If you are looking for a way to simplify your data manipulation in Python, then look no further than summing dictionary values by key.
Dictionaries are powerful data structures in Python that allow you to store data in key-value pairs.
And summing the values of a particular key is a highly useful operation that can help you better understand your data and make more informed decisions.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Dictionaries in Python
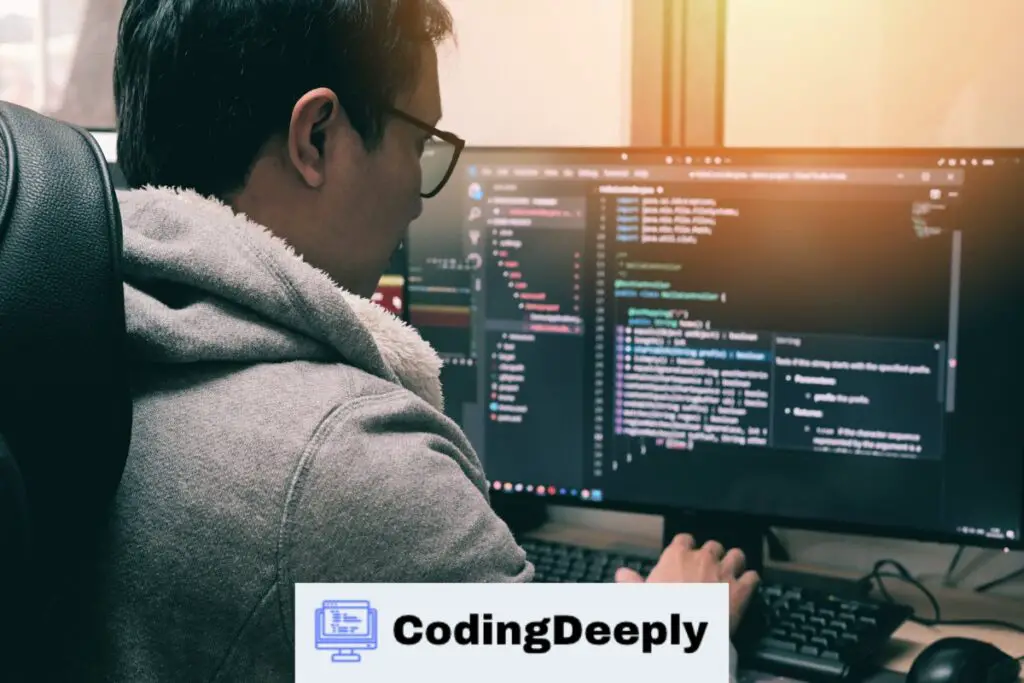
Before diving into how to sum dictionary values by key in Python, it’s important to understand what dictionaries are and how they work in Python programming. Dictionaries are data structures that store key-value pairs.
Each key in a dictionary must be unique and associated with a value. Dictionaries are denoted by curly braces {} with each key-value pair separated by a colon. For example:
{'apple': 2, 'banana': 3, 'orange': 4}
In the above example, ‘apple’, ‘banana’, and ‘orange’ are the keys, and 2, 3, and 4 are the corresponding values.
Accessing and Modifying Dictionary Elements
To access a value in a dictionary, you can use the key in square brackets. For example:
fruit_dict = {'apple': 2, 'banana': 3, 'orange': 4}
print(fruit_dict['apple']) # Outputs 2
You can also modify the value associated with a key by using the key in square brackets and assigning a new value. For example:
fruit_dict = {'apple': 2, 'banana': 3, 'orange': 4}
fruit_dict['apple'] = 5
print(fruit_dict) # Outputs {'apple': 5, 'banana': 3, 'orange': 4}
Dictionary Methods
Python provides a number of built-in methods for dictionaries that can be useful when working with them. Some common methods include:
keys()
: Returns a list of all the keys in the dictionaryvalues()
: Returns a list of all the values in the dictionaryitems()
: Returns a list of all the key-value pairs in the dictionary as tuplesget()
: Returns the value associated with a key, or a default value if the key does not existupdate()
: Updates the dictionary with key-value pairs from another dictionary
By understanding how these methods work, you can efficiently manipulate and analyze data stored in dictionaries.
Summing Dictionary Values by Key
Python’s dictionaries are a powerful data structure that allows you to store and manipulate data in key-value pairs. One common task when working with dictionaries is summing the values by key.
Fortunately, Python provides a simple and efficient way to perform this operation.
Method 1: Using a loop
One way to sum dictionary values by key is to use a loop. The following code demonstrates how to do this:
my_dict = {'a': 100, 'b': 200, 'c': 300, 'd': 400, 'e': 500} result_dict = {} for key, value in my_dict.items(): if key in result_dict: result_dict[key] += value else: result_dict[key] = value print(result_dict)
In this example, we create a new dictionary result_dict
to store the summed values. We then loop through the original dictionary my_dict
using the items()
method to access both the key and value for each item.
We then check if the key already exists in result_dict
, and if it does, we add the current value to the existing value for that key.
If the key doesn’t exist yet, we set the value for that key equal to the current value. Finally, we print out the resulting dictionary with the summed values.
Method 2: Using the defaultdict class
Another way to sum dictionary values by key is to use the defaultdict
class from the collections
module.
This class provides a default value for any key that hasn’t been seen before, which can be useful in cases where you want to avoid having to check if a key exists in the dictionary manually.
from collections import defaultdict my_dict = {'a': 100, 'b': 200, 'c': 300, 'd': 400, 'e': 500} result_dict = defaultdict(int) for key, value in my_dict.items(): result_dict[key] += value print(dict(result_dict))
In this example, we import the defaultdict
class and create a new instance of it with a default value of int
(which will default to 0). We then follow a similar process as in the previous example, looping through the original dictionary and adding the values to the appropriate keys in the result_dict
.
Finally, we convert the resulting defaultdict
object to a regular dictionary for printing.
Method 3: Using the Counter class
Finally, we can also use the Counter
class from the collections
module to sum dictionary values by key.
This class provides a shorthand way to count the occurrences of elements in a list or other iterable, which can be adapted to summing values in a dictionary.
from collections import Counter my_dict = {'a': 100, 'b': 200, 'c': 300, 'd': 400, 'e': 500} result_dict = dict(Counter(my_dict) + Counter()) print(result_dict)
In this example, we first create a new class instance for the original dictionary, which will count the occurrences of each key-value pair. We then create another empty Counter object, adding to the first one using the +
operator.
This will create a new Counter object that sums the values for any keys present in both dictionaries. Finally, we convert the resulting Counter
object to a regular dictionary for printing.
Enhancing Data Manipulation Skills
Python programming allows for advanced data manipulation capabilities. These capabilities enable users to efficiently analyze data and extract its most relevant information. Below are some tips for enhancing your data manipulation skills with Python.
1. Utilize comprehensive libraries
Python has numerous libraries that provide efficient and comprehensive ways of manipulating data structures. These libraries include NumPy, Pandas, and SciPy. They provide tools for data analysis, manipulation, and visualization.
Being familiar with these libraries and their functions will give you more control over your data and enable you to work more efficiently.
2. Simplify data manipulation with comprehension
Comprehensions are a powerful tool for data manipulation in Python. They enable you to perform complex operations on data structures in a single line of code.
List comprehensions, for example, can be used to filter, map, and reduce data in a list.
Similarly, dictionary comprehensions can be used to create or modify existing dictionaries. Being proficient in comprehension will make your code more concise and readable.
3. Develop a strong understanding of data types and structures
Python has different data types and structures with unique characteristics that are used differently. Being proficient in these data types and structures will increase your capability to manipulate data.
Familiarize yourself with data types like strings, integers, booleans, and structures like lists and dictionaries.
Python allows for creating user-defined data types and structures, so having a strong understanding of how to create these structures will allow for even more advanced data manipulation.
By utilizing comprehensive libraries, simplifying data manipulation with comprehension, and developing a strong understanding of data types and structures, you can enhance your data manipulation skills with Python programming.
These tips will enable you to analyze and gain insights from data more efficiently and effectively, making you a more proficient data analyst or programmer.
FAQ – Frequently Asked Questions
Here are some of the frequently asked questions regarding summing dictionary values by key in Python:
1. What is a dictionary in Python?
A dictionary is an unordered collection of elements stored as key-value pairs. It resembles a hash table where each key is unique and maps to a specific value.
In Python, dictionaries are enclosed in curly braces and a colon separates each key-value pair.
2. How do I sum the values in a dictionary in Python?
To sum up, the values associated with a particular key in a dictionary, you can create an empty dictionary and iterate over the original one to populate the new one with the summed values.
Alternatively, you can use the defaultdict class from the collections module to automatically create a new key with a default value of 0 if the key doesn’t exist already.
3. Can I use Python to manipulate data in other formats besides dictionaries?
Absolutely! Python is a versatile language that can manipulate data in various formats, including lists, tuples, and arrays. It also has modules for working with databases, spreadsheets, and other data formats.
4. What other skills should I learn to enhance my data manipulation abilities in Python?
In addition to understanding dictionaries and key-value pairs, learning about data cleaning and preprocessing techniques, data visualization libraries such as Matplotlib and Seaborn, and machine learning algorithms for predictive modeling is recommended.
5. Where can I find more resources for learning Python?
Many resources are available for learning Python, including online courses, books, and tutorials. Some popular options include Codecademy, Code School, and Udemy.
Additionally, the Python documentation provides extensive resources for learning the language and its various modules.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.