Abstract classes are very useful in Python programming. They allow us to create new types of objects without having to define their entire structure. This makes them great for creating reusable code.
Abstract classes are similar to interfaces in Java or C. They provide a common interface for subclasses to inherit from. In other words, they allow a class to be reused across multiple projects.
Abstract classes are defined using the abstract keyword. The abstract keyword indicates that the class does not contain any data members.
Instead, it provides a default implementation for some methods. Subclasses then override these methods to add additional functionality.
The abstract keyword must come before the class name. It cannot appear after the class definition.
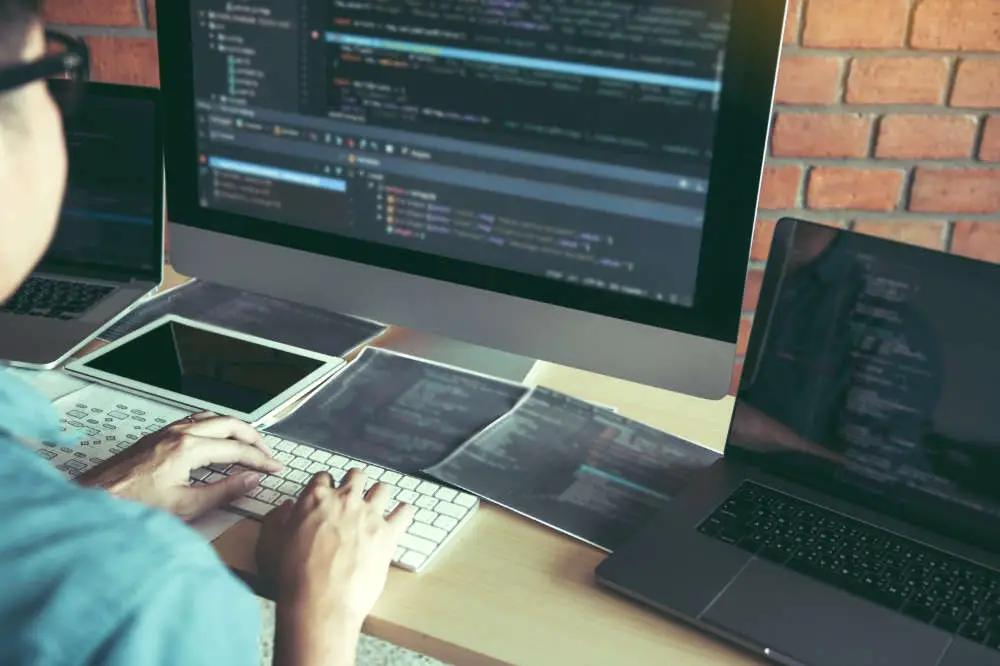
Abstract classes are used when you want to create an abstract base class.
An abstract base class is a class that defines some behavior or functionality without providing any specific implementation details. You can think of an abstract class as a blueprint for creating other concrete classes.
For example, say we wanted to make a class called Shape. We could start by defining what shapes are allowed to be created. This would be our abstract shape class. Now let’s define a concrete subclass of Shape.
This would be a circle class. In order to make sure that only circles are ever created, we need to check if the object being created is actually a Circle before allowing it to be added to the collection. For example, students struggling with similar assignments can get help with Python homework from coding experts at professional services similar to AssignmentCore.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
A Sturdy Introduction
Abstract classes are used as blueprints for other classes. You can create classes that inherit these abstract classes. They do not need to implement any methods.
Methods defined in an abstract class must have a body, but they do not need to return anything. This makes them useful for defining interfaces.
The ABC module provides you with the facilities for defining abstract base classes by using the @abstractmethod decorator.
For example, to create an abstract class, you need to use the Abstract ABC module.
An abstract class is used to force its subclasses to implement certain functions. In this case, the function feed() is implemented by all classes that inherit from Animal. We should inherit from the abstract base class, ABC.
For abstract methods, we must use a decorator: “@abstractmethod”. The built-in ABC test module contains both of these things. If we do not implement any abstract methods, we will get an error when trying to instantiate our class.
What Exactly Is An Abstract Class Python?
Abstract classes are used when we need to design a class that provides a common interface for different subclasses.
We use them when we want to provide basic functionality for different types of objects. An abstract class is defined using the keyword def. The abstract method is marked with the keyword @abtractmethod.
The concrete classes are registered as implementations of the abstract class. The output shows how to create an abstract class and register concrete classes as implementations of that abstract class.
An abstract class is a class, but not one you can instantiate directly. Abstract classes are used to describe the structure of other classes.
Methods and properties defined in an abstract class must be implemented in a child. In this example, we inherit from the ABC class, which provides some common functionality.
An abstract class is defined as having any number of abstract methods, but none of them must be explicitly declared as such.
When creating objects of an abstract class, the first step taken is calling the constructor of the base class, then the rest of the arguments passed to the superclass are used to initialize the object.
The Employee Style Class
An Employee class represents an employee. There are two types of employees: Full-Time and Hourly. The Employee class should define a property that returns the name of an employee.
This property should also return the salary of the employee. The salary calculation method should be an abstract method, since there are only two kinds of employees, which are full-time and hourly.
Full Time
The Full-time Employee class provides an implementation for the get_pay method. The salary can be initialized in the constructor of the Full-time Employee.
Hourly
The Hourly Employee class inherits from the Employee base class. You can initialize the hourly employee’s salary in the constructor.
To calculate the hourly rate and number of working hours, you multiply the hourly rate and the number of hours worked.
When Should The Abstract Class Python Be Used?
Abstract classes are used to share common properties among different classes. In this example, the full name of an employee is shared by all employees.
In order to make sure that every subclass implements the same behavior, there should be a single base class that contains some basic functionality (like a constructor).
This base class should also contain common properties and methods that all subclasses need to implement. Finally, you should always include documentation about what each property or method does.
So, What Do We Now Know About Abstract Class Pythons?
Python doesn’t provide any built-in support for abstract classes. But we can use the ABC library to implement them. An abstract class must contain at least one abstract method. Abstract methods have two forms: abstract_method and @abstractmethod decorator.
The first form defines a function that should be overridden in concrete classes. The second form is used to declare a decorated method as an abstract method. Both forms can be used together.
In this case, the decorated method is inherited by subclasses automatically.
In this case, there is no need to make the move abstract method totally abstract. Since we already defined some common things in the move abstract method, we do not need to write them again in every subclass.
We can simply call the move abstract method in the superclass using super. This makes the move abstract method more flexible and reusable.
Final Thoughts
We looked at abstract base classes and how they allow us to create an interface that describes how a class should behave without specifying how it actually behaves.
This allows us to define what a subclass must do, but still, let us use the class as if it were a normal object.
In this article, we have talked about how abstract classes can help us write code more safely by helping us avoid mistakes such as forgetting to implement a method.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.