If you’re working with Python lists, you may have encountered the issue of having quotes around the elements of your list.
This can hinder data manipulation and make it difficult to perform calculations or comparisons.
But don’t worry. We have an easy and efficient method to remove quotes from your Python list.
In this section, we’ll discuss the problem of quotes in Python lists and provide a step-by-step guide to removing them. Our method is straightforward and requires only a few lines of code.
So, let’s get started and make your data manipulation cleaner and more efficient!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Quotes in Python Lists
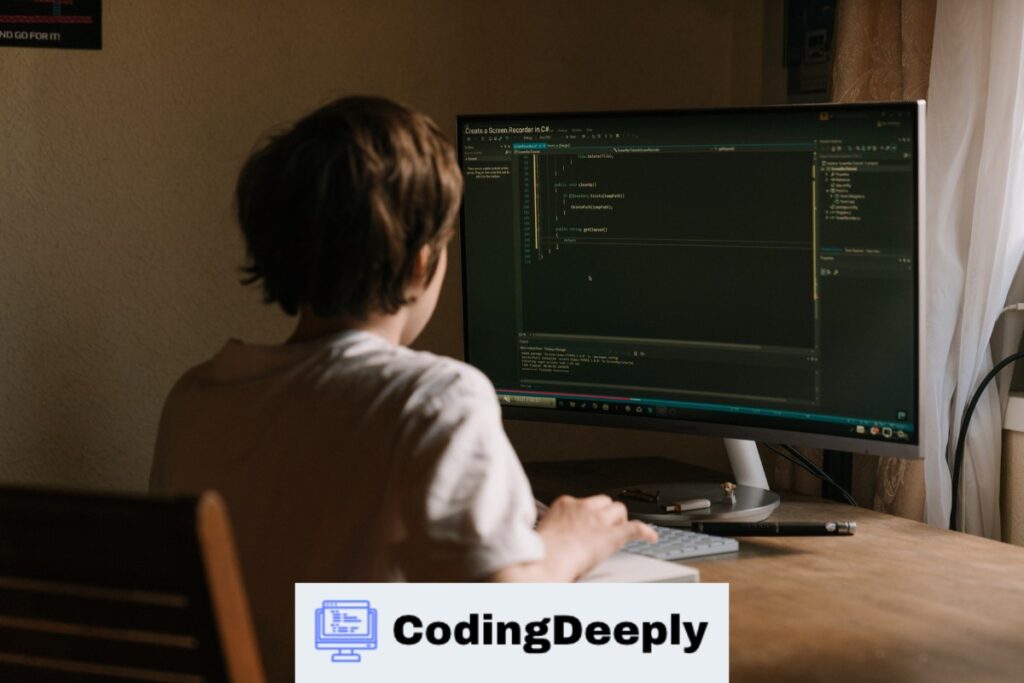
Before we dive into removing quotes, it is important to understand how quotes are represented in Python lists. Anything enclosed within single or double quotes is considered a string in Python.
Therefore, each string will be enclosed within quotes when we have a list containing strings.
For example:
my_list = ['apple', 'banana', 'orange']
my_list = ["John", "Doe", "30"]
In the first example, the list contains three strings, ‘apple’, ‘banana’, and ‘orange’, each enclosed within single quotes.
In the second example, the list contains three strings, “John”, “Doe”, and “30”, each enclosed within double quotes.
It is important to note that when we print a list, Python automatically adds the quotes to each string.
Nevertheless, we don’t see the quotes when we access a specific string within the list.
For example:
print(my_list)
outputs['apple', 'banana', 'orange']
print(my_list[0])
outputsapple
Keep this in mind when manipulating Python lists that contain strings.
The Method to Remove Quotes from a Python List
Now that we clearly understand quotes in Python lists, let’s explore the easy and efficient method to remove them. The method involves using a list comprehension to iterate through the list and remove the quotes from each string element.
The syntax of the method
The syntax for the method is as follows:
new_list = [element.replace(‘”‘, ”) for element in old_list]
The above syntax uses a list comprehension to iterate through the old_list and remove the quotes from each element using the replace() method.
The resulting list, new_list, contains all the elements of old_list with quotes removed.
Example code:
Here’s an example code that demonstrates the method:
old_list = ['"apple"', "'banana'", '"cherry"', "'date'"] new_list = [element.replace('"', '') for element in old_list] print(new_list)
The output of the above code will be:
['apple', 'banana', 'cherry', 'date']
In this example, the old_list contains four elements, two enclosed within double quotes and two within single quotes. The list comprehension removes all the quotes, creating a new_list with clean and tidy data for efficient Python list manipulation operations.
Benefits of Removing Quotes from Python Lists
Removing quotes from a Python list offers several benefits. One of the primary benefits is achieving clean and tidy data manipulation, especially when dealing with numerical calculations or comparisons.
When quotes are present in a list, Python treats them as strings, which can lead to errors when performing mathematical operations or comparisons.
Removing quotes from a list makes the data cleaner and easier to manipulate. Working with large datasets or complex calculations can save time and effort.
Another advantage of removing quotes from a Python list is improved efficiency. When quotes are present in a list, Python needs to perform additional string operations, which can slow down the code and increase processing time.
The code becomes more efficient and runs faster by eliminating unnecessary string operations.
For example, imagine you have a list of numbers represented as strings, like this:
numbers = ['10', '20', '30']
If you want to add these numbers together, you need first to convert them to integers:
total = 0 for num in numbers: total += int(num)
However, if the quotes were removed from the list, you could skip this conversion step and perform the addition directly:
numbers = [10, 20, 30] total = sum(numbers)
Removing quotes from a Python list can lead to cleaner, more efficient code and improved data manipulation capabilities.
FAQ – Frequently Asked Questions
Here are some frequently asked questions related to removing quotes from a Python list:
Q: Why do I need to remove quotes from a Python list?
A: Removing quotes from a Python list can help achieve clean and tidy data manipulation, especially when dealing with numerical calculations or comparisons.
It also improves the efficiency of Python list manipulation operations by eliminating unnecessary string operations.
Q: How do I know if my Python list contains quotes?
A: If your Python list contains strings, each string will be enclosed within quotes. You can check for the presence of quotes by using the type() function to determine the data type of each element in your list.
Q: Will removing quotes from my Python list affect the integrity of my data?
A: Removing quotes from a Python list only affects the appearance of the data, not its content. The actual data values remain the same.
Q: Can I remove quotes from multi-dimensional Python lists?
A: Yes, the method to remove quotes from a multi-dimensional Python list is the same as for a one-dimensional list. Simply apply the method to each element of the list.
Q: What if I accidentally remove quotes from a non-string element in my Python list?
A: If you accidentally remove quotes from a non-string element, it will not affect the functionality of your code. However, it is important to be mindful of the data types of your elements before performing any operations on them.
Q: Are there any risks associated with removing quotes from Python lists?
A: No inherent risks are associated with removing quotes from Python lists. However, ensuring that your code is error-free and thoroughly tested before deploying it in a production environment is important.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.