As a Python programmer, you may have encountered situations where you need to read lines from a file or input stream without the newline character.
This can be particularly important when processing text files or working with APIs that expect input in a specific format.
This section will explain the importance of reading lines without newlines in Python and introduce you to Pythonic code that simplifies this task.
We will explore how Pythonic code can simplify the task of reading lines without newlines in Python.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Python’s Newline Character
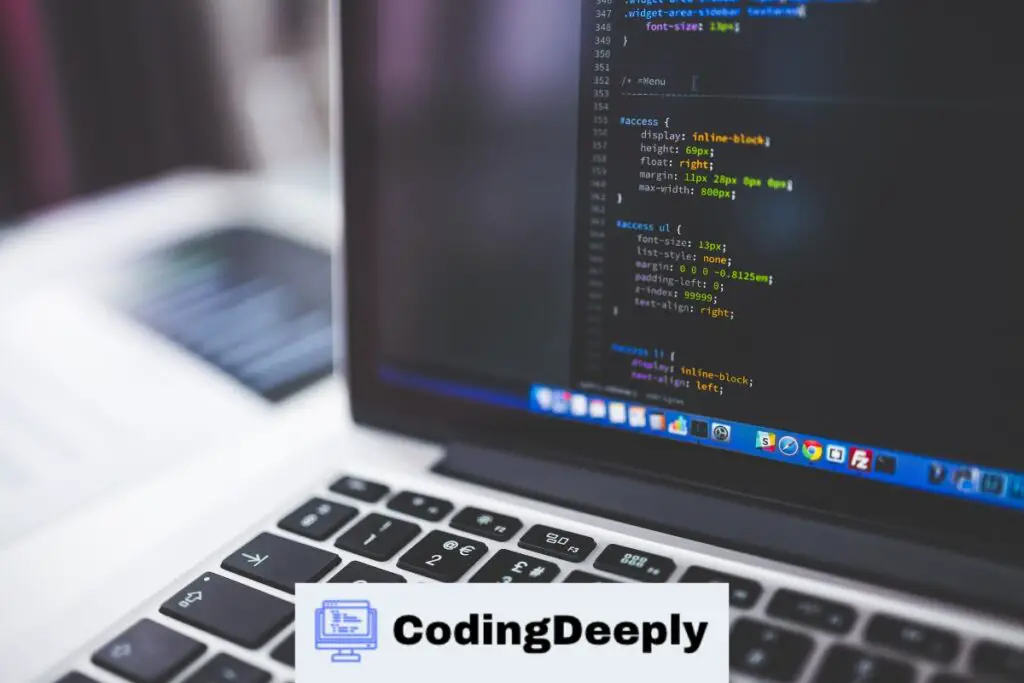
Before delving into how to read lines without newlines in Python, it’s essential to understand what a newline character is and how it is represented in Python.
In Python, a newline character is represented by ‘\n’. This character indicates the end of one line and the beginning of a new one.
However, it’s important to note that different operating systems have different conventions for newlines. For example, Windows uses ‘\r\n’ while Unix uses ‘\n’.
This difference in newline conventions can cause issues when reading and processing text files in Python, especially when the file was created on a different operating system. It’s crucial to handle newlines correctly to ensure the proper functioning of the code.
Reading Lines with Newlines in Python
When reading lines from a file in Python, it is important to consider the newline character used to indicate the end of a line. If the newline character is not handled properly, it can affect the output of your program and create unexpected behavior.
The traditional approach to reading lines in Python involves using the file handling methods:
- Open the file using the open() function
- Call the read() function to read the contents of the file
- Split the contents into separate lines using the split() function
For example:
file = open(‘example.txt’, ‘r’)
content = file.read()
lines = content.split(‘\n’)
However, this method can present challenges when dealing with newlines. For example, if the file contains a line with no newline character at the end, the read() function will not add one.
Conversely, the split() function will create empty lines if a line ends with multiple newline characters.
Therefore, using Pythonic code with string methods is a more robust approach when handling newlines.
This will be discussed in the next section.
The Pythonic Way to Read Lines Without Newlines
Pythonic code refers to code that follows the Python programming language’s best practices and conventions.
When it comes to reading lines without newlines in Python, a more efficient and cleaner method follows these guidelines.
The rstrip() Method
The rstrip() method is a string method in Python that removes whitespace characters from the end of a string. By default, it removes newline characters as well.
Here is an example of using rstrip() to read lines without newlines:
with open('file.txt') as f: lines = [line.rstrip() for line in f]
The code above opens a file named ‘file.txt’ and reads its contents line by line. The rstrip() method is then used to remove any trailing whitespace, including newline characters, from each line.
The resulting list, ‘lines,’ contains the file’s contents without any newlines.
Benefits of this Approach
Using the rstrip() method to read lines without newlines offers several benefits:
- Cleaner code: This method simplifies the code and makes it easier to read and understand.
- Efficient: Removing newlines using rstrip() is faster than using traditional file handling methods.
- Pythonic: This approach follows the guidelines of Pythonic code, improving the overall quality of the code.
Using the rstrip() method to read lines without newlines is a more efficient, cleaner, and Pythonic approach. It is also becoming the standard way to do things in the Python community.
Advantages of Using Pythonic Code
Pythonic code is a term used to describe programming practices that follow the guidelines and conventions of the Python language, resulting in clean code that is easy to read and maintain.
Regarding reading lines without newlines in Python, there are several advantages to using Pythonic code over traditional methods.
Clean Code
Pythonic code emphasizes readability, which means that code is organized and written in a way that makes it easy to understand. This is especially important when reading lines without newlines as it can be easy to introduce errors or make the code difficult to understand.
By using Pythonic code, you can write clean and easy to read code, making it easier to debug and maintain.
Improved Efficiency
Pythonic code is also designed to be efficient and can process data quickly and easily.
When reading lines without newlines, Pythonic code can be much faster and more efficient than traditional methods.
For example, using the rstrip() method to remove the newline character from a string is much faster than using regular expressions or other methods.
Follow Python Coding Standards
Using Pythonic code also means following the coding standards and conventions of the Python language. This ensures that your code is consistent with other Python code and makes it easier for others to understand and maintain your code.
Additionally, following Python coding standards can help you avoid common pitfalls and errors when writing Python code.
Using Pythonic code for reading lines without newlines can result in cleaner, more efficient, and more maintainable code. By following these best practices, you can ensure that your code is easy to understand and follows Python coding standards.
Tips for Handling Newlines in Python
Handling newlines in Python can be tricky, especially when dealing with different operating systems and file formats. Here are some tips and best practices to follow:
1. Use Universal Newlines
When opening files, use the ‘rU’ mode to enable universal newlines. This allows Python to handle different newline conventions automatically. For example:
with open('file.txt', 'rU') as f:
lines = f.readlines()
This will read the lines from ‘file.txt’ and automatically convert any newline convention to the universal newline format.
2. Strip Newlines with rstrip()
When reading lines in Python, use the string method rstrip() to remove any trailing newline characters. For example:
line = 'example\n'
clean_line = line.rstrip() # 'example'
This will remove the newline character from the end of the string.
3. Use the End Parameter in print()
When printing output, use the end parameter in the print() function to specify the end character. By default, print() adds a newline character at the end of each line. For example:
print('example', end='') # 'example'
This will print ‘example’ without a newline character at the end.
4. Follow PEP 8 Guidelines
Follow the PEP 8 guidelines for writing clean and consistent Python code. This includes using four spaces for indentation, writing readable code, and using proper variable naming conventions.
5. Test for Different Scenarios
Test your code for different scenarios, including empty lines, varying newline conventions, and large files. This will ensure that your code is robust and can handle different situations.
Following these tips and best practices, you can avoid common pitfalls when handling newlines in Python and write cleaner, more efficient code.
Real-World Examples of Pythonic Code
To better understand how to read lines in Python without newline characters, let’s look at some real-world examples where the Pythonic code approach is used.
Example 1: Reading Text Files
One common scenario in which Pythonic code is useful is when reading text files. In this example, we are reading a text file called “example.txt” that contains multiple lines of text. We want to read each line of text and remove any trailing newline characters:
with open("example.txt", "r") as f:
lines = [line.rstrip("\n") for line in f]
print(lines)
In this code, we use the Pythonic list comprehension approach to iterate over each line in the file and remove any trailing newline characters using the rstrip() method.
Example 2: Processing Data from APIs
Another common scenario where Pythonic code is useful is when processing data from APIs. In this example, we are making a GET request to an API endpoint that returns multiple lines of JSON data. We want to read each line of JSON data and remove any trailing newline characters:
import requests
response = requests.get("https://api.example.com/data")
lines = [line.rstrip("\n") for line in response.iter_lines()]
print(lines)
In this code, we use the iter_lines() method to iterate over each data line the API returns and remove any trailing newline characters using the rstrip() method.
Example 3: Removing Newlines from Strings
Finally, we can use the Pythonic approach to remove newlines from strings. In this example, we have a string that contains multiple lines of text, separated by newline characters. We want to remove all newline characters from the string:
text = "This is a\nmulti-line\ntext string."\n
text = text.replace("\n", "")
print(text)
In this code, we use the replace() method to replace all newline characters with an empty string, effectively removing the newlines from the original string.
As these examples demonstrate, using Pythonic code for reading lines without newlines can simplify your code and improve efficiency.
FAQ – Frequently Asked Questions
Q: How do I handle empty lines when reading files in Python?
A: When reading files in Python, empty lines are treated as lines containing a newline character. To skip empty lines, check if the line is empty and move on to the next line.
Q: How do I preserve whitespace when reading lines in Python?
A: By default, the Python rstrip() method removes all whitespace from the end of a string, including newlines. If you want to preserve whitespace, you can use the string.rstrip(‘\n’) method instead.
Q: How do I handle different newline conventions when reading files in Python?
A: Specify the newline parameter when opening the file to handle different newline conventions. For example, to read a file with Unix-style newlines on a Windows system, use ‘r’ mode and specify newline=’\n’.
Q: Can I use Pythonic code to process large files?
A: Yes, Pythonic code can process large files efficiently. One approach is to read the file in chunks using the read() method and then process each chunk using Pythonic string methods such as rstrip().
Q: What are some common pitfalls when reading lines in Python?
A: One common pitfall is forgetting to strip the newline character from the end of the line, which can cause errors when processing the data. Another pitfall is not handling exceptions properly when reading files.
Q: How can I test my code for different scenarios when reading lines in Python?
A: It’s important to test your code for different scenarios, such as empty lines, large files, and different newline conventions. You can use test cases and unit tests to verify that your code works as expected.
Q: Should I always use Pythonic code when reading lines in Python?
A: While Pythonic code can be more efficient and readable, there may be cases where the traditional file-handling methods are more appropriate. It’s important to consider your project’s specific requirements and choose the best approach.
Q: What are some best practices for handling newlines in Python?
A: Some best practices include following Python coding standards, handling newline conventions, and testing code for different scenarios. It’s also important to handle exceptions properly and document your code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.