Python is a popular programming language that allows users to create dynamic and efficient code.
As with any programming language, it’s important to understand how to work with variables, including clearing them when necessary. This section covers the different methods for clearing variables in Python.
Whether you’re a beginner or an experienced developer, our guide will provide you with the best practices and examples to help you easily clear a variable in Python.
Key Takeaways:
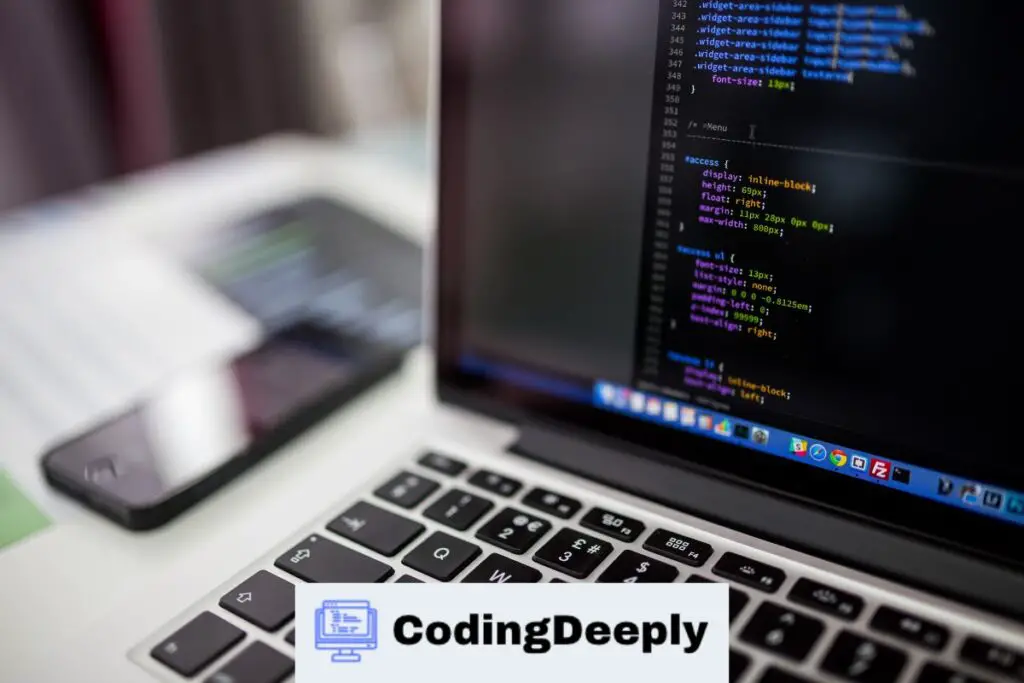
- Clearing a variable in Python involves either assigning a new value or using the ‘del’ keyword to delete the variable.
- Understanding how variable deletion works in Python is important for writing efficient code.
- Clearing a variable in Python may vary depending on specific use cases.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Variable Deletion in Python
Before we dive into the methods of clearing variables in Python, let’s first explore the concept of variable deletion. In Python, variables are dynamically typed, meaning that the variable type is inferred at runtime.
This contrasts statically typed languages like C++ or Java, where the variable type must be explicitly stated.
In Python, when any part of the program no longer references a variable, it becomes eligible for garbage collection. Garbage collection is the process of automatically deallocating memory that the program no longer uses.
When a variable is garbage collected, the allocated memory is freed up and can be used for other purposes.
Note that just because a variable is no longer being used by the program doesn’t necessarily mean that it will be garbage collected immediately. Garbage collection is handled by the Python interpreter, which periodically checks for unused memory and frees it up as needed.
One implication of this dynamic typing and garbage collection is that Python doesn’t provide a way to destroy a variable explicitly.
Instead, the Python interpreter keeps track of which program still uses variables and deallocates memory automatically as needed.
Clearing Variables in Python: Method 1
There are different ways to clear a variable in Python, and the first method involves assigning a new or empty value to the variable.
This approach is useful when you want to reset the variable to its initial state or change its data type.
For example, let’s say you have a variable count that stores the number of times a user has logged in. If you want to reset this count to 0, you can assign the value 0 to the variable:
count = 0
Alternatively, you can assign an empty value to the variable, depending on its data type. For strings, you can use an empty string (''
), for lists and dictionaries, you can use an empty set of brackets ([]
and {}(respectively), and you can use a value of 0 or 0.0 for numeric types
.
It’s important to note that assigning a new value to a variable may not completely “clear” it if there are other references to the same value in your code.
In such cases, you may need to use the del
keyword to remove the reference to the variable altogether, which we’ll cover in the next section.
Clearing Variables in Python: Method 2
Another approach to clearing variables in Python is to use the ‘del’ keyword. This method explicitly deletes the given variable, and any reference to it will result in a NameError.
The syntax of the ‘del’ statement is straightforward. Type the keyword followed by the variable name you wish to delete. For instance:
number = 5
del number
After executing this code, you can no longer use the ‘number’ variable. If you try to reference it in any way, Python will raise a NameError.
It’s worth noting that deleting a variable explicitly isn’t always necessary or recommended. In general, it’s better to let Python’s garbage collector handle variable deletion when the variable goes out of scope.
However, there are some use cases where the ‘del’ keyword is particularly useful. For example, in a long-running program with limited memory resources, you may need to explicitly delete large data structures or variables to reduce memory usage.
Overall, deciding which variable-clearing method to use in Python depends on the context of your code and your specific needs.
Method 2 allows you to delete variables when necessary explicitly, but it’s important to understand their implications.
Conclusion
In conclusion, we’ve explored different methods to clear a variable in Python. Whether you choose to assign a new value or delete the variable using the ‘del’ keyword, it’s important to understand the implications of each method.
Choose the Right Method
When clearing a variable, consider your specific requirements and the context of your code. If you want to reset the value of a variable, assigning a new or an empty value is a common and easy method.
However, if you need to completely delete the variable to free up memory or to avoid potential errors, using the ‘del’ keyword might be a better option.
Write Efficient Code
Clearing variables is a common task in Python programming.
Understanding variable deletion and the different methods to clear variables can help you write more efficient and effective code. By optimizing your code, you can improve its performance and reduce the risk of errors or bugs.
So, next time you need to clear a variable in Python, remember the options we’ve covered and choose the best method for your code.
FAQ:
How can I clear a variable in Python?
There are multiple methods to clear a variable in Python. You can either assign a new value or an empty value to the variable, or use the ‘del’ keyword to delete it.
What is a variable deletion in Python?
Variable deletion refers to removing a variable from memory in Python. When a variable is deleted, its reference is removed, and its occupied memory becomes available for other purposes.
How does clearing a variable using method 1 work?
Method 1 involves assigning a new or empty value to the variable. This can be achieved by using the assignment operator (=) to assign a new value or an empty value such as None or an empty string.
What is the syntax for clearing a variable using method 2?
In method 2, you can clear a variable by using the ‘del’ keyword followed by the variable name. For example, ‘del my_variable’ will delete the variable ‘my_variable’.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.