If you’ve ever dealt with Python strings that contain brackets, you know how frustrating it can be to extract the desired information.
Whether parsing data or manipulating strings, brackets can get in the way, making your code harder to read and maintain.
This article will show you effective methods to remove brackets from your Python strings. By doing so, you can improve the cleanliness of your code and make it more readable.
We will cover built-in Python string methods, regex, and other helpful techniques to do the job efficiently.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Why should you remove brackets from Python strings?
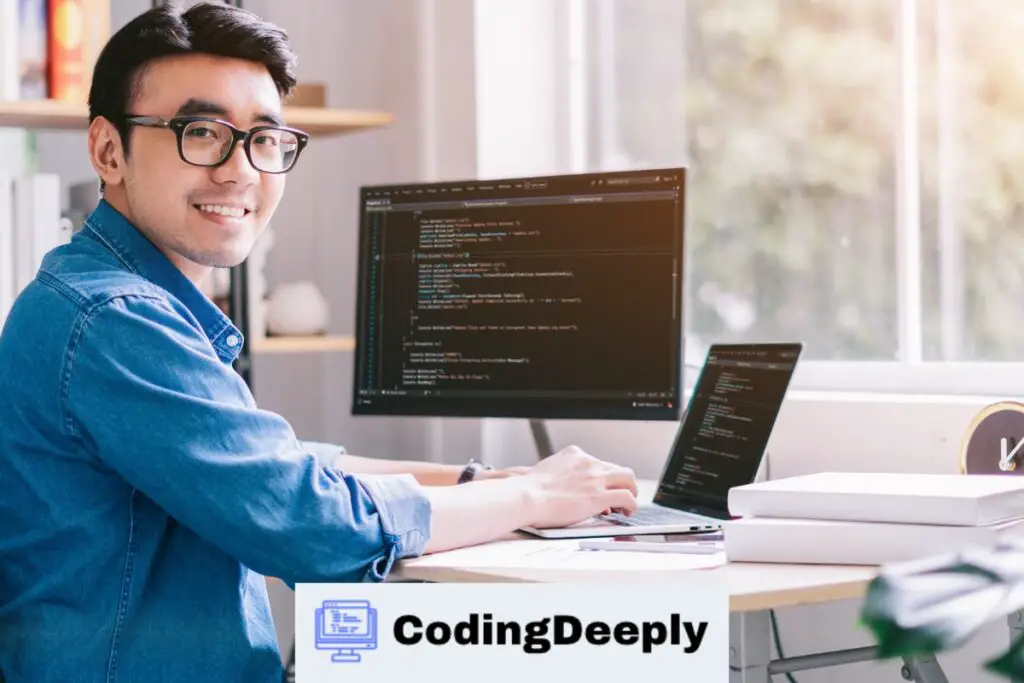
Python strings are a fundamental component of any Python program. They are used to store and manipulate textual data such as words, sentences, and paragraphs. However, strings can become cluttered and difficult to read when they contain unnecessary characters such as brackets.
Removing brackets can significantly improve code cleanliness and readability.
Code cleanliness is an essential aspect of programming. When code is clean, it is easier to understand, maintain, and scale. Removing brackets from Python strings helps achieve code cleanliness by eliminating unnecessary characters that make code confusing or hard to read.
Removing brackets also enhances code readability. When code is easy to read, it is more likely to be understood by other developers. Readable code is particularly important when working on a team or contributing to open source projects.
For example, consider the following string:
employees = [‘John’, ‘Jane’, ‘Mark’, ‘Susan’]
This string contains unnecessary square brackets. Removing them results in a cleaner and more readable string:
employees = ‘John, Jane, Mark, Susan’
Removing brackets from Python strings can be especially beneficial in scenarios where code must be optimized for performance.
In these cases, removing unnecessary characters can lead to more efficient code execution.
Methods to remove brackets from Python strings
There are several ways to remove brackets from Python strings. Here are some popular methods:
- Slice notation: You can use slice notation to remove the first and last characters from a string. This approach assumes that the brackets always appear as the first and last characters, which may not always be true.
- replace() method allows you to replace a specified substring with another substring. In this case, you can replace the bracket characters with an empty string to remove them from the string.
- join() method with split() method: You can use the split() method to split the string at the brackets, then use the join() method to concatenate the resulting substrings without the brackets.
- Regular expressions (regex): Regular expressions can be used to match and remove specific patterns of characters, including brackets. The flexibility of regex makes it a powerful tool for manipulating strings.
Slice notation
The simplest way to remove brackets from a string is to use slice notation. Assuming that the brackets always appear as the first and last characters in the string, you can remove them like this:
string_with_brackets = '[Hello, World!]' string_without_brackets = string_with_brackets[1:-1]
This approach may not work if the brackets appear elsewhere in the string. In that case, you can use one of the other methods described below.
replace() method
The replace() method is a common way to remove specified characters or substrings from a string. In this case, you can use it to remove the brackets:
string_with_brackets = '[Hello, World!]' string_without_brackets = string_with_brackets.replace('[','').replace(']','')
This method will work even if the brackets appear multiple times in the string.
join() method with split() method
The split() method can be used to split the string at a specific character or substring. In this case, we can split the string at the brackets and then concatenate the resulting substrings without the brackets:
string_with_brackets = '[Hello, World!]' string_without_brackets = ''.join(string_with_brackets.split('[')).join(']')
This method may be useful if you need to split the string into multiple parts for further manipulation.
Regular expressions (regex)
Regular expressions provide a flexible way to match and remove specific patterns of characters from a string. In this case, we can use regex to match the brackets and remove them:
import re string_with_brackets = '[Hello, World!]' string_without_brackets = re.sub(r'\[|\]', '', string_with_brackets)
This method is particularly useful if the brackets are nested or otherwise variable in position and frequency.
Using the replace() method
The replace() method in Python is a built-in string method that allows you to replace occurrences of a specified substring within a string with a different substring. This makes it a useful tool for removing brackets from Python strings.
To remove brackets using the replace() method, you can simply call the replace() method on a string and specify the opening and closing brackets as the substring to be replaced. For example:
my_string = "Hello, [World]!"
new_string = my_string.replace('[', '').replace(']', '')
In this example, we first define a string called my_string that contains a pair of square brackets surrounding the word “World”. We then call the replace() method twice, once to replace the opening bracket with an empty string and again to replace the closing bracket with an empty string. This results in a new string called new_string that contains the same text as my_string, but with the brackets removed.
The replace() method is fast and efficient, making it a good choice for removing brackets from large strings or for performing the operation repeatedly. However, it is important to note that the method will only replace the first occurrence of the specified substring by default. To replace all occurrences, you will need to use the optional count parameter.
Using the replace() method with count parameter
To remove all occurrences of brackets from a Python string using the replace() method, you can specify a count of -1. This tells the method to replace all occurrences of the specified substring within the string. For example:
my_string = "Hello, [World]! [How] are [you] doing?"
new_string = my_string.replace('[', '', -1).replace(']', '', -1)
In this example, we first define a string called my_string that contains multiple pairs of square brackets surrounding various words. We then call the replace() method twice, once to replace all opening brackets with an empty string and again to replace all closing brackets with an empty string. This results in a new string called new_string that contains the same text as my_string, but with all brackets removed.
The replace() method is a simple and efficient way to remove brackets from Python strings. It is especially useful for dealing with large strings or for operating repeatedly.
Removing brackets using regular expressions (regex)
Regular expressions, or regex, offer a powerful and flexible approach to manipulating strings in Python. Removing brackets from strings is no exception. This section will explore how to use regex to remove brackets from Python strings.
Regex syntax for removing brackets
The basic syntax for removing brackets using regex is to use the square brackets characters, []
, to specify a set of characters to match. To remove brackets, we can specify the opening and closing brackets as part of this set.
For example, the following regex pattern will match any opening or closing bracket in a string:
[()]
By using the re.sub()
function provided by Python’s built-in re
module, we can replace all matches of this pattern with an empty string, effectively removing all brackets from the original string:
import re original_string = "Hello (world)!" pattern = "[()]" new_string = re.sub(pattern, "", original_string) print(new_string) # Output: "Hello world!"
Regex for nested brackets
If our string contains nested brackets, such as in the case of a dictionary or list representation, we can modify our regex pattern to handle this scenario.
For example, the following pattern will match any set of brackets, including nested ones:
\[(?:[^\[\]]|(?R))*\]
This pattern uses the \[(?:...)*\]
syntax to match any set of brackets, including any characters or other sets of brackets that may be nested inside. The [^\[\]]
part of the pattern matches any character that is not an opening or closing bracket, while the (?R)
part recursively matches any nested bracket sets.
We can also use the re.DOTALL
flag to ensure that the pattern matches across multiple lines:
import re original_string = "{'name': 'John', 'age': [30, (20, 40)]}" pattern = r"\[(?:[^\[\]]|(?R))*\]" new_string = re.sub(pattern, "", original_string, flags=re.DOTALL) print(new_string) # Output: "{'name': 'John', 'age': ()}"
When to use regex for removing brackets
While regex is a powerful and versatile tool for manipulating strings, it can also be complex and difficult to read. In general, using regex to remove brackets should be reserved for cases where the brackets are not easily matched using built-in string methods or other simpler approaches.
If the brackets to be removed, follow a consistent pattern or are easily identifiable, using a simpler string manipulation method may be more readable and maintainable.
Performance considerations when removing brackets
While removing brackets from Python strings can improve code readability and cleanliness, it’s essential to consider performance implications. Here are some key factors to keep in mind:
String length
The length of the string being operated on can significantly affect performance. For small strings, most approaches to removing brackets should perform well.
Still, some methods may become inefficient for longer strings, and certain techniques can become useful.
For example, if you need to remove brackets from a file containing thousands of lines, a regex-based approach may be more efficient than using string methods.
Regex complexity
Regular expressions can be powerful tools for manipulating strings but could become expensive or slow if the pattern becomes too complex.
When using regex to remove brackets, consider the complexity of the pattern and how it could impact performance.
Memory usage
Manipulating strings can consume large amounts of memory, especially when dealing with long strings. Some methods, such as slicing or concatenation, can create new strings in memory, leading to performance issues on large strings.
Consider using in-place modification approaches to reduce memory usage. For example, the string.replace() method modifies the string instead of creating a new one. Additionally, the re.sub() function performs regex substitutions in place, which can be more memory-efficient than creating a new string.
Best practices for removing brackets from Python strings
Removing brackets from Python strings is essential to writing clean and efficient code. Here are some best practices to follow when handling brackets in your code.
1. Use descriptive variable names
When working with strings containing brackets, using descriptive variable names that accurately represent the data is crucial. Variables such as “string1” or “temp” can make your code difficult to read and maintain. Instead, use meaningful names like “text_with_brackets” or “cleaned_text”.
2. Handle potential errors
When removing brackets from strings, it’s important to anticipate any potential errors. For example, your code may break if a string contains no brackets.
Add error handling code, such as try-except blocks or assert statements, to prevent such occurrences to ensure your program runs smoothly.
3. Optimize your code for performance
Removing brackets from large or complex strings can be time-consuming and impact performance.
Consider using efficient techniques such as list comprehensions or generator expressions to optimize your code. Additionally, avoid using unnecessary operations or redundant loops that can slow down your code.
4. Use a consistent approach
When removing brackets from strings in your code, be consistent in your approach. For instance, use the same method to remove brackets throughout your program. Using a consistent approach can improve the readability of your code and make it easier to maintain in the long run.
5. Document your code
Documentation is crucial when it comes to writing clean and maintainable code. When handling brackets in your code, document your approach, assumptions, and any important details to help other developers understand your thought process and avoid confusion or errors.
Frequently Asked Questions (FAQ) about removing brackets from Python strings
Here are some common questions developers might have when removing brackets from Python strings:
What is the best way to remove brackets from a Python string?
The best way to remove brackets from a Python string depends on the use case. Built-in string methods like replace() or strip() can be effective for simple scenarios, while regular expressions can handle more complex situations.
When choosing a method to remove brackets from a string, it’s important to consider factors such as performance, readability, and maintainability.
How can I remove brackets only at the beginning and end of a string?
To remove brackets only at the beginning and end of a string, you can use the strip() method with the specific characters to remove passed as an argument.
For example:
my_string = '[Hello World]' cleaned_string = my_string.strip('[]') print(cleaned_string) # Output: Hello World
How can I remove brackets from strings inside a list or dictionary?
To remove brackets from strings inside a list or dictionary, you can use a loop to iterate over each element and apply the desired string method or regex pattern.
For example:
my_list = ['[1, 2, 3]', '[4, 5, 6]'] cleaned_list = [string.strip('[]') for string in my_list] print(cleaned_list) # Output: ['1, 2, 3', '4, 5, 6']
What if my string has nested brackets?
If your string has nested brackets, you may need to use a recursive function or a more advanced regular expression pattern to remove the brackets effectively.
Testing your code thoroughly is important to ensure all brackets are removed as intended.
How can I handle errors when removing brackets from a string?
To handle errors when removing brackets from a string, you can use try-except blocks to catch any exceptions that might occur. It’s also a good idea to include error-handling messages or logging statements to help with debugging.
Can removing brackets from strings impact performance?
Depending on the size and complexity of the string, removing brackets can impact performance.
More efficient methods, such as regex patterns or built-in string methods, can help mitigate performance issues.
Testing your code and optimizing it as necessary for the specific use case is important.
Are there any caveats to consider when removing brackets from strings?
One caveat to consider when removing brackets from strings is that it can alter the format or structure of the string. This may affect downstream processes or functions that rely on the original string format.
Understanding the implications of removing brackets before implementing this change in your code is necessary.
How do I know if I’ve successfully removed all the brackets from a string?
Print () statements or logging statements can confirm that all brackets have been effectively removed from a string.
You can also write test cases or use a debugger to verify the output of your code. It’s a good practice to test your code thoroughly to ensure it functions as intended.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.