Working with lists in Python is an essential part of data handling, and sometimes removing commas from a list becomes necessary. Clean data handling is crucial for accurate results, and mastering this technique can help streamline data manipulation tasks.
This guide will provide easy-to-follow steps for removing commas from a list in Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Lists and Commas in Python
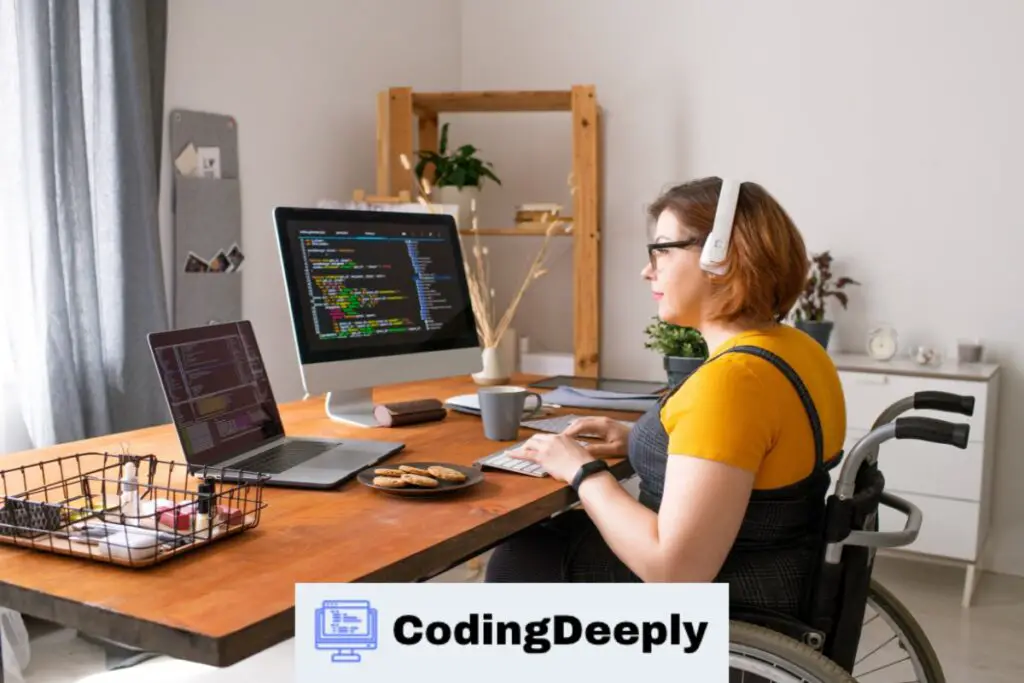
In Python, lists are a type of data structure that can contain multiple elements, including integers, strings, and other types of objects. Commas are used to separate these elements within the list, allowing for easy manipulation and organization of data.
For example, a list of integers might look like this:
[1, 2, 3, 4, 5]
while a list of strings might look like this:
['apple', 'banana', 'orange', 'grape']
Using commas as separators makes it easy to perform operations on individual elements within the list and iterate over the entire list as a single entity.
However, there are times when it is necessary to remove commas from a list in Python. This may be required for certain data manipulation tasks or to ensure the data is properly formatted for other applications.
Removing commas from a list can be a simple process, but it requires understanding how lists work and how they are structured.
In the following sections, we will explore different techniques for removing commas from lists in Python, including built-in functions and methods, as well as more advanced approaches for handling nested lists and other complex data structures.
Using the .replace() Method to Remove Commas
If you have a list of elements separated by commas that you want to remove in Python, the easiest way to accomplish this task is by using the .replace() method.
This method replaces a specified substring with another string and returns a new string. In our case, we will replace commas with an empty string to remove them from the list.
Here is an example:
- Create a list with commas:
my_list = ['apple', 'banana', 'pear', 'orange, peach'] # Note the comma after orange
- Use a for loop to iterate through each element of the list:
for i in range(len(my_list)): my_list[i] = my_list[i].replace(",", "")
- Print the updated list:
print(my_list)
The output will be:
['apple', 'banana', 'pear', 'orange peach']
Note that the comma has been removed from the last element of the list.
It is also possible to use list comprehension to achieve the same result:
my_list = ['apple', 'banana', 'pear', 'orange, peach'] my_list = [item.replace(",", "") for item in my_list] print(my_list)
The output will be the same as before:
['apple', 'banana', 'pear', 'orange peach']
The .replace() method is a straightforward and efficient way to remove commas from a list in Python. It is particularly useful when dealing with large datasets where cleaning the data before performing any analyses is necessary.
Removing Commas from a List of Strings
If a list contains strings that include commas, it can be challenging to remove them without altering the original string’s meaning. Below are some techniques to remove commas from strings in a list.
Using List Comprehension
One way to remove commas from a list of strings is to use list comprehension. This technique involves iterating over the list and applying the replace()
method to each string to remove the commas. Here’s an example:
string_list = ['John,Smith', 'Jane,Doe', 'Bob,Baker'] no_commas_list = [string.replace(',', '') for string in string_list]
This code creates a new list called no_commas_list
, which contains the same strings as string_list
but with the commas removed.
Using the join() Method
Another technique to remove commas from a list of strings is to use the join()
method. This technique involves joining the strings in the list into one long string, removing the commas, and then splitting the string back into separate strings in a list.
Here’s an example:
string_list = ['John,Smith', 'Jane,Doe', 'Bob,Baker'] no_commas_list = ["".join(string.split(',')) for string in string_list]
This code creates a new list called no_commas_list
, which contains the same strings as string_list
but with the commas removed.
Using the map() Function
The map()
function can also be used to remove commas from a list of strings. This function applies a function to each element in a list and returns a new list with the function’s output.
Here’s an example:
string_list = ['John,Smith', 'Jane,Doe', 'Bob,Baker'] no_commas_list = list(map(lambda x: x.replace(',', ''), string_list))
This code creates a new list called no_commas_list
, which contains the same strings as string_list
but with the commas removed.
Handling Nested Lists with Commas
Removing commas from nested lists in Python can be a little trickier than removing them from flat lists.
A nested list refers to a list that contains one or more sub-lists. These sub-lists can also contain other sub-lists, and so on, creating a nested structure.
This section’ll discuss some strategies for handling nested lists with commas.
Recursion
A recursive function is one way to remove commas from a nested list. A recursive function is a function that calls itself until a specific condition is met, in this case, until all commas have been removed from the nested list.
Here’s an example:
<img src="https://seowriting.ai/32_6.png" alt="python remove comma from list item"> def remove_commas(nested_list): new_list = [] for item in nested_list: if isinstance(item, list): new_list.append(remove_commas(item)) else: new_list.append(item.replace(',', '')) return new_list nested_list = [[1, 2, 3], [4, 5, 6,], [7, [8, 9, 10]], 11, [12, 13, [14, 15, 16]], 17] new_list = remove_commas(nested_list) print(new_list)
In the example above, the remove_commas() function takes a nested list as input and returns a new list with all commas removed. The function checks if each item in the list is a list itself.
If it is, the function is called again, and the item is passed as input. This process continues until all commas have been removed from each item in the nested list.
Iteration
Another way to remove commas from a nested list is to use iteration. Iteration refers to the process of accessing each item in a list individually. Here’s an example:
<img src="https://seowriting.ai/32_6.png" alt="python remove comma from list item"> def remove_commas(nested_list): for i in range(len(nested_list)): if isinstance(nested_list[i], list): remove_commas(nested_list[i]) else: nested_list[i] = nested_list[i].replace(',', '') nested_list = [[1, 2, 3], [4, 5, 6,], [7, [8, 9, 10]], 11, [12, 13, [14, 15, 16]], 17] remove_commas(nested_list) print(nested_list)
In the above example, the remove_commas() function modifies the nested_list directly by iterating through each item in the list. If the item is a list itself, the function is called again, and the item is passed as input.
This process continues until all commas have been removed from each item in the nested list.
Other Methods for Removing Commas
While the .replace() method is a commonly used for removing commas from a list in Python, some other built-in functions and libraries can achieve the same result.
The .split() Method
The .split() method in Python allows you to split a string into a list of substrings based on a specified delimiter. Using a comma as the delimiter, you can split each string in the list into separate elements without commas.
Here’s an example:
my_list = ['apple, banana, orange', 'grape, mango, pineapple'] new_list = [elem.split(', ') for elem in my_list] print(new_list)
This code will output:
[['apple', 'banana', 'orange'], ['grape', 'mango', 'pineapple']]
The .split() method is particularly useful for lists containing strings with commas.
Regular Expressions
Regular expressions are a powerful tool for pattern matching in Python. You can use regular expressions to find and replace commas within list elements. Here’s an example:
import re my_list = ['apple, banana', 'orange, grape'] new_list = [re.sub(r',', '', elem) for elem in my_list] print(new_list)
This code will output:
['apple banana', 'orange grape']
The re.sub() function replaces all occurrences of a comma (,) with an empty string (”).
NumPy
NumPy is a Python library that supports large, multi-dimensional arrays and matrices. It offers a variety of functions for performing operations on arrays, including removing commas from lists. Here’s an example:
import numpy as np my_list = ['apple, banana', 'orange, grape'] new_list = np.array(my_list) new_list = [elem.replace(',', '') for elem in new_list] print(new_list)
This code will output:
['apple banana', 'orange grape']
You can use the .replace() method to remove commas from each element by converting the list into a NumPy array.
Best Practices for Clean Data Handling
Removing commas from lists in Python can improve the accuracy and reliability of your data. However, it’s important to approach this task with clean data handling practices in mind.
Here are some tips to ensure your data remains accurate and reliable throughout the process:
Validate Your Data
It’s crucial to validate the data before attempting to remove commas from a list. Ensure that the data is formatted correctly and that there are no errors or inconsistencies.
Data validation can help prevent errors and ensure your results are accurate and reliable.
Handle Errors Effectively
Even with proper data validation, errors are bound to occur. When they do, it’s important to handle them effectively. Be sure to include error-handling code in your script to catch and handle any errors that may arise.
Optimize Your Code
When removing commas from a list in Python, optimizing your code for efficiency is important.
This includes using built-in functions and libraries when appropriate, minimizing the number of loops, and minimizing redundant code.
Document Your Code
Documenting your code ensures others can understand and use it effectively. Be sure to include comments and descriptions of your code to make it easier for others to understand and modify your script if necessary.
Following these best practices can help ensure that your data remains clean and accurate while removing commas from a list in Python.
FAQ on Removing Commas from Lists in Python
Here are some frequently asked questions related to removing commas from lists in Python:
Can I remove commas from a list without modifying the original list?
Yes, you can use the .join()
method to create a new string without commas from the original list without modifying the original list.
What if my list contains both integers and strings?
If the list contains both integers and strings, you can either convert all the integers to strings or use conditional statements to check the type of each element before removing the comma.
Can I remove only specific commas from a list?
Yes, you can use the .replace()
method to replace only specific commas with a different character or string.
What if my list has multiple levels of nesting?
If your list has multiple levels of nesting, you can use recursion or iteration to remove commas from all levels.
How can I handle errors while removing commas from a list?
Try-except blocks can handle errors while removing commas from a list. Additionally, performing data validation before data manipulation can help prevent errors.
Are there any libraries that can help with removing commas from lists?
Yes, the re
library can be used to remove commas using regular expressions. The pandas
library can also be used for data manipulation tasks, including removing commas from lists.
Why is removing commas from a list important?
Removing commas from a list is important for various data manipulation tasks, such as converting a comma-separated list to a dictionary or analyzing data in a specific format. Additionally, it helps ensure data accuracy and reliability.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.