As a programmer, you know that working with files in Python can be a real challenge.
Fear not!
Checking for end of file (EOF) shouldn’t be one of those issues. Mastering EOF can improve code efficiency and quality. This article will teach you how to verify EOF in Python.
Let’s start!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is End of File (EOF) and why is it Important?
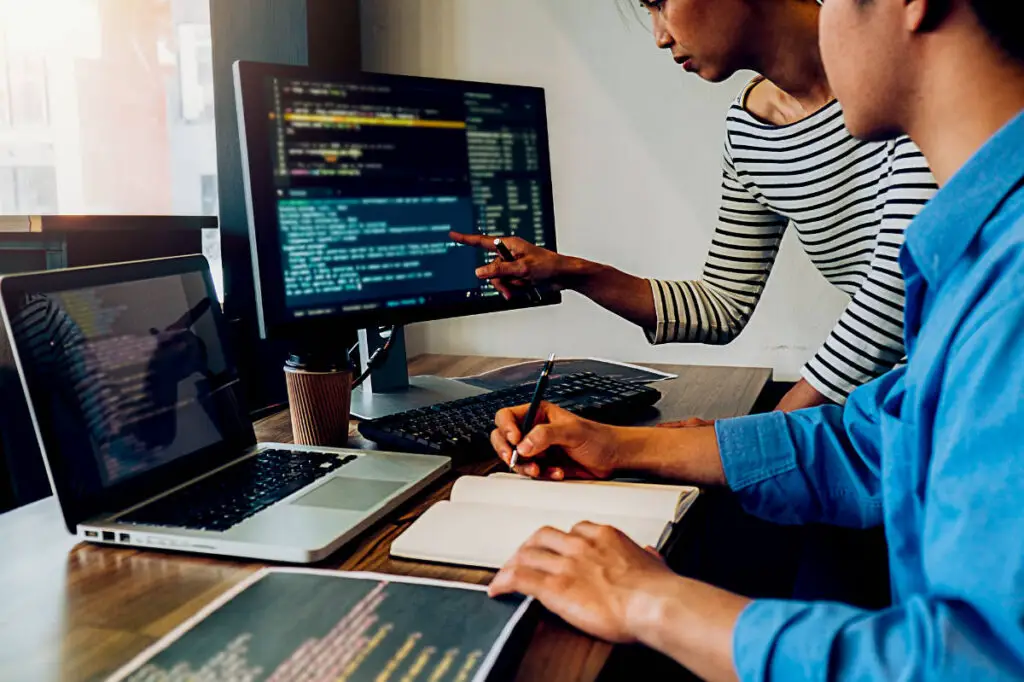
Have you ever had trouble attempting to use Python’s end of file (EOF) check? Since it is a common issue, do not worry! We’re here to assist you in comprehending and overcoming it.
What exactly is end of file (EOF) then?
It serves as a file’s final file marker, essentially. When working with files in Python, knowing when you’ve reached the end of a file is essential.
But occasionally, performing an EOF check might be challenging and even result in unanticipated errors. Herein is the value of this article.
We’ll explain what EOF is, why checking for it in Python can sometimes cause problems, and what it implies. You’ll have a thorough understanding of the issue at hand by the end of this part, and you’ll be better prepared to address it head-on.
How to Find out Whether a File is at its EOF in Python?
When it comes to checking for the end of file (EOF) in Python, there are a few different methods you can use. Let’s explore each one in detail:
#1: Built-in functions
Python provides several built-in functions for checking EOF, including the readline()
and read()
methods.
These methods are easy to use and can be helpful in many scenarios.
Here are some pros and cons of using built-in functions:
Pros:
- Easy to use and understand
- Can handle most basic cases of EOF
- Good for reading files with a known structure
Cons:
- May not work well for large files
- May not be the best choice for complex file structures
- Can be slow for some use cases
#2: Custom methods
If you need more control over how EOF is checked, you can create your own custom method.
This method can be tailored to your specific use case and give you more flexibility in your code.
Here are some pros and cons of using custom methods:
Pros:
- More control over EOF checking
- Better suited for complex file structures
- Can be faster for some use cases
Cons:
- More difficult to create and maintain
- May not be necessary for basic use cases
- May require more technical knowledge
When to use each method:
- Use built-in functions for simple file structures and when speed is not a concern
- Use custom methods for complex file structures or when you need more control over EOF checking
It is worth mentioning that verifying the end of file (EOF) in Python can affect the system’s performance when dealing with large files.
For instance, if you check for EOF at the end of every line in a file that contains millions of lines, it can significantly slow down the overall runtime of your script.
To improve system performance in such situations, you can create custom methods for EOF verification, like scanning the file header.
Considering the advantages and disadvantages of each method can help you devise a successful approach to EOF checking in Python that suits your requirements the best
Looking for a step-by-step guide to learn coding?
Practical examples
Let’s take a look at some practical examples of how to implement each method.
First, we’ll use the readline()
method to check for EOF in a simple file structure:
with open('myfile.txt', 'r') as f: line = f.readline() while line: # process line line = f.readline()
Next, let’s use a custom method to check for EOF in a more complex file structure:
def custom_eof(file): # read the last byte of the file file.seek(-1, 2) last_byte = file.read() # if the last byte is EOF, return True if last_byte == b'\n': return True else: return False with open('myfile.txt', 'rb') as f: eof = custom_eof(f) if eof: print('Reached EOF!')
Conclusion: Checking for EOF in Python Made Easy
Congratulations, you’ve made it to the end of our guide to checking for the end of file (EOF) in Python! Let’s recap what we’ve learned:
- EOF is an important marker that indicates the end of a file
- Checking for EOF is critical when working with files in Python
- Python provides built-in functions and custom methods for checking EOF
- Built-in functions are easy to use and good for simple file structures, while custom methods give you more control and flexibility
- When choosing which method to use, consider your specific use case and file structure
- Practical examples can help you better understand how to implement each method
- By following the tips and tricks in this guide, you’ll be able to confidently check for EOF in Python and write more efficient and error-free code.
So go forth and conquer your file handling challenges with ease!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.