Are you struggling with managing CSV files in Python? Do you need to write a Python list to a specific column in a CSV file? Look no further; we have a simple and efficient solution for you.
This section will guide you through writing a Python list to a specific column in a CSV file. Following our step-by-step approach, you can streamline your data management process and manipulate CSV files effortlessly.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Manipulating CSV Files in Python
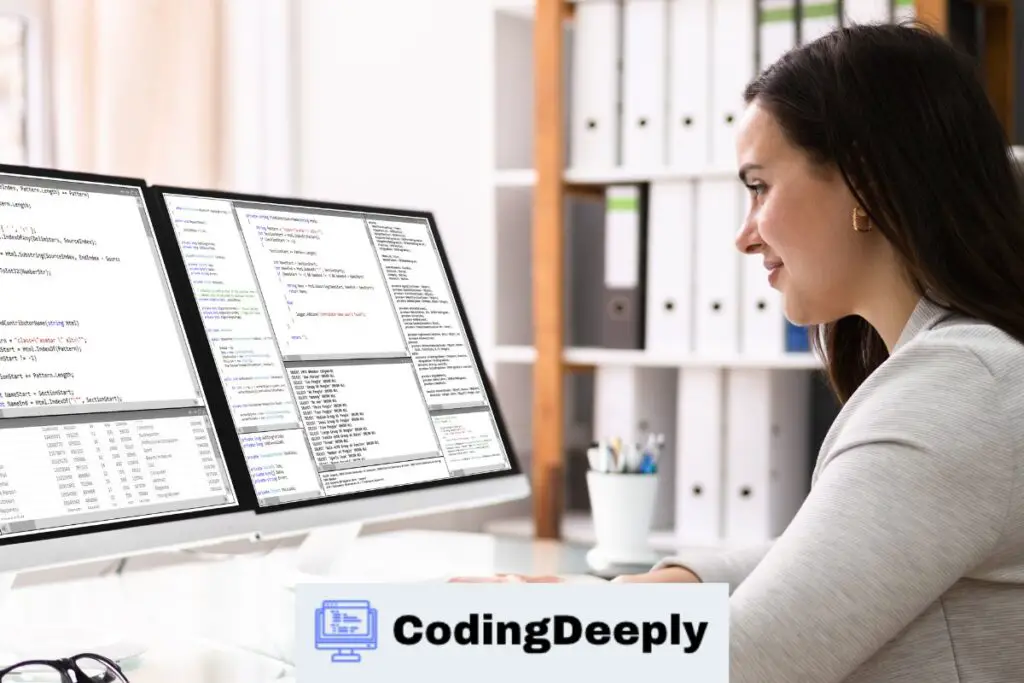
To write a list to a CSV column in Python, it is necessary to understand how to manipulate CSV files using Python’s built-in CSV library. This library provides functionality for reading and writing data to and from CSV files, making it a powerful tool for managing tabular data.
Python CSV Library Tutorial
The first step in manipulating CSV files in Python is to import the CSV library. This can be done using the following code:
import csv
Once the library is imported, you can open a CSV file using the following code:
with open('filename.csv', 'r') as file:
Note: The ‘r’ argument indicates that the file is being opened in read-only mode. To open a file in write mode, use ‘w’ instead.
Once the file is open, you can read and write data using the csv.reader and csv.writer objects. These objects provide methods for reading and writing data to and from CSV files, respectively.
Handling CSV Files in Python
Several important operations can be performed on CSV files using the Python CSV library.
These operations include:
- Reading data from a CSV file using the csv.reader object
- Writing data to a CSV file using the csv.writer object
- Appending data to a CSV file using the csv.writer object
- Filtering data in a CSV file using the csv.reader object
Each of these operations can be performed using the appropriate method provided by the CSV library. It is important to read the documentation carefully to ensure the correct method is used for your specific use case.
Writing Data to a Specific Column in a CSV File
To write data to a specific column in a CSV file using Python, you must use the CSV writer object, available in Python’s built-in CSV library.
Here’s an example of how you can use the CSV writer object to write a list to a specific column in a CSV file:
import csv # Create a list of values to write data = ['value1', 'value2', 'value3'] # Open the CSV file in write mode with open('example.csv', 'w', newline='') as file: # Create a CSV writer object writer = csv.writer(file) # Write each value to a separate column for value in data: writer.writerow([value])
In this example, we first import Python’s built-in CSV library. We then create a list of values to write to the CSV file, and open the file in write mode using the ‘w’ option. We also set the newline parameter to an empty string to avoid issues with line endings.
Next, we create a CSV writer object by calling the csv.writer() function and passing it our file object. We then loop through the list of values, and write each value to a separate row in the CSV file using the writer.writerow() method.
Writing Data to a Specific Column
If you want to write data to a specific column in a CSV file, you need to slightly modify the way you use the CSV writer object.
Here’s an example of how you can write a list of values to a specific column in a CSV file:
import csv # Open the CSV file in write mode with open('example.csv', 'w', newline='') as file: # Create a CSV writer object writer = csv.writer(file) # Write each value to the second column for value in data: writer.writerow(['', value])
In this example, we open the CSV file in write mode as before, and create a CSV writer object. We then loop through our list of values, and use the writer.writerow() method to write each value to the second column in the CSV file.
Note that we write an empty string to the first column before writing our actual value. This is because CSV files are structured as rows and columns, and each row must have the same number of columns. If we didn’t write anything to the first column, our data would be misaligned with the other rows in the file.
Best Practices for Writing to a CSV Column
Writing data to a CSV column is a common task when working with data in Python. It’s important to follow best practices to ensure accurate and efficient results. Here are some tips and tricks for writing data to a specific column in a CSV file using Python.
1. Use the CSV Writer Object
The CSV writer object is a built-in Python module that simplifies writing to CSV files. It automatically formats the data and handles special characters. Use the writer object to write to columns in a CSV file.
2. Include Headers
Headers are the names of the columns in a CSV file. Including headers is essential to ensure that the data is organized and easily readable. Use the writerow() function to write the headers to the CSV file.
3. Open the File in Binary Mode
It’s important to open the file in binary mode when working with CSV files. This ensures that special characters and encoding are handled correctly. Use the ‘wb’ mode when opening the file in Python.
4. Avoid Using Comma Separated Values in Data
While writing data to a CSV column, avoid using commas in the data values. Comma separation is used to separate different values in a CSV file. If the data contains commas, your program may confuse where one value ends and another begins.
5. Use Exception Handling
Exception handling is a crucial part of writing to CSV files in Python. It prevents your program from crashing if an error occurs while reading or writing to the file. Use try-except statements to handle exceptions.
6. Test Your Code
Before moving on to the next step, testing your code is essential. Write a small number of records to the CSV file to check that the data is being written to the correct column and that the file is formatted correctly. This will save time and hassle in the long run.
By following these best practices, you can streamline your data management process and ensure that your CSV files are accurate and easy to work with.
FAQ – Writing a Python List to a CSV Column
1. What is the most efficient way to write a Python list to a CSV column?
You can easily write a list to a specific column in a CSV file using the Python CSV writer. Following the steps outlined in this article, you can streamline your data management process and manipulate CSV files effortlessly.
2. How do I manipulate CSV files in Python?
To manipulate CSV files in Python, you can use the built-in CSV library that provides essential operations required for writing data to a CSV file. This article includes a tutorial on CSV file manipulation in Python to help you get started.
3. Can I write data to a specific column in a CSV file using Python?
You can write data to a specific column in a CSV file using Python’s CSV writer. The step-by-step example provided in this article will give you practical knowledge to implement this method in your own projects.
4. What are some best practices for writing to a CSV column?
When writing data to a CSV column, it’s essential to follow best practices to ensure accurate and efficient results. Some tips and tricks include creating a header row, using an appropriate delimiter, and avoiding special characters. This section details best practices for writing data to a specific column in a CSV file using Python.
5. Can I write a Python list to multiple columns in a CSV file?
You can write a Python list to multiple columns in a CSV file by creating a nested list and using the CSV writer’s writerows() method. This article focuses on writing data to a specific column, but you can easily modify the code to write data to multiple columns.
6. Is it possible to append data to an existing CSV file using Python?
You can use Python’s built-in method “a” to append new data to an existing CSV file. This section provides a brief example of appending data to an existing CSV file using Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.