Ever wondered why Python scripts start with #!/usr/bin/env? Dive into this guide and save yourself from common pitfalls.
You’ll benefit from the following:
- Understanding the Shebang mystery.
- Mastering cross-platform compatibility.
- Running scripts with different Python versions.
Let’s unravel the power of #!/usr/bin/env in Python scripts together!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
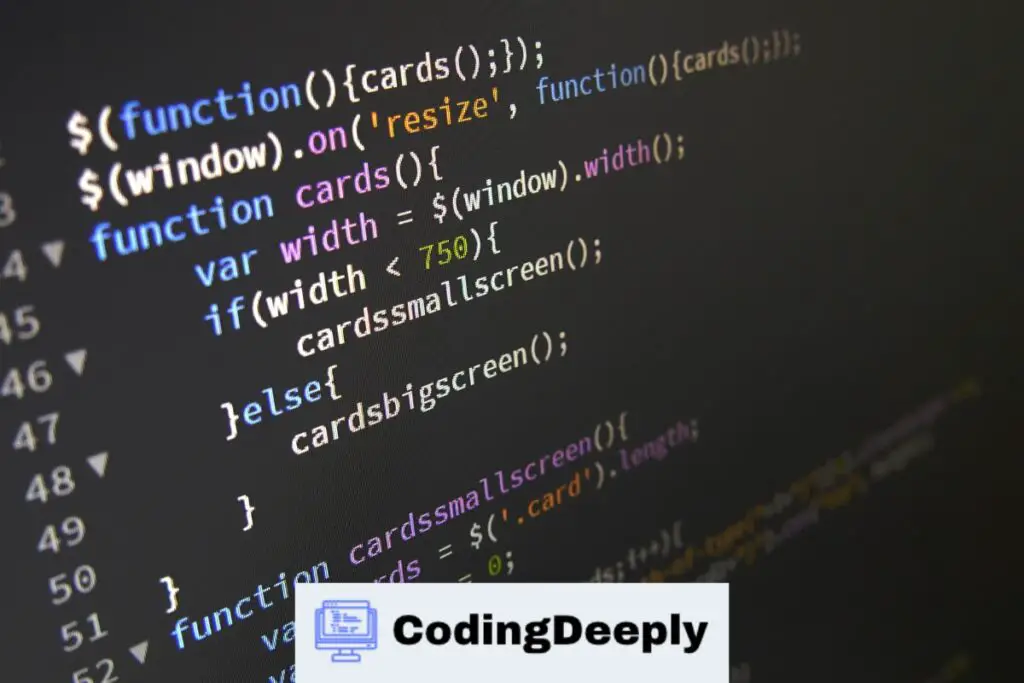
- The Shebang line in Python scripts specifies the interpreter for running the script.
- #!/usr/bin/env python ensures the script runs with the Python interpreter in the environment.
- Shebang ensures cross-platform compatibility for Python scripts.
- Specifying the Python version in the Shebang line can influence script execution.
- The Shebang line plays a crucial role in setting script execution permissions.
Understanding the Shebang Line in Python Scripts
Ever stumbled upon a line in Python scripts that starts with a #! and wondered what it’s all about? Well, you’re not alone. This line is known as the Shebang line and is more important than you might think.
What is a Shebang Line?
The Shebang line is the first line in a script that tells the system which interpreter to use for running the script. It’s like a roadmap for your script, guiding it to the right interpreter.
Why is it Used in Python Scripts?
The Shebang line is used in Python scripts to ensure that the script runs using the Python interpreter, regardless of the environment where the script is executed. This means that whether you’re running your script on your local machine, a server, or a different operating system, the Shebang line ensures your script will be interpreted correctly.
Here is a practical example:
With Shebang line:
Let’s say you have a Python script named hello.py
:
#!/usr/bin/env python3 print("Hello, World!")
You can make the script executable using the command chmod +x hello.py
and then run it directly from the command line with ./hello.py
.
The output will be Hello, World!
.
Without Shebang line:
Now, let’s remove the Shebang line from the script:
print("Hello, World!")
If you try to run the script directly from the command line with ./hello.py
, you’ll get an error because the system doesn’t know which interpreter to use. Instead, you’ll have to specify the Python interpreter explicitly when running the script: python3 hello.py
.
The output will still be Hello, World!
.
This example illustrates how the Shebang line makes it easier to run Python scripts directly from the command line, without having to specify the Python interpreter each time.
The Role of #!/usr/bin/env in Python
Now that we’ve covered the basics of the Shebang line let’s dive deeper into one of its most common forms in Python scripts: #!/usr/bin/env python.
What Does #!/usr/bin/env Do?
The #!/usr/bin/env python line is a universal way to run Python scripts. It tells the system to use the Python interpreter located in the environment where the script is running.
This is particularly useful when you’re working in environments where the location of the Python interpreter might vary.
How Does It Affect the Execution of Python Scripts?
The #!/usr/bin/env python line ensures your Python script runs smoothly across different environments. It allows you to run the script directly from the command line without specifying the Python interpreter each time.
This saves you time and makes your script more portable and easier to use.
The Importance of Shebang in Cross-Platform Compatibility
One of the biggest advantages of using the Shebang line in Python scripts is its role in ensuring cross-platform compatibility.
How Does Shebang Ensure Cross-Platform Compatibility?
The Shebang line allows your Python script to run on any system that has Python installed, regardless of the specific path to the Python executable.
This means that whether you’re running your script on a Windows, Linux, or MacOS system, the Shebang line ensures your script will execute correctly.
Examples of Different Operating Systems and How Shebang Works in Each
Let’s take a look at how the Shebang line works in different operating systems:
- Windows: Windows doesn’t natively recognize the Shebang line, but tools like Cygwin or the Windows Subsystem for Linux can interpret it.
- Linux: Linux recognizes the Shebang line and uses it to determine the correct interpreter for the script.
- MacOS: Like Linux, MacOS also recognizes the Shebang line and uses it to determine the correct interpreter.
In each case, the Shebang line ensures that your Python script runs correctly, regardless of the operating system.
So, the next time you see #!/usr/bin/env python at the top of a Python script, you’ll know exactly what it’s doing and why it’s so important.
Running Scripts with Different Versions of Python
Python has evolved over the years, with different versions offering various features. But how does this affect our scripts? Let’s dive in.
How to Specify the Python Version in the Shebang Line
The Shebang line can be tailored to specify which version of Python to use. For instance, if you want your script to run with Python 3, you can use #!/usr/bin/env python3 as your Shebang line.
This ensures that your script will run using Python 3, even if Python 2 is your environment’s default interpreter.
The Impact of Different Python Versions on Script Execution
Different versions of Python can interpret scripts differently. For instance, Python 2 and 3 have different syntax rules and library functions.
By specifying the Python version in the Shebang line, you can ensure that your script runs as intended, regardless of the default Python version in the environment.
Setting Script Execution Permissions with Shebang
The Shebang line isn’t just about specifying the interpreter. It also plays a role in setting script execution permissions.
How to Set Execution Permissions for a Python Script Using Shebang
To make a Python script executable, include a Shebang line and then set the script’s permissions.
This can be done using the chmod command in Unix-based systems. Once the script is executable, you can run it directly from the command line without explicitly calling the Python interpreter.
Practical Examples of Setting Permissions
Here’s a quick example:
- Include a Shebang line in your script: #!/usr/bin/env python3
- Set the script’s permissions: chmod +x myscript.py
- Run the script: ./myscript.py
#!python vs #!/usr/bin/env
Finally, compare two common Shebang lines: #!python and #!/usr/bin/env python.
The Difference Between #!python and #!/usr/bin/env python
The #!python line tells the system to use the Python interpreter located in the system’s default path. On the other hand, #!/usr/bin/env python tells the system to use the Python interpreter located in the environment where the script is running.
This makes #!/usr/bin/env python more flexible and portable across different environments.
When to Use Each One
If you’re writing a script that will only be run in a specific environment, #!python might be sufficient. However, if you want your script to be portable and run across different environments, #!/usr/bin/env python is the way to go.
Frequently Asked Questions
Can I use the Shebang line with other programming languages besides Python?
Absolutely! The Shebang line isn’t exclusive to Python. It’s used in scripts for many other programming languages like Perl, Ruby, and Shell. The key is to specify the correct interpreter for your language. For instance, you might use #!/usr/bin/env ruby for a Ruby script.
What happens if I don’t include a Shebang line in my Python script?
If you don’t include a Shebang line, you must specify the Python interpreter each time you run the script from the command line. For example, you’d run python myscript.py instead of just ./myscript.py. Without a Shebang line, the script isn’t as portable and can’t be run directly as an executable.
Is there a difference between #!/usr/bin/python and #!/usr/bin/env python?
Yes, there is. #!/usr/bin/python specifies the exact path to the Python interpreter. This assumes that Python is located at /usr/bin/python, which might only be the case in some environments. On the other hand, #!/usr/bin/env python uses the environment’s path to find the Python interpreter, making it more flexible and portable.
Can I specify a specific version of Python with #!/usr/bin/env?
Yes, you can specify a version of Python with #!/usr/bin/env by appending the version number after python. For example, #!/usr/bin/env python3 would specify Python 3 as the interpreter for the script.
What if I have multiple versions of Python installed? Which one does #!/usr/bin/env python use?
The #!/usr/bin/env python line uses the first Python interpreter found in your environment’s path. If you have multiple versions of Python installed, it will use the one that appears first in the path. You can check which Python interpreter is used by default with the python command.
Conclusion
Understanding the Shebang line and the role of #!/usr/bin/env in Python scripts is crucial for writing portable and flexible scripts. It ensures your scripts run correctly across different Python versions and operating systems.
So, remember the Shebang line the next time you’re writing a Python script!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.