If you’re a Python developer, you might have encountered this error message: SyntaxError: invalid character in identifier.
This error typically occurs when there is a mistake in the syntax of your Python code. It’s frustrating, but fear not! In this section, we’ll explore this error in detail and provide step-by-step solutions to fix it.
Let’s dive into the common causes of this error and ways to resolve it.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Identifiers in Python
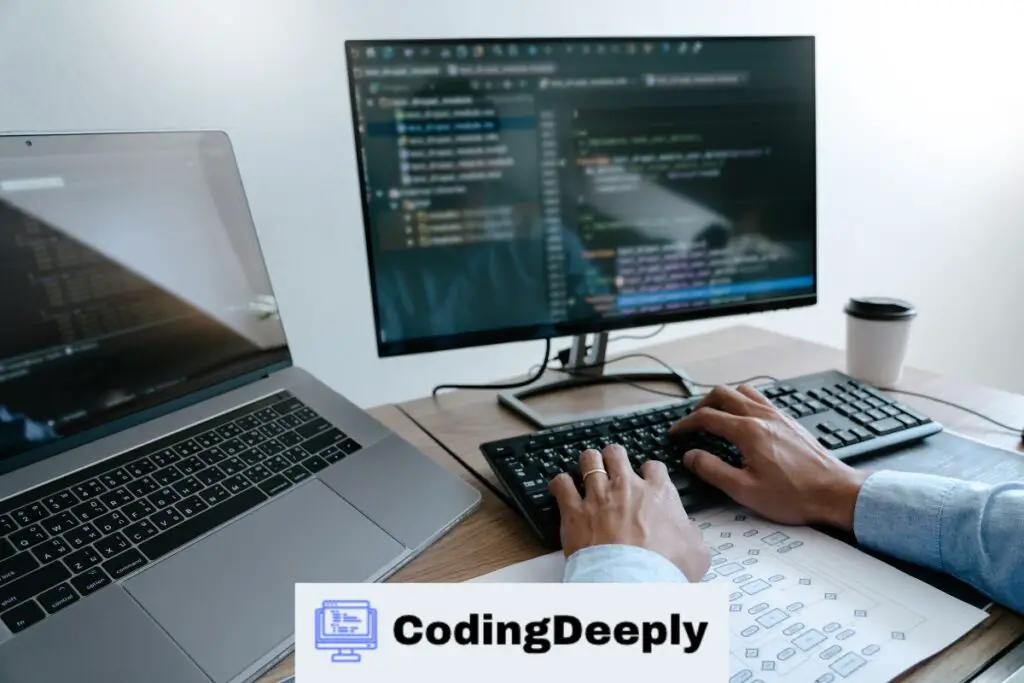
Before we dive into the issue of invalid characters in identifiers, it’s essential to understand what identifiers are in Python. Identifiers represent program elements like variables, functions, and classes.
Identifiers are crucial in programming because they help programmers create readable and well-structured code.
In Python, an identifier can be a combination of letters in lowercase (a-z) or uppercase (A-Z), digits (0-9), or an underscore (_). However, it’s important to follow some specific rules while naming an identifier:
- Identifiers must start with a letter or an underscore, and not with digits.
- Identifiers can’t include special characters like @, $, %, etc.
- Identifiers can’t use reserved words such as if, while, for, def, etc.
Python is a case-sensitive language, which means that uppercase and lowercase letters are distinct. For example, the variable name ‘age’ differs from ‘Age.’
Naming Conventions for Identifiers
Python has some conventions for naming identifiers that help make code more readable. Following these conventions is not mandatory, but it’s good practice. Here are some common conventions:
- Use lowercase letters for variable names.
- Use uppercase letters for constants.
- Use underscores to separate words for readability, like ‘first_name’ instead of ‘firstname.’
Now that we have a basic understanding of identifiers, let’s move on to the next section and explore the causes of invalid characters in identifiers.
Causes of Invalid Characters in Identifiers
Identifiers are an essential part of Python programming to name variables, functions, and other entities. However, SyntaxErrors might occur due to invalid characters in identifiers.
Here are some common causes:
- Using special characters: Identifiers should only contain letters, numbers, and underscores. Using special characters such as %, *, #, and others will result in a SyntaxError.
- Starting identifiers with numbers: Identifiers cannot start with a number, which is considered invalid syntax.
- Using reserved words as identifiers: Python has reserved words that have predefined meanings in the language. Using these words as identifiers will cause a SyntaxError.
Avoiding the above scenarios to prevent encountering the SyntaxError related to invalid characters in identifiers is important.
How to Identify and Fix Invalid Characters
Now that we have discussed the possible causes of invalid characters in Python identifiers let us explore how to identify and fix them.
Step 1: Analyze Error Messages
When encountering a SyntaxError with invalid characters, Python usually prints an error message to help you identify the issue. The error message will contain the line number and a description of the problem.
For instance, if you have a variable named “my$var” in your code, Python will throw the following error:
my$var = 42 SyntaxError: invalid character in identifier
As you can see, the error message points to the variable “my$var” as the source of the problem.
Step 2: Check the Code for Special Characters
Once you have identified the line where the error occurred, you can start analyzing the code to find the invalid character. The most common cause of invalid identifier characters is the use of special characters.
In Python, identifiers can only contain alphabetic characters, numbers, and underscores. They cannot start with a number and must not be a reserved keyword.
Therefore, if you have a variable named “my-Var” or “1_var”, Python will throw a SyntaxError with an invalid character. To fix it, you must remove the special character and only use allowable characters.
Step 3: Use Built-in Functions
Python provides built-in functions that can detect invalid characters in identifiers. For instance, the isidentifier() function checks whether a given string is a valid identifier or not.
You can use this function to detect invalid characters in your code and fix them accordingly.
Here’s an example:
- my_var = 42
- if my_var.isidentifier():
- print(“Valid identifier”)
- else:
- print(“Invalid identifier”)
The code above will output “Valid identifier” since “my_var” is valid.
Similarly, if you have a variable with an invalid character:
- my-var = 42
- if my-var.isidentifier():
- print(“Valid identifier”)
- else:
- print(“Invalid identifier”)
You will get the following error:
if my-var.isidentifier(): SyntaxError: invalid character in identifier
In this case, you can use the replace() function to remove the invalid character:
- my_var = my-var.replace(“-“, “_”)
- if my_var.isidentifier():
- print(“Valid identifier”)
- else:
- print(“Invalid identifier”)
Now, the code will output “Valid identifier” since the invalid character has been removed.
Step 4: Use IDEs with Built-in Error Detection
Most modern Python IDEs provide built-in error detection features that can help you identify and fix issues with invalid characters in identifiers. These features usually highlight the problematic code and provide suggestions for fixing it.
If you are new to Python or struggle with identifying errors in your code, using an IDE with built-in error detection can save you time and headache.
Best Practices for Avoiding Invalid Characters
When programming in Python, it is important to follow certain best practices to avoid encountering SyntaxError due to invalid identifier characters. Here are some tips to keep in mind:
- Follow proper naming conventions: Identifiers should begin with a letter or an underscore character. Avoid starting identifiers with numbers or special characters.
- Avoid using reserved words: In Python, certain words are reserved for specific purposes. Using these words as identifiers can lead to SyntaxError. Check the Python documentation for a list of reserved words and avoid using them as identifiers.
- Use descriptive identifiers: Use meaningful and descriptive words for naming variables, functions, and other entities in your code. Avoid using abbreviations or acronyms that might confuse others who read your code.
- Attention to code formatting: Proper indentation and consistent code formatting can help you identify any errors in your code, including invalid characters in identifiers.
- Use Python IDEs with built-in error detection: Some Python IDEs have built-in error detection that can highlight issues such as invalid characters in identifiers. Use these tools to make your coding experience more efficient and error-free.
Frequently Asked Questions (FAQ)
Here are some common questions related to Python SyntaxError with invalid characters in identifiers:
Q: What does “SyntaxError: invalid character in identifier” mean?
A: This error message indicates an issue with the characters used in an identifier (such as a variable or function name) in your Python code.
Q: What are some common causes of invalid characters in identifiers?
A: Some causes include using special characters like “!” or “-“, starting an identifier with a number, or using a reserved word in Python as an identifier.
Q: How do I identify where the invalid character is in my code?
A: The error message often includes the line number where the issue occurred. You can also try looking for any special characters or incorrect naming convention within the identifier.
Q: How can I fix the SyntaxError with invalid characters?
A: Once you have identified the location of the invalid character, you can either remove it or replace it with a valid character.
Q: How can I avoid encountering this error in the future?
A: Following best practices such as using appropriate naming conventions, avoiding special characters, and using Python IDEs with built-in error detection can help prevent encountering this error.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.