If you have encountered the error message “Python String Error: ‘str’ Object Does Not Support Item Assignment,” then you may have been attempting to modify a string object directly or assigning an item to a string object incorrectly.
This error message indicates that the ‘str’ object type in Python is immutable, meaning that once a string object is created, it cannot be modified.
In this article, we will dive into the details of this error message, explore why it occurs, and provide solutions and best practices to resolve and prevent it.
By the end of this article, you will have a better understanding of how to work with strings in Python and avoid common mistakes that lead to this error.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the error message
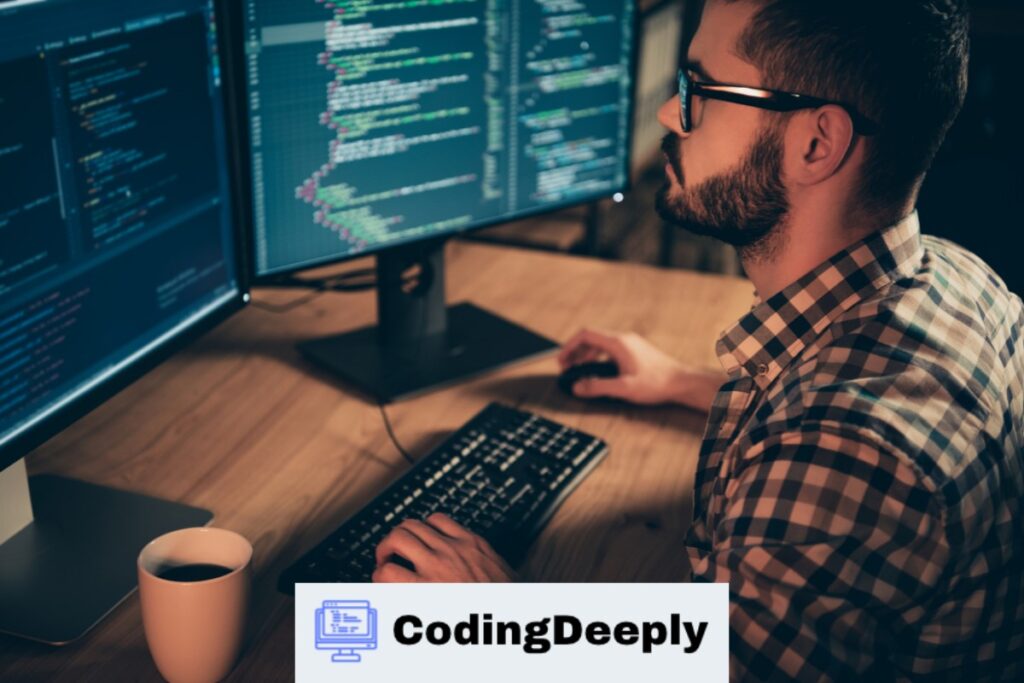
When encountering the Python String Error: ‘str’ Object Does Not Support Item Assignment, it’s essential to understand what the error message means.
This error message typically occurs when one attempts to modify a string directly through an item assignment.
Strings in Python are immutable, meaning that their contents cannot be changed once they have been created. Therefore, when trying to assign an item to a string object, the interpreter throws this error message.
For example, consider the following code snippet:
string = “hello”
string[0] = “H”
When executing this code, the interpreter will raise the Python String Error: ‘str’ Object Does Not Support Item Assignment. Since strings are immutable in Python, it’s impossible to change any individual character in the string object through item assignment.
It’s important to note that this error message is solely related to item assignment. Other string manipulations, such as concatenation and slicing, are still possible.
Understanding the ‘str’ object
The ‘str’ object is a built-in data type in Python and stands for string. Strings are a collection of characters enclosed within single or double quotes, and in Python, these strings are immutable.
While it’s impossible to modify an existing string directly, we can always create a new string using string manipulation functions like concatenation, replace, and split, among others.
In fact, these string manipulation functions are specifically designed to work on immutable strings and provide a wide range of flexibility when working with strings.
Common causes of the error
The “Python String Error: ‘str’ Object Does Not Support Item Assignment” error can occur due to various reasons. Here are some of the common causes:
1. Attempting to modify a string directly
Strings are immutable data types, meaning their values cannot be changed after creation.
Therefore, trying to modify a string directly by assigning a new value to a specific index or item will result in the “Python String Error: ‘str’ Object Does Not Support Item Assignment” error.
Example:
string = "Hello World"
string[0] = "h"
This will result in the following error message:
TypeError: 'str' object does not support item assignment
2. Misunderstanding the immutability of string objects
As mentioned earlier, string objects are immutable, unlike other data types like lists or dictionaries.
Thus, attempting to change the value of a string object after it is created will result in the “Python String Error: ‘str’ Object Does Not Support Item Assignment” error.
Example:
string = "Hello World"
string += "!"
string[0] = "h"
This will result in the following error message:
TypeError: 'str' object does not support item assignment
3. Using the wrong data type for assignment
If you are trying to assign a value of the wrong data type to a string, such as a list or tuple, you can encounter the “Python String Error: ‘str’ Object Does Not Support Item Assignment” error.
Example:
string = "Hello World"
string[0] = ['h']
This will result in the following error message:
TypeError: 'list' object does not support item assignment
Ensure that you use the correct data type when assigning values to a string object to avoid this error.
Resolving the error
There are several techniques available to fix the Python string error: ‘str’ Object Does Not Support Item Assignment.
Here are some solutions:
Using string manipulation methods
One way to resolve the error is to use string manipulation functions that do not require item assignment.
For example, to replace a character in a string at a specific index, use the replace() method instead of assigning a new value to the index. Similarly, to delete a character at a particular position, use the slice() method instead of an item assignment.
Creating a new string object
If you need to modify a string, you can create a new string object based on the original.
One way to modify text is by combining the portions before and after the edited section. This can be achieved by concatenating substrings.
Alternatively, you can use string formatting techniques to insert new values into the string.
Converting the string to a mutable data type
Strings are immutable, which means that their contents cannot be changed.
Nevertheless, you can convert a string to a mutable data type such as a list, modify the list, and then convert it back to a string. Be aware that this approach can have performance implications, especially for larger strings.
When implementing any of these solutions, it’s essential to keep in mind the context of your code and consider the readability and maintainability of your solution.
Best practices to avoid the error
To avoid encountering the “Python String Error: ‘str’ Object Does Not Support Item Assignment,” following some best practices when working with string objects is important.
Here are some tips:
1. Understand string immutability
Strings are immutable objects in Python, meaning they cannot be changed once created.
Attempting to modify a string directly will result in an error. Instead, create a new string object or use string manipulation methods.
2. Use appropriate data types
When creating variables, it is important to use the appropriate data type. If you need to modify a string, consider using a mutable data type such as a list or bytearray instead.
3. Utilize string manipulation functions effectively
Python provides many built-in string manipulation functions that can be used to modify strings without encountering this error. Some commonly used functions include:
- replace() – replaces occurrences of a substring with a new string
- split() – splits a string into a list of substrings
- join() – combines a list of strings into a single string
- format() – formats a string with variables
4. Avoid using index-based assignment
Index-based assignment (e.g. string[0] = ‘a’) is not supported for strings in Python. Instead, you can create a new string with the modified value.
5. Be aware of context
When encountering this error, it is important to consider the context in which it occurred. Sometimes, it may be due to a simple syntax error or a misunderstanding of how strings work.
Taking the time to understand the issue and troubleshoot the code can help prevent encountering the error in the future.
By following these best practices and familiarizing yourself with string manipulation methods and data types, you can avoid encountering the “Python String Error: ‘str’ Object Does Not Support Item Assignment” and efficiently work with string objects in Python.
FAQ – Frequently asked questions
Here are some commonly asked questions regarding the ‘str’ object item assignment error:
Q: Why am I getting a string error while trying to modify a string?
A: Python string objects are immutable, meaning they cannot be changed once created. Therefore, you cannot modify a string object directly. Instead, you must create a new string object with the desired modifications.
Q: What is an example of an item assignment with a string object?
A: An example of an item assignment with a string object is attempting to change a character in a string by using an index. For instance, if you try to modify the second character in the string ‘hello’ to ‘i’, as in ‘hillo’, you will get the ‘str’ object item assignment error.
Q: How can I modify a string object?
A: There are a few ways to modify a string object, such as using string manipulation functions like replace() or split(), creating a new string with the desired modifications, or converting the string object to a mutable data type like a list and then modifying it.
Q: Can I prevent encountering this error in the future?
A: Yes, here are some best practices to avoid encountering this error: use appropriate data types for the task at hand, understand string immutability, and use string manipulation functions effectively.
Diving deeper into Python data structures and understanding their differences, advantages, and limitations is also helpful.
Q: Why do I need to know about this error?
A: Understanding the ‘str’ object item assignment error is essential for correctly handling and modifying strings in Python.
This error is a common source of confusion and frustration among Python beginners, and resolving it requires a solid understanding of string immutability, data types, and string manipulation functions.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.