Programming often uses arrays, which store multiple values of the same data type.
Checking if all array elements are equal may be necessary. Data analysis, machine learning, and scientific computing use this task.
This blog post will discuss Python solutions for checking if all array elements are equal. To help you implement each solution in your code, we will provide practical examples.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is an Array in Python?
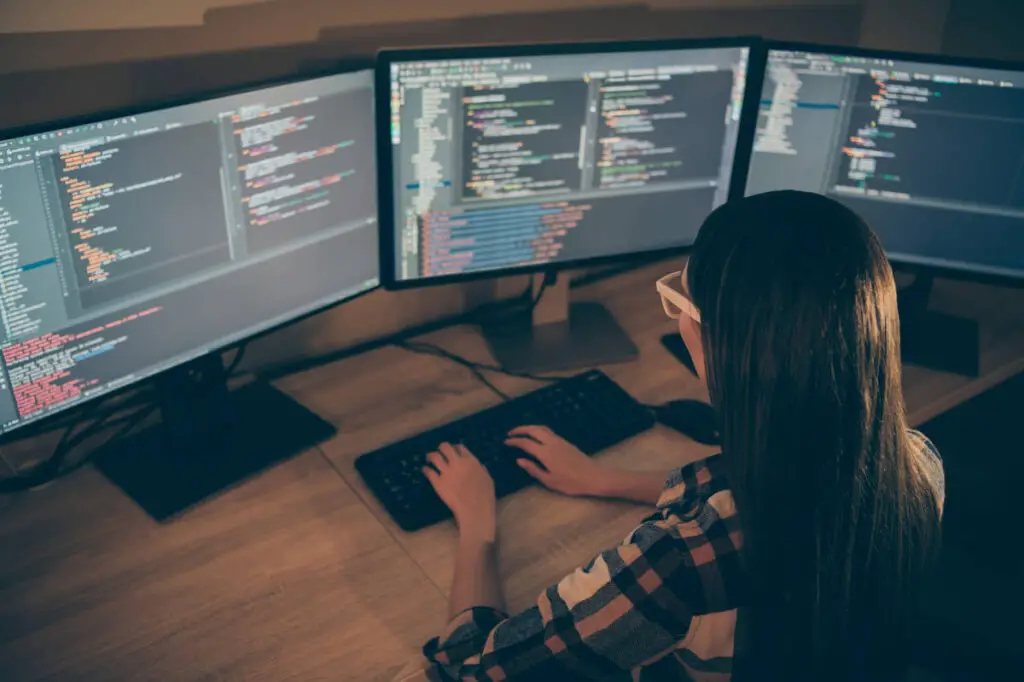
Let’s first take a moment to grasp what an array is and why we might need to check if all elements in an array are equal before moving on to the solutions for the problem of determining whether all elements in an array are equal.
An array in Python is comparable to a container that may hold numerous values of the same type. These values could be anything, including words, integers, or even other arrays. In an array, each value has a unique index that starts at zero.
Now picture yourself dealing with a dataset that has information about people’s heights. You might wish to know if each column’s heights are uniform. This is where the issue of determining whether or not every element in an array is equal arises.
We need to figure out how to compare different values in an array to determine if they are all the same. Several programming situations, including data analysis, machine learning, and scientific computing, can make use of this issue.
We’ll look at a number of approaches that can quickly and accurately determine whether every element in an array is equal in the section that follows.
Check If All Elements In Array Are Equal – Solutions and Practical Examples
Let’s investigate alternative Python solutions to the issue of determining whether all elements in an array are equal now that we have a deeper understanding of the issue.
Together with the advantages and disadvantages of utilizing each approach, we will provide code examples for each solution.
Looking for a step-by-step guide to learn coding?
#1: Comparing Each Element to the Initial Element Using a Loop
The first approach entails iteratively traversing the array and contrasting each element with the first element. This approach is simple to understand and simple to use.
Nevertheless, if the array is huge or the comparison is performed numerous times, this technique may not be efficient. Moreover, the array must include hashable elements in order for this method to function.
def check_all_elements_equal(arr): for i in range(1, len(arr)): if arr[i] != arr[0]: return False return True # Example usage array1 = [1, 1, 1, 1, 1] array2 = [1, 2, 1, 1, 1] print(check_all_elements_equal(array1)) # Output: True print(check_all_elements_equal(array2)) # Output: False
#2: Use of the Count() Method as a second option
One can count the instances of a specific element in an array using the count() method. We can check to see if all the array’s elements are the same by using this function to the array’s initial element.
This approach is effective and can be used with elements that can be hashed and those that cannot. It can only be used, though, if every element in the array is the same data type.
Example in practice:
def check_all_elements_equal(arr): return arr.count(arr[0]) == len(arr) # Example usage array1 = [1, 1, 1, 1, 1] array2 = [1, 2, 1, 1, 1] print(check_all_elements_equal(array1)) # Output: True print(check_all_elements_equal(array2)) # Output: False
#3: Using the Set() Method as Solution 3
Duplicates in an array can be removed using the set() method. After using set(), all elements in an array are identical if there is just one unique element left.
This approach is effective and can be used with elements that can be hashed and those that cannot. It might not preserve the array’s original element order, though, and huge arrays may require a lot of RAM.
def check_all_elements_equal(arr): return len(set(arr)) == 1 # Example usage array1 = [1, 1, 1, 1, 1] array2 = [1, 2, 1, 1, 1] print(check_all_elements_equal(array1)) # Output: True print(check_all_elements_equal(array2)) # Output: False
#4: Utilizing the Numpy Library
The “np.all()” method from the Numpy library determines whether every element in an array is equal. Any size and data types of arrays can be used with this approach.
This approach is effective and can be used with elements that can be hashed and those that cannot. However, installing the Numpy library is necessary.
import numpy as np def check_all_elements_equal(arr): return np.all(arr == arr[0]) # Example usage array1 = np.array([1, 1, 1, 1, 1]) array2 = np.array([1, 2, 1, 1, 1]) print(check_all_elements_equal(array1)) # Output: True print(check_all_elements_equal(array2)) # Output: False
You will have a thorough understanding of the numerous approaches to the Python problem of determining whether all elements in an array are equal by the end of this session.
Conclusion
In conclusion, a common issue in Python programming is determining if all entries in an array are equal. Fortunately, there are several options available to effectively tackle this issue.
Using a loop to compare each element with the first element, the count() method, the set() method, and the numpy library were the four approaches we looked at in this article.
The ideal way will rely on the particular use case and the characteristics of the array being studied. Each method has pros and limitations of its own.
You can pick the greatest strategy for your particular programming wants by comprehending these solutions and their actual applications.
We trust that this chapter has given you a thorough grasp of how to determine whether all array members are equal in Python. Coding is fun!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.