The concept of “null” can be confusing when you first start off in Java.
Does it mean that something has gone wrong? Perhaps a code line is broken? Or maybe the language is telling you that nothing is there?
We will walk you through the basics of “null” and straighten out any confusion you may have around the subject.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
The Basics – Explaining Null
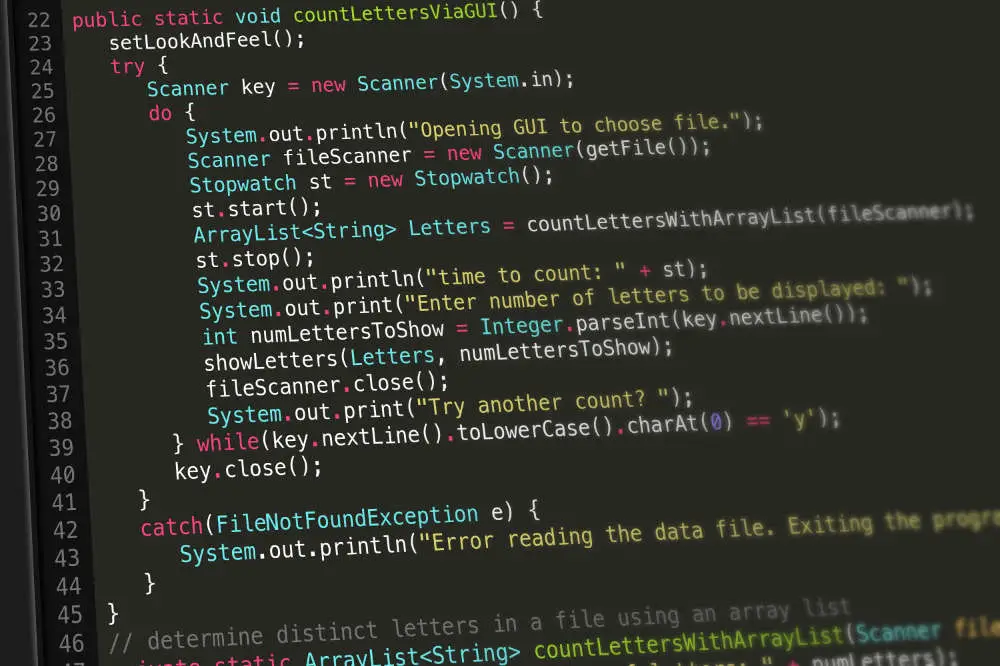
In short, “null” is the reserved keyword you use to suggest that there is no value.
A lot of new starters confuse “null” as a type or an object, but it should be thought of as a value (or lack thereof).
You would use “null” to indicate a lack of something or the non-existence of something.
As a reserved keyword, “null” basically acts as a true or false statement. If the value is false or doesn’t exist, the outcome will be “null”.
Behind The Scenes – What Null Does
For “null” to work properly, the script must know what it should be looking for. Without a variable for it to point towards, the “null” won’t know what to expect an absence of.
You can consider variables in two main ways, primitives and references. We will explain them both to show you how “null” can interact with them.
Primitive Variables
Primitive variables are a set of data pre-defined by the language programming, in this case, Java.
When you acknowledge or declare a primitive variable, it will contain the expected value for “null” to consider. Examples of primitive variables are “float” and “int”.
Reference Variables
References are considered a type of pointer. They show you the value being represented.
Declaring a reference means you’ve stored the address, directing toward the value in question. It doesn’t point toward the value directly. For example, strings, arrays, and classes are all reference types.
Null And Its Variables
Primitive variables aren’t able to use “null” values. Attempting such will create an error message. Reference variables, however, can have “null” assigned to whatever type you choose.
Null And String
An example of “null” with a string reference could look like this – String myStr = null;
Null And Integers
Although integers are a primitive variable, you can assign null to them, however, you would need to assign it through a reference variable instead of directly.
An example of “null” with an integer reference could look like this – Integer a = null;
You would not write it as – int myInt = null;
If you try to use “null” to point to a primitive variable, you will receive an error message similar to this – incompatible types : required int found null
The Easiest Mistake To Make With Null
Now you understand what “null” is, it’s time to understand it with Java.
As with any programming language, each one will have its own spin on central processing. For “null” the nuance of Java isn’t complicated.
“Null”, just like every keyword in Java, is sensitive to case or capitalization usage. If you write “Null” or “NULL”, you will be given errors. Instead, you need to write “null” in all lowercase.
For a visual representation, look at these examples.
- Integer Int = null;
- With this line, you will be given a null value.
- Integer errInt = NULL;
- With this line, you will be given the error – compile-time error : can’t find symbol ‘NULL’
The Easiest Way To Use Null
The easiest way to use “null” correctly, is to recognize it as the default. When looking at primitive variables, the default is often 0 or false, depending on if you are using integer, booleans, etc).
When looking at reference variables, the value is “null”. This is the same for static variables and instance variables too.
Null As A False Return
If you ever get confused about if an object can be considered as an interface, subclass, or class, you can use the “instanceOf” operator to check.
However, if you use this hint with a reference variable, you will be given a false return. Be aware of this difference.
Explaining NullPointException
If you forget to or simply don’t initialize the variables, you may be shown this error message – java.lang.NullPointerException
This mistake can happen easily, as “null” is the expected default for any uninitialized reference variable.
However, there is more than one way to receive this message. You may fall into this trap if you do the following:
- By modifying or accessing a member or data field with “null”.
- Treating “null” as an array in Java.
- Using a “null” in a method as an argument.
- Attempting to synchronize a “null”.
- Trying to involve a method using a “null”.
In simple terms, all variables need to be initialized correctly, which means that all objects need to have reference points leading to values considered valid.
Here are some ways to avoid the Null PointEr exception error.
Choosing A Return Of Returning Empty Collections
If you hate the idea of null, and would rather something else instead, we suggest trying empty collections. To do this, follow this method:
public class emptyString {
private static List<Integer> numbers = null;
public static List<Integer> getList() { if (numbers == null)
return Collections.emptyList(); else
return numbers;}}
Ternary Operators
Perhaps you would like an “empty when not in use” function instead. In that case, follow this method:
//boolean expression ? value1 : value2;
String myStr = (str == null) ? "" : str.substring(0, 20);
//If str’s reference is null, myStr will be empty. Else, the first 20 characters will be retrieved.
Summary
Understanding how “null” works and why it is important, will help you navigate through the language of Java with fewer error messages.
“Null” is a powerful tool, and shouldn’t be disregarded as a nuisance to get rid of, as it is a central part of the Java programming language.
Use our examples above directly or modify them to create a more accurate creation in your script.
This information is based solely on the computer language Java, so keep that in mind when considering other languages.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.