Python is a popular programming language for various applications, including string manipulation.
When removing multiple characters from strings, Python offers several techniques that are both easy to use and efficient.
This article will discuss how to strip multiple characters from strings in Python without compromising performance.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
An Overview of the Strip Method in Python
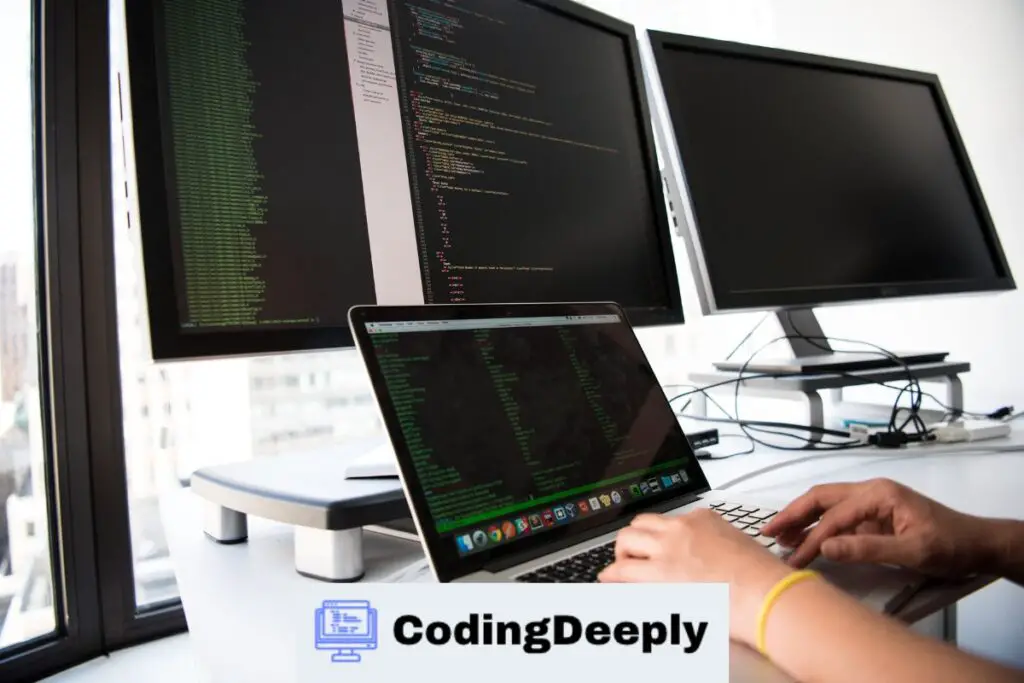
Before we dive into the details of stripping multiple characters in Python, let’s first provide an overview of the strip method. This method removes characters from the beginning and end of a string in Python.
It can be particularly useful when dealing with whitespace or line breaks.
The strip method takes an optional argument that specifies which characters should be removed from the string.
The method will remove whitespace characters by default if no argument is provided.
How to Use the Strip Method
Here’s an example of how to use the strip method:
string = " hello world! "
new_string = string.strip()
print(new_string)
The output of this code will be “hello world!”, with the leading and trailing whitespace removed.
It’s also possible to specify which characters to remove by passing them as an argument:
string = "!!!hello world!!"
new_string = string.strip("!")
print(new_string)
The output of this code will be “hello world”, with all exclamation marks removed from the beginning and end of the string.
It’s important to note that the strip method only removes characters from the beginning and end of the string. If you need to remove characters from the middle of a string, you’ll need to use a different method, such as the replace method or regular expressions, as discussed in later sections.
Stripping Single Characters from Strings in Python
When it comes to removing single characters from a string, Python provides a straightforward solution through the use of the strip method.
Using the strip() method
The strip method we introduced in the previous section can be applied to remove a single character from a string.
To do so, we need to specify the character to be removed within the parentheses when calling the strip method. For instance, to remove the letter ‘a’ from the string ‘banana’, we would use the following code:
'banana'.strip('a')
The output of this code would be ‘bnn’, which shows that all instances of the letter ‘a’ have been removed from the original string.
Note that the strip method only removes the specified character(s) at the beginning and end of the string. To remove all occurrences of the character within the string, we can use the replace method, which we will cover in the next section.
Example: Stripping vowels from a string
Let’s try an example where we remove all vowels from the string ‘hello world’. To achieve this, we need to specify all the vowel characters within the parentheses when calling the strip method:
'hello world'.strip('aeiou')
The output of this code would be ‘hll wrld’, which shows that all vowels have been successfully removed from the original string.
Using the strip method, we can easily remove single characters from strings in Python.
Stripping Multiple Characters Using Replace Method in Python
While the strip method is a straightforward way to remove multiple characters from strings in Python, it has limitations. Specifically, the strip method only removes characters from the beginning and end of a string.
You can use the replace method if you need to remove characters from the middle of a string.
Using the Replace Method
To use the replace method, specify the character(s) you want to remove and the character(s) you want to replace them with. Here’s an example:
text = "Hello, world! How are you?" new_text = text.replace(",", "").replace("!", "") print(new_text)
In this example, we replace commas and exclamation points with an empty string (i.e., we remove them).
The resulting output is:
Hello world How are you
Note that the replace method doesn’t modify the original string (i.e., it returns a new string with the specified replacements).
If you want to modify the original string, you can assign the new string to the original variable:
text = "Hello, world! How are you?" text = text.replace(",", "").replace("!", "") print(text)
Differences Between Strip and Replace Methods
While both the strip and replace methods can remove characters from strings in Python, they have different functionalities.
The strip method only removes characters from the beginning and end of a string, while the replace method can remove characters from anywhere in the string.
Additionally, the strip method only removes characters if they match exactly (i.e., it doesn’t remove substrings). The replace method, on the other hand, can replace substrings with an empty string.
For example, consider the following string:
text = "This is an example string."
If we want to remove the word “is” from the string, we can use the replace method:
new_text = text.replace("is", "") print(new_text)
Output:
Th an example string.
Notice that the replace method also removed the “is” in “example.”
However, if we try to use the strip method to remove “is,” it won’t work:
new_text = text.strip("is") print(new_text)
Output:
This is an example string.
As you can see, the strip method only removed the “i” and “s” from the beginning and end of the string, respectively.
Stripping Multiple Characters Using Regular Expressions in Python
Regular expressions, or regex, provide a powerful and flexible way to pattern-match strings in Python.
They are particularly useful for efficiently and precisely removing multiple characters from strings.
What are regular expressions?
Regular expressions are a sequence of characters that define a search pattern. They can be used to search, replace, and manipulate text in Python.
Regular expressions use special characters and syntax to create rules matching specific text patterns.
How can regular expressions be used to remove multiple characters?
We can use the function to remove multiple characters from a string using regular expressions.
This function searches a string for a specified pattern and replaces all occurrences of that pattern with a specified string.
For example, suppose we want to remove all occurrences of the letters ‘a’ and ‘b’ from the following string:
<code>string = "apple banana cherry"</code>
We can use the following regular expression:
<code>pattern = "[ab]"</code>
This regular expression matches any occurrence of the letters ‘a’ or ‘b’ in the string. We can then use the re.sub()
function to replace all occurrences of this pattern with an empty string:
<code>import re
string = "apple banana cherry"
pattern = "[ab]"
new_string = re.sub(pattern, "", string)</code>
The resulting new_string
would be:
<code>"ple nnn chery"</code>
As we can see, the letters ‘a’ and ‘b’ have been removed from the string.
What are some other examples of using regular expressions to strip multiple characters?
Here are a few more examples of regular expressions that could be used to strip multiple characters from strings in Python:
- Remove all whitespace characters:
-
<code>pattern = "\s+"</code>
- Remove all non-alphanumeric characters:
-
<code>pattern = "[^a-zA-Z0-9]+"</code>
- Remove all digits:
-
<code>pattern = "\d+"</code>
We can use these regular expressions with the re.sub()
function to remove the specified characters from a string.
Overall, regular expressions provide a powerful and flexible way to pattern-match and manipulate strings in Python. They can be particularly effective for stripping multiple characters efficiently and with precision.
Frequently Asked Questions (FAQ) – Stripping Multiple Characters in Python
If you have been following this article, you may have questions about stripping multiple characters in Python. Here are some frequently asked questions that we hope will provide additional clarity and assistance for our readers.
Q: What is the difference between the strip and replace methods for stripping multiple characters in Python?
A: The strip method removes characters from the beginning and end of a string. It removes all occurrences of the specified characters until it hits a character not in the set of characters to be removed.
On the other hand, the replace method replaces all occurrences of the specified characters with another specified character or string.
Q: Can the strip method remove multiple characters in the middle of a string?
A: No, the strip method removes characters only from the beginning and end of a string. If you need to remove characters from the middle of a string, you can use the replace method with an empty string as the replacement argument.
Q: What are regular expressions, and how can they be used to strip multiple characters in Python?
A: Regular expressions are a powerful tool for pattern matching in strings. They allow you to specify a pattern and search for all occurrences of that pattern in a string.
In the context of stripping multiple characters in Python, regular expressions can be used to match and remove specific patterns of characters.
Q: Can the strip method and regular expressions be used together to strip multiple characters in Python?
A: You can use the strip method and regular expressions together to achieve more complex stripping tasks. For example, you can use the strip method to remove a set of characters from the beginning and end of a string and then use regular expressions to remove specific characters from the middle of the string.
Q: Are there any limitations to using regular expressions for stripping multiple characters in Python?
A: Regular expressions can be complex and difficult to understand for beginners. They also have the potential to match unintended characters if the pattern is not specified correctly.
Additionally, using regular expressions for simple stripping tasks may be overkill and less efficient than using a simpler method like the strip method.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.