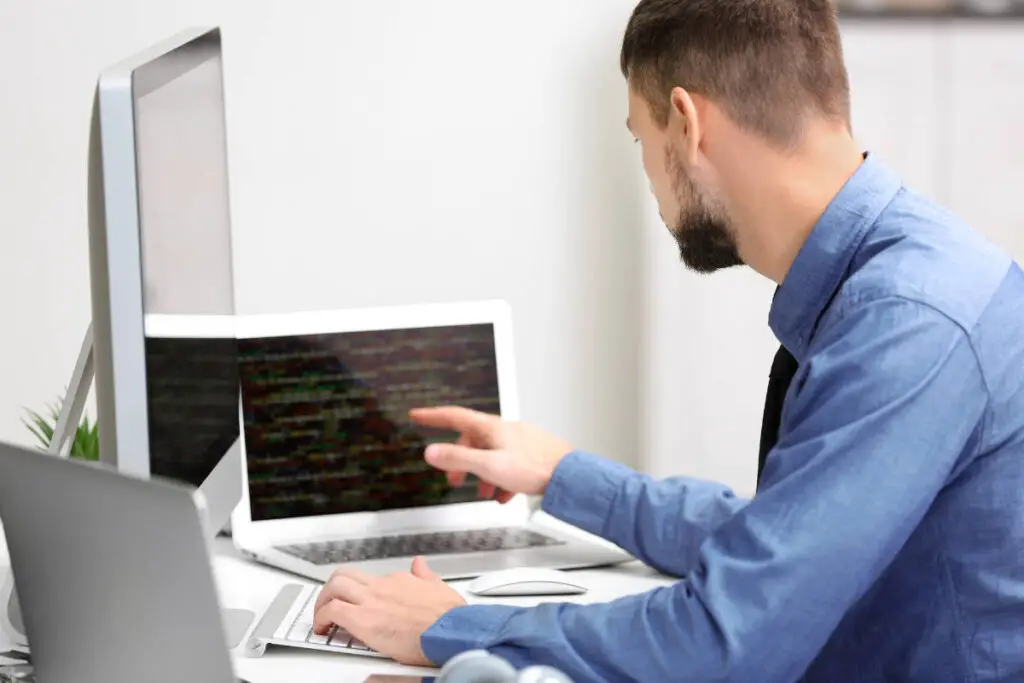
Area | Asyncio | Threading |
---|---|---|
Task Types | I/O-bound | CPU-bound |
Code Design | Coroutines, Event Loop | Multiple Threads |
Performance | Scales well for I/O-bound tasks, less for CPU-bound | Scales well for CPU-bound tasks |
Debugging | Can be more challenging than threading | Can be prone to synchronization issues |
Context Switching | Can incur overhead | Can incur overhead |
Scalability | Designed to be highly scalable for I/O-bound tasks | Can become less efficient with a large number of threads |
Ease of Use | Can be more complex than threading | Can be easier to understand and implement |
Real-world Applications | Ideal for network operations, web servers, and asynchronous I/O | Suitable for CPU-intensive tasks, GUI applications, and I/O |
Synchronization Primitives | Coroutines and event loops | Locks and semaphores |
Profiling Tools | Built-in profiler, cProfile | cProfile |
Third-party Libraries | aiohttp, uvloop, curio, trio | N/A |
What do Python’s Asyncio and Threading concepts mean?
Python concurrent programming can be done using either threading or asyncio. Let’s investigate them in greater detail:
Asyncio is a built-in Python module that was first made available in Python 3.4 and offers a productive way to create asynchronous programming using coroutines.
It is made to be scalable and lightweight, enabling high concurrency and parallelism. Coroutines, which are functions that can halt and resume their execution at particular places, are managed by Asyncio via an event loop.
Several threads of execution can operate concurrently within a single process thanks to threading, a low-level approach to concurrency.
Threads are autonomous execution units that have access to and control over the same data as well as the same memory. Within your Python code, you can create and manage threads using the threading module.
In the chapters that follow, we will contrast these strategies in further detail, examining their benefits and drawbacks as well as offering real-world instances of when to employ each strategy instead of the others.
Contrasting Asyncio and Threading in Various Contexts
To provide a more thorough knowledge of the differences and similarities between Asyncio and Threading, we shall examine them in various contexts in this chapter.
Performance
Asyncio can execute many operations concurrently within a single thread, making it generally more efficient for I/O-bound activities.
Contrarily, because threading may utilize many CPU cores, it is better suited for CPU-bound jobs that demand intensive calculation.
Ease of Use
Asyncio can be more challenging to learn and use than threading because it necessitates working knowledge of coroutines and event loops.
But if mastered, it can result in more scalable and effective programming. On the other hand, threading is comparatively simple to comprehend and use but can be vulnerable to synchronization problems like race situations.
Scalability
Asyncio can handle many concurrent activities without spawning new threads, making it a very scalable design.
The expense involved in handling many threads, on the other hand, means that threading may become less effective as the number of threads rises.
Debugging
Troubleshooting asyncio code can be more difficult than debugging threaded code since it calls for knowledge of event loops and coroutines.
On the other hand, threading can be vulnerable to synchronization problems and race circumstances, which can be challenging to debug.
Asyncio and threading both offer advantages and disadvantages, so which one you choose ultimately depends on the particular needs of your project.
For performance and scalability, Asyncio can be the superior choice if your project requires I/O-bound processes. Using threading to make use of several CPU cores may be the superior choice if your project contains CPU-intensive tasks.
Examples from the real world: When to utilize Asyncio and Threading
This chapter will give concrete examples of when to use threading and when to utilize asyncio in applications that are seen in the real world.
Network Operations
Network operations that require sending queries to external servers and waiting for a response are best suited for asyncio. For instance, Asyncio’s capacity to manage many I/O-bound tasks concurrently can be advantageous to a web scraper that must make multiple queries to retrieve data from various sources.
Although it might not be as effective as Asyncio in this situation, threading may still be appropriate.
CPU-intensive tasks
Tasks that require a lot of computing, such as mathematical calculations or image processing, are better suited for threading.
In these circumstances, using multiple threads to distribute the workload among several CPU cores might speed up computing. As Asyncio is made for I/O-bound operations, it might not be as useful in this situation.
GUI Applications
Asyncio was created for console-based applications. Hence GUI apps are not a good fit for it. As threading enables for responsive user interfaces while carrying out background operations, it is better suited for GUI applications.
Web Servers
Asyncio works well with web servers that manage many concurrent connections, like web sockets or long-polling connections. In this situation, threading can also be employed, although as the number of threads rises, it may become less effective.
Guidelines for using Python’s asyncio and threading
It’s crucial to adhere to a few best practices while using asyncio or threading in Python to make sure your code is effective, dependable, and maintainable.
Here are some ideas to think about:
- Avoid blocking calls: In asyncio, blocking calls can cause performance to suffer and slow down the event loop. Use async versions of libraries that enable asyncio to avoid blocking calls, or use threads for blocking operations. Similar to this, avoid blocking calls in the main thread while threading because they may cause the user interface to become unresponsive.
- Use synchronization primitives: When threading, race situations can be avoided and shared resources are safely accessed by using synchronization primitives like locks and semaphores. Coroutines and event loops can be used to synchronize in asyncio.
- Context switching should be avoided because it can impede performance in both asyncio and threading. Use a suitable number of threads or coroutines and think about using a thread or coroutine pool to prevent frequent context switching.
- Utilize the right data structures: Decide which data structures are best for the task at hand. For activities that need a lot of I/O-bound operations, employing a deque in asyncio can boost speed.
- Use profiling tools: To find performance bottlenecks and improve your code, use profiling tools like cProfile or the built-in profiler in asyncio.
- Think about using third-party libraries: Third-party libraries can streamline routine activities and offer extra capabilities. For instance, the widely used asyncio-based HTTP client and server software known as aiohttp can make handling HTTP requests and responses easier.
FAQ
Is threading preferable than asyncio?
The response is based on the particular needs of your project. For I/O-bound operations, asyncio is typically more effective, whereas threading is better suited for CPU-bound workloads.
It’s critical to comprehend the advantages and disadvantages of both strategies before deciding which one best suits your programming requirements.
What distinguishes Python’s async IO from multithreading?
In Python, there are two methods for concurrency: asyncio and multithreading. While multithreading executes work concurrently through multiple threads of operation, asyncio manages tasks using coroutines and an event loop.
For I/O-bound operations, asyncio is typically more effective, but multithreading is better suited for CPU-bound workloads.
Do threads get used in Python asyncio?
No, threads are not automatically used by asyncio. Instead, it manages tasks and coroutines using an event loop.
Why is Python not a good choice for threading?
It might be challenging to debug synchronization problems and race scenarios when using threading in Python. Furthermore, managing and creating threads can add overhead and impair efficiency.
For these reasons, Python concurrent programming does not always benefit from the use of threading.
Why is asyncio not thread-safe?
Because Asyncio manages tasks and coroutines using an event loop, which can be corrupted if accessed concurrently from different threads, it is not thread-safe. Asyncio offers synchronization primitives like locks and semaphores to prevent this.
Conclusion
We have examined the distinctions and parallels between Python’s asyncio and threading in this blog post. As we’ve seen, each strategy has advantages and disadvantages, and which one you choose ultimately relies on the particular needs of your project.
For I/O-bound operations, asyncio is typically more effective, whereas threading is better suited for CPU-bound workloads. Whereas threading uses multiple execution threads, asyncio manages tasks using coroutines and an event loop.
You can use Python’s asyncio and threading to develop safe, efficient programs by adhering to best practices including avoiding blocking calls, leveraging synchronization primitives, and using the right data structures.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.