Are you struggling with how to skip lines in a Python file? Do you need to skip certain lines based on specific conditions? This article will provide you with the knowledge and techniques to effectively handle file skipping in Python.
Skipping lines in a file is a common requirement for various Python projects, such as data processing, text manipulation, and log analysis.
Your code may run into errors or inefficiencies without the ability to skip lines.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding File Handling in Python
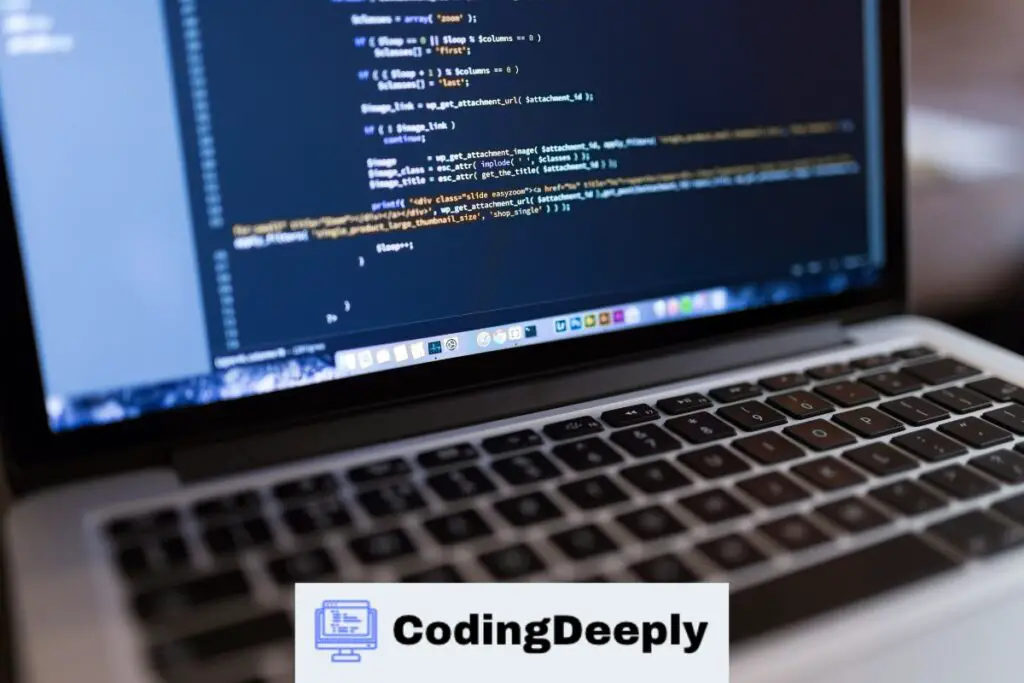
File handling is an essential aspect of any programming language, including Python. It involves reading and writing data to files and performing various operations.
In Python, file handling is accomplished by using built-in functions and modules.
The Basics of File Handling
The first step in file handling is opening the file.
This is done using the `open()` function, which takes two arguments: the file’s name and the mode to open it. Modes can be `r` for reading, `w` for writing, `a` for appending, `x` for creating a new file, or `b` for binary mode.
For example, to open a file named “example.txt” in read mode, the following code snippet is used:
file = open("example.txt", "r")
Once the file is opened, the `read()` function can be used to read its contents. The `read()` function returns the entire content of the file as a string. Alternatively, the `readline()` function can read one line at a time.
After reading or writing to a file, it is essential to close it using the `close()` function to free up system resources.
Examples of File Operations
Let’s consider some examples of file operations in Python:
- Reading a File: To read a file named “example.txt” line by line, the code looks like this:
-
file = open("example.txt", "r")
for line in file:
print(line)
file.close()
- Writing to a File: To write to a file named “example.txt” and add some text to it, the code looks like this:
-
file = open("example.txt", "w")
file.write("This is some text.")
file.close()
- Appending to a File: To append text to a file named “example.txt”, the code looks like this:
-
file = open("example.txt", "a")
file.write("This is some new text.")
file.close()
Reading and Skipping Lines in a Python File
When working with files in Python, reading and skipping specific lines is often necessary. This can be useful, for example, when you want to ignore header or comment lines within a file.
This section will explore different methods for reading and skipping lines in a Python file.
Using a Loop to Read and Skip Lines
A loop is a common method to read and skip lines in a Python file.
You can use the built-in open() function to open a file and then iterate over each line in the file using a for loop. You can use conditional statements within the loop to determine which lines to skip.
For example:
with open('example.txt') as file: for line in file: if line.startswith('#'): continue # do something with line
In this example, we open a file named example.txt
and iterate over each line in the file using a for loop.
We use an if
statement to check if the line starts with a ‘#’ character, which is the common way to indicate a comment line in many programming languages. If the line is a comment, we use the continue
statement to skip the line and proceed to the next line in the file.
If the line is not a comment, we can perform some operation on it, such as printing it to the console or writing it to a new file.
Using the ‘next()’ Function to Skip Lines
Another method to skip lines in a Python file is to use the built-in next()
function. The next()
function returns the next item from an iterator, which in this case is the file object returned by the open()
function.
You can use the next()
function to skip a specific number of lines in a file.
For example:
with open('example.txt') as file: # skip first two lines next(file) next(file) # do something with next line line = next(file) # do something with line
In this example, we open a file named example.txt
and use the next()
function to skip the first two lines of the file.
We then assign the next line to the variable line
and perform some operation on it, such as printing it to the console or writing it to a new file.
Using the next()
function can be useful when you only need to skip a specific number of lines in a file, allowing you to skip lines quickly and easily.
Skipping Lines Based on Conditions
Skipping lines in a Python file based on certain conditions can be a powerful way to extract specific data or remove unwanted information.
This section will cover different approaches for skipping lines in Python files based on conditions.
Using Conditional Statements
One common approach for skipping lines based on conditions is to use conditional statements.
For example, if you want to skip all lines that include the word “error,” you could use the following code:
with open("file.txt", "r") as f:
for line in f:
if "error" in line:
continue
print(line)
In the example above, the “continue” keyword is used to skip lines that include the word “error”.
The rest of the lines are printed to the output.
Using Regular Expressions
Another approach for skipping lines based on conditions involves using regular expressions. Regular expressions are patterns that match strings of text.
They can search for specific patterns or words within a string.
For instance, if we want to skip all the lines that start with a “#” character, we can use regular expressions along with the re module:
import re
with open("file.txt", "r") as f:
for line in f:
if re.match("^#", line):
continue
print(line)
In the code above, we use the `re.match()` function to check if the line starts with a “#” character. If so, we skip the line using “continue”.
Otherwise, the line is printed to the output.
Using regular expressions can be an effective way to skip lines based on complex patterns or conditions.
Handling Errors and Exceptions
While skipping lines in a Python file, errors and exceptions may occur. It is important to handle them efficiently to avoid program crashes and data loss.
Try-Except Statement
The try-except statement is used in Python to handle errors and exceptions. It works by attempting to execute a given block of code and, if an error occurs, it catches the exception and executes a specified block of code instead of crashing the program.
When skipping lines in a file, a try-except statement can be used to handle possible errors. For example:
try: file = open("example.txt", "r") for line in file: if line.startswith("Skip"): continue print(line) except IOError: print("Error: File not found or could not be opened")
In this example, an IOError exception is raised if the specified file cannot be opened or does not exist. The try-except statement catches this exception and prints an error message instead of crashing the program.
Graceful Error Handling
When handling errors and exceptions during file skipping, providing clear and concise error messages to the user is important. This helps identify the cause of the error and provides a possible solution.
Additionally, it is recommended to log errors to a file or database for future debugging and troubleshooting. This allows for easier identification and resolution of errors during large or complex file-skipping operations.
Using proper error-handling techniques, file skipping in Python can be made more efficient and reliable.
Efficiently Skipping Large Files
Skipping lines in a large file can take considerable time, so optimizing the process for better performance is essential. Here are some strategies for efficiently skipping lines in large files:
Utilize Memory Mapping
Memory mapping is a technique used to access large files by mapping the file into virtual memory. This allows the file to be accessed as if it were in RAM, providing faster access speeds.
To use memory mapping, you can utilize the mmap module in Python. Using memory mapping, you can skip lines in a file without reading the entire file into memory.
Use the Linecache Module
The linecache module in Python provides a caching mechanism for file lines, allowing you to access specific lines in a file without reading the entire file. This technique is useful when working with large files by allowing you to skip lines in a file without loading the entire file into memory.
Implement Parallel Processing
When working with large files, parallel processing can help to increase performance by distributing the workload across multiple processors or cores.
This technique can be applied to file skipping by dividing the file into smaller sections and assigning each section to a different processor for processing.
By using these strategies, you can efficiently skip lines in large files and improve the performance of your file-skipping workflows. It’s important to identify the most suitable technique for your specific use case and ensure that your code is designed for optimization.
Best Practices for File Skipping in Python
File skipping in Python can be a powerful tool, but it must be used carefully.
Here are some best practices to help you effectively and efficiently skip lines in your Python files:
Organize Your Code
As with any coding project, organizing your code can make a big difference in readability and maintainability. Use descriptive variable names, comment your code, and break up long code blocks into smaller, more manageable pieces.
Consider Performance Optimization
If you are working with very large files or complex operations, consider using tools such as memory mapping or parallel processing to improve performance.
However, be cautious and test your code thoroughly to avoid unintended consequences.
Handle Errors Gracefully
Before skipping lines in a Python file, it is important to consider and handle any potential errors or exceptions that may occur. Use the `try-except` statement to handle any issues that may arise during file skipping gracefully.
Avoid Hardcoding
Avoid hardcoding values or paths in your code, leading to errors and making your code less flexible. Instead, use variables or command-line arguments for greater flexibility and easier maintenance.
Test Your Code Thoroughly
Before deploying your code, test it thoroughly to ensure it functions as intended. Test your code with various input files and scenarios to catch any potential errors or bugs before they become a problem in production.
- Tip: Consider using assert statements to test your code. This can help catch errors early and ensure your code works as intended.
By following these best practices, you can ensure that your file-skipping workflows in Python are more effective, efficient, and maintainable.
Frequently Asked Questions (FAQ)
You may encounter some questions and concerns as you delve deeper into file skipping in Python.
Here are some of the most frequently asked questions:
What is the difference between file.read() and file.readline()?
file.read()
reads the entire file and returns its contents as a string, while file.readline()
reads a single line from the file and returns it as a string. If you want to read a specific number of characters or bytes, you can pass an argument to file.read()
.
Can I skip a certain number of lines instead of skipping lines based on conditions?
Yes, you can skip a certain number of lines by using a for loop and calling the next()
function a specific number of times.
For example, to skip the first two lines of a file, you can use:
with open('file.txt', 'r') as file: for i in range(2): next(file) for line in file: # do something with the remaining lines
What is the best way to handle errors when skipping lines in a file?
Using a statement is the best way to handle errors when skipping lines in a file. This allows you to catch any errors and perform appropriate actions, such as displaying an error message or skipping to the next line.
How can I improve the performance of skipping lines in large files?
You can use several techniques to improve the performance of skipping lines in large files.
One approach is to use the linecache
module, which caches lines from a file in memory to reduce disk access. Another technique is to utilize memory mapping, which allows you to access the file directly from memory.
Additionally, implementing parallel processing using libraries such as multiprocessing
or concurrent.futures
can significantly improve performance.
Are there any best practices for file skipping in Python?
Yes, there are several best practices for file skipping in Python.
First, it is important to organize your code logically and readably. Using descriptive variable names and commenting your code can also improve readability.
Further, handling errors gracefully and optimizing performance using techniques like the ones mentioned above is important.
By following these best practices, you can ensure that your file-skipping workflows are efficient, reliable, and maintainable.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.