Python is an incredibly versatile programming language used for various tasks. One common task is reading input from stdin, also known as standard input.
This allows users to enter data into a program through the command line or other input channels.
However, it’s important to properly handle the end of file (EOF) condition when reading input from stdin, especially when dealing with large amounts of data or long-running programs.
In this article, we’ll explore how to read input from stdin in Python and how to handle the end-of-file condition.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Standard Input (stdin) in Python
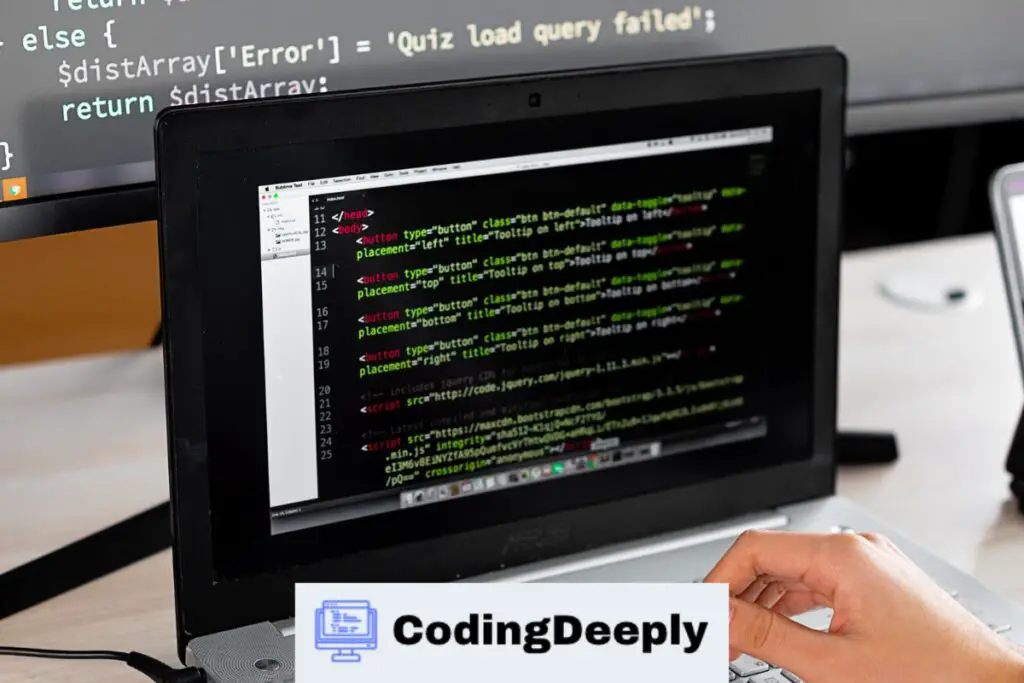
Standard input, or stdin, is a fundamental feature of most programming languages. It allows programs to read input from the user or other sources.
In Python, standard input is a file-like object accessed using the sys module.
When a Python program is executed in a terminal or console, stdin is typically connected to the keyboard. This means that any text entered by the user will be read by the program using stdin.
Reading Input from stdin in Python
Standard input (stdin) is a stream of data that comes into a program from an input device such as a keyboard or a file. In Python, you can read input from the user using the input() function or by reading data from stdin.
There are various methods available in Python for reading input from stdin. The most commonly used method is the input() function, which reads a line of text from stdin and returns it as a string.
Another method is to use the sys.stdin.readline() function reads a line of text from stdin and returns it as a string. The fileinput module can also be used to read input from stdin.
Using the input() Function
The input() function reads a line of text from the user and returns it as a string. It can be used to read input from stdin in the following way:
- Prompt the user to enter input using the print() function
- Read the input entered by the user using the input() function
- Process the input as required
For example:
print("Enter your name:") name = input() print("Hello, " + name)
This code prompts the user to enter their name, reads the input using the input() function, and then prints a greeting message.
Using the sys.stdin.readline() Function
The sys.stdin.readline() function reads a line of text from stdin and returns it as a string. It can be used to read input from stdin in the following way:
- Import the sys module
- Read a line of text from stdin using the sys.stdin.readline() function
- Process the input as required
For example:
import sys line = sys.stdin.readline().strip() print("You entered: " + line)
This code reads a line of text from stdin using the sys.stdin.readline() function, strips any leading or trailing whitespace, and then prints the input entered by the user.
Using the fileinput Module
The fileinput module can also be used to read input from stdin. It provides a convenient way to read input from both files and stdin.
To use the fileinput module to read input from stdin, you can use the following code:
import fileinput for line in fileinput.input(): # Process the input as required
This code reads input from stdin line by line, and processes each line as required. It can be used in the same way as reading input from a file.
These are some of the methods available in Python for reading input from stdin. You can choose the method that best suits your requirements and use it to read input from stdin in your program.
Handling End of File (EOF) in Python
End of File (EOF) is a signal that indicates the end of data in a file or when reading from stdin. In Python, detecting EOF and handling it appropriately is crucial to ensure that the program doesn’t hang or fail unexpectedly.
Detecting EOF using a while loop
One of the most common ways to detect EOF when reading from stdin is to use a while loop that reads input until there is no more data. Here is an example:
while True: try: line = input() except EOFError: break # do something with line
This code reads input from stdin one line at a time using the input() function.
If there is no more data, the input() function raises an EOFError exception, which the try-except block catches. The while loop is then exited using the break keyword.
Detecting EOF using an empty string
Another way to detect EOF when reading from stdin is to check for an empty string. This approach is often used with the sys.stdin.readline() method. Here is an example:
import sys for line in sys.stdin: if line.strip() == '': break # do something with line
This code reads input from stdin one line at a time using the sys.stdin.readline() method. If an empty string is read, there is no more data, and the for loop is exited using the break keyword.
Reading stdin Until EOF: Step-by-Step Instructions
Now that you understand the basics of reading input from stdin in Python, let’s walk through a step-by-step guide until the end of file (EOF).
Step 1: Import Required Libraries
First, you must import the necessary libraries at the beginning of your Python program.
- To read input from stdin, you need to import the
sys
library. - If you plan to use the
fileinput
module, you should also import it at the beginning of your program.
Here’s an example:
import sys import fileinput
Step 2: Begin the Loop
The next step is to begin a loop that will continue until the end of file (EOF) is reached.
Here’s an example:
for line in sys.stdin: # do something with the input pass
Alternatively, you can use a while loop to continue reading input until EOF:
while True: line = sys.stdin.readline() if not line: break # do something with the input pass
Step 3: Process the Input
Within the loop, you can process the input as needed for your specific program.
For example, you might want to split the input into words or perform some calculations on the input.
Here’s an example:
total = 0 for line in sys.stdin: words = line.strip().split() for word in words: total += int(word) print(total)
Step 4: End the Loop
When the end of file (EOF) is reached, the loop will end automatically. However, you might want to include additional code to handle any tasks that need to be performed after the loop has ended.
Here’s an example:
for line in sys.stdin: # do something with the input pass # perform some final tasks after the loop has ended print("End of file reached")
And that’s it!
You now understand how to read input from stdin until the end of file (EOF) in Python.
Best Practices for Reading stdin in Python
When reading input from stdin in Python, it is important to implement certain best practices to ensure efficient and error-free execution. Here are some tips to follow:
1. Use the Right Input Method for the Job
Python offers multiple methods for reading input from stdin, each with its own strengths and weaknesses. For example, the input() function is convenient but inefficient for large inputs. Consider the input size, required performance, and data structure requirements before choosing an appropriate method.
2. Validate Input Before Processing
Always validate input before processing it to prevent errors and improve program efficiency. This can be done using try-except blocks, regular expressions, or user-defined functions.
3. Avoid Repetitive Code
If you are typing the same code repeatedly, consider using a function to avoid redundancy. This can make your code easier to read, modify, and maintain.
4. Provide Meaningful Input Prompts
Informative prompts help users provide correct input and prevent errors. These prompts should be clear, concise, and relevant to the input required.
5. Use Error Handling Techniques
Always incorporate error-handling techniques, such as try-except blocks and assertion statements, to catch and handle errors. This can prevent program crashes and unexpected behavior.
6. Handle Exceptions Gracefully
When exceptions are raised, handle them gracefully to prevent program crashes. This involves providing informative error messages and guiding users toward possible solutions.
7. Optimize Performance
Efficient input handling can improve program performance. Consider optimizing your code by using faster input methods, minimizing redundant input validation, and breaking down input processing into smaller, more manageable tasks.
By following these best practices, you can ensure efficient, error-free input handling in your Python programs. Always consider the unique requirements of your program and choose the input method and validation techniques that meet these needs.
Common Issues and Troubleshooting
While reading input from stdin is a straightforward process, occasionally, problems can arise that can disrupt the flow of your program. This section will address some common issues and provide troubleshooting tips and solutions.
Issue: Program hangs when waiting for input
If your program appears to be hanging when waiting for input from stdin, it may be because the input buffer is not being flushed properly.
One solution is to use the flush() method to clear the input buffer before requesting additional input.
Issue: “ValueError: not enough values to unpack” error
If you are using the input() function to read input from stdin and encounter a “ValueError: not enough values to unpack” error, it may be because the input string does not contain enough values to unpack into the specified variables.
To fix this, make sure that the input string contains the correct number of values and that the correct delimiter separates them.
Issue: “EOFError: EOF when reading a line” error
The “EOFError: EOF when reading a line” error is often encountered when the program reaches the end of the file without detecting the end of file (EOF) condition. One solution is to use a try-except block to catch the EOFError and handle it appropriately.
Issue: Unexpected input
If your program receives unexpected input from stdin, it may be because the input is not being sanitized or validated properly.
Ensure to validate input and handle errors and edge cases appropriately to avoid unexpected behavior.
By following best practices and troubleshooting tips, you can ensure that your program reads input from stdin efficiently and without errors.
FAQ: Frequently Asked Questions
Here are some of the most common questions and concerns about reading input from stdin in Python:
Q: What is stdin in Python?
A: stdin stands for “standard input,” a built-in file object in Python that represents the input stream from which a program can read data.
Q: What is EOF in Python?
A: EOF stands for “end of file,” which is a condition that indicates that there is no more data to be read from a file or other input stream. In Python, EOF is represented by an empty string.
Q: How do I read input from stdin in Python?
A: Several methods are available in Python for reading input from stdin, including the input() function, sys.stdin.readline(), and the fileinput module.
Q: How do I handle end of file (EOF) when reading input from stdin?
A: You can handle EOF in Python by detecting an empty string or using a while loop to continue reading input until there is no more data.
Q: What are some best practices for reading input from stdin in Python?
A: Some best practices for reading input from stdin in Python include error handling, input validation, and performance considerations. It is also important to close the file object after reading input.
Q: What are some common issues and problems when reading input from stdin in Python?
A: Some common issues and problems when reading input from stdin in Python include reading too much data, not detecting EOF properly, and encountering unexpected characters or data types.
Q: How can I troubleshoot problems when reading input from stdin in Python?
A: You can troubleshoot problems when reading input from stdin in Python by checking your code for errors, validating input, and using debugging tools or techniques like printing debug statements.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.