When working with files in Python, it’s common to have a header line at the beginning of the file that contains information about the data in the file.
Nonetheless, this header line is often unnecessary when processing data and can cause issues if included. In this section, we’ll explore how to skip the first line when reading a file in Python to avoid including the header in your data processing.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
File handling in Python
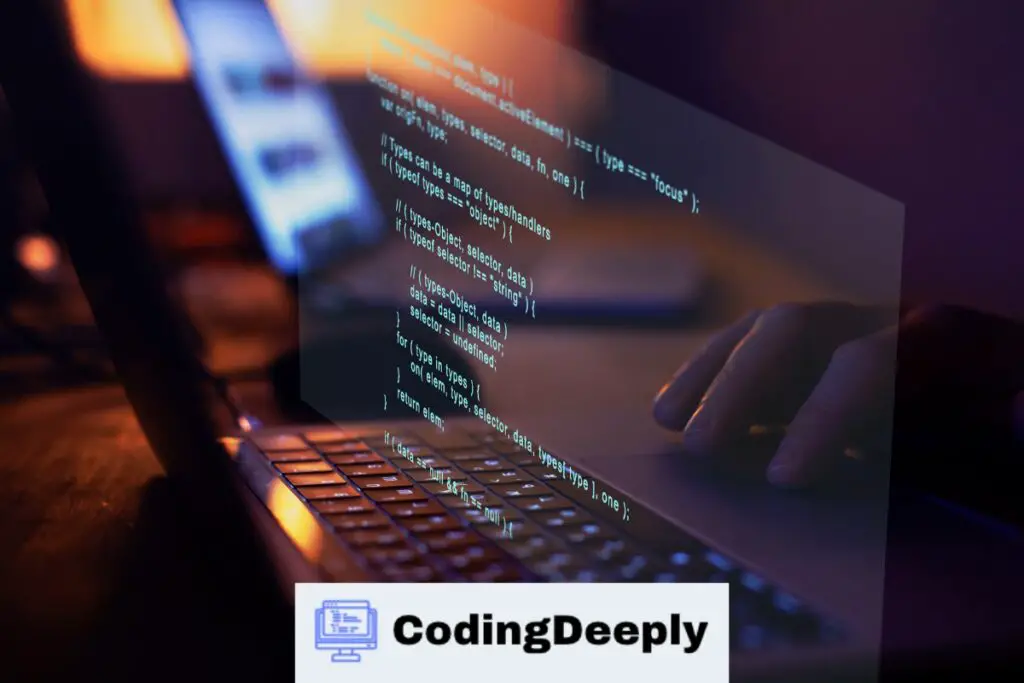
Before we dive into skipping the first line, let’s quickly review the basics of file handling in Python. File handling is an essential aspect of programming that enables you to read, write, and manipulate files on your computer.
Python provides built-in functions and methods to work with files, making it a powerful language for handling data.
Opening a file
To open a file in Python, you need to use the built-in open() function. The open() function takes two arguments: the filename and the mode you want to open the file. The filename is the path to the file you want to open, and the mode specifies whether you want to read, write, or append to the file.
The most common modes are:
- ‘r’: Read mode. It opens the file for reading (default mode).
- ‘w’: Write mode. It opens the file for writing. If the file exists, it truncates the file. If the file doesn’t exist, it creates a new file.
- ‘a’: Append mode. It opens the file for writing. If the file exists, it appends the data to the end of the file. If the file doesn’t exist, it creates a new file.
Here is an example of opening a file in read mode:
f = open('example.txt', 'r')
This code opens the file named ‘example.txt’ in read mode and returns a file object (f) you can use to read the file’s contents.
Reading the contents of a file
Once you have opened a file, you can read its contents using various methods. The most common method is the read() method, which reads the file’s entire contents at once.
Here is an example of reading the entire contents of a file:
contents = f.read()
This code reads the entire contents of the file object f and stores them in the variable contents.
You can also read the contents of a file line by line using the readline() method. The readline() method reads a single line from the file and moves the file pointer to the next line.
Here is an example of reading a file line by line:
line = f.readline()
This code reads a single line from the file object f and stores it in the variable line. You can use a loop to read all the lines in the file.
The next section’ll discuss how to read a file line by line using a loop.
Reading a file line by line
Now that we know the basics of file handling, we can move on to reading a file line by line. This is necessary when we want to skip the first line of a file.
To read a file line by line in Python, we use a loop to iterate over the lines of the file. Here’s an example:
with open('example.txt') as file: for line in file: print(line)
This code snippet opens the file ‘example.txt’ and iterates over each line of the file, printing each line to the console.
Notice how we don’t need to specify the number of lines to read or the starting position. The loop automatically reads and processes each line until there are no more lines to read.
Skipping the first line
Now that we know how to read a file line by line, we can implement the logic to skip the first line. The simplest approach is to use a boolean variable to track whether we have read the first line.
We can then continue reading and processing the remaining lines as needed.
Here’s an example:
with open('file.txt', 'r') as f: first_line = True for line in f: if first_line: first_line = False continue # skip the first line # process and manipulate data as needed
Inside the loop, we check if the boolean first_line is True.
If it is, we set it to False and skip the first line using the continue keyword. All subsequent lines in the file will be processed and manipulated as needed.
Alternative approach:
Another approach is to use the next() method to skip the first line. The next() method returns the next item from an iterator. In the case of an open file, it returns the first line. We can call next() once before iterating over the remaining lines of the file.
Here’s an example:
with open('file.txt', 'r') as f: next(f) # skip the first line for line in f: # process and manipulate data as needed
This approach is more concise but may be less intuitive for novice programmers. It also raises a StopIteration exception if called on an empty file. Therefore, it’s important to wrap the call to next() in a try-except block to handle this exception.
Handling exceptions and edge cases
While skipping the header line is a simple task, it’s essential to consider exceptions and edge cases that may arise.
What happens when the file is empty?
If the file is empty, attempting to read it will result in an error. As a result, it’s essential to account for this possibility and handle it appropriately. The following code snippet demonstrates one way to handle an empty file:
# Open file
try:
with open(‘file.txt’, ‘r’) as file:
first_line = file.readline()
if not first_line:
print(“File is empty!”)
# Continue processing the rest of the file
except FileNotFoundError:
print(“File not found!”)
What happens when the file only has one line?
If the file only has one line – which happens to be the header – then the header line should be skipped. One way to handle this is to use a try-except block to read the second line of the file instead of the header line:
# Open file
try:
with open(‘file.txt’, ‘r’) as file:
try:
header = file.readline()
second_line = file.readline()
except IndexError:
print(“File has only one line!”)
# Continue processing the rest of the file
except FileNotFoundError:
print(“File not found!”)
By considering these edge cases, you can ensure that your program can handle any situation while processing files.
Efficient data processing without the header
Now that we know how to skip the first line of a file, we can efficiently process the data without the header. Here are some data manipulation techniques that can help:
Sorting
If you need to sort the data in a file, you can use the sorted()
function. For example, let’s say you have a file with numbers separated by commas:
10, 5, 7, 3, 9 22, 11, 19, 13, 25
You can sort the numbers in ascending order like this:
with open('data.txt') as f: next(f) # skip header for line in f: numbers = [int(n) for n in line.split(',')] sorted_numbers = sorted(numbers) print(sorted_numbers)
This will output:
[3, 5, 7, 9, 10] [11, 13, 19, 22, 25]
Aggregation
You can also perform aggregation functions on the data, such as calculating the average or sum. Let’s say you have a file with sales data:
Product, Sales A, 100 B, 150 C, 75 A, 50 B, 200
You can calculate the total sales for each product like this:
sales = {} with open('data.txt') as f: next(f) # skip header for line in f: product, amount = line.split(',') amount = int(amount.strip()) if product in sales: sales[product] += amount else: sales[product] = amount for product, total_sales in sales.items(): print(f'Total sales for {product}: ${total_sales}')
This will output:
Total sales for A: $150 Total sales for B: $350 Total sales for C: $75
Filtering
You can also filter the data based on certain criteria. Let’s say you have a file with customer information:
Name, Age John, 25 Jane, 30 Bob, 20 Alice, 35
You can filter the customers who are above a certain age like this:
with open('data.txt') as f: next(f) # skip header for line in f: name, age = line.split(',') age = int(age.strip()) if age > 25: print(name)
This will output:
Jane Alice
These are just a few examples of efficiently processing data without the header line.
The possibilities are endless!
FAQ – Frequently Asked Questions
Now that we’ve covered the basics of skipping the first line when reading a file in Python, let’s address some common questions and concerns.
Q: What happens if the file is empty?
If the file is empty, reading the first line will throw an error. To avoid this, check if the file is empty before reading the first line.
Q: What if the file only has one line?
If the file only has one line and it is the header, skipping the first line will result in an empty file. You may consider including the header or adjusting your data processing logic in this case.
Q: Can I skip a different line besides the first one?
You can modify the logic demonstrated in this article to skip any line you want by changing the line number in the loop.
Q: How can I include the header in my data processing?
If you want to include the header in your data processing, remove the code that skips the first line.
Q: Is it possible to skip multiple lines?
Yes, you can modify the loop to skip multiple lines. Simply adjust the range in the loop to start from the desired line number.
Q: Can I skip lines based on a condition?
Yes, you can use a conditional statement inside the loop to skip lines that meet certain criteria. For example, you can skip lines that contain a specific value or pattern.
Q: Why is it important to consider edge cases?
By considering edge cases, you can ensure that your code can handle unexpected scenarios and prevent errors from occurring. This is especially important when working with real-world data with variations and inconsistencies.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.