Welcome to this guide on using the Python open w+ function to create a file and write content in Python.
If you are new to Python programming or just starting file handling, this article will introduce the open function and its w+ mode.
Whether you’re a developer or a data analyst, understanding how to create files and write content in Python is valuable.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the Python open function
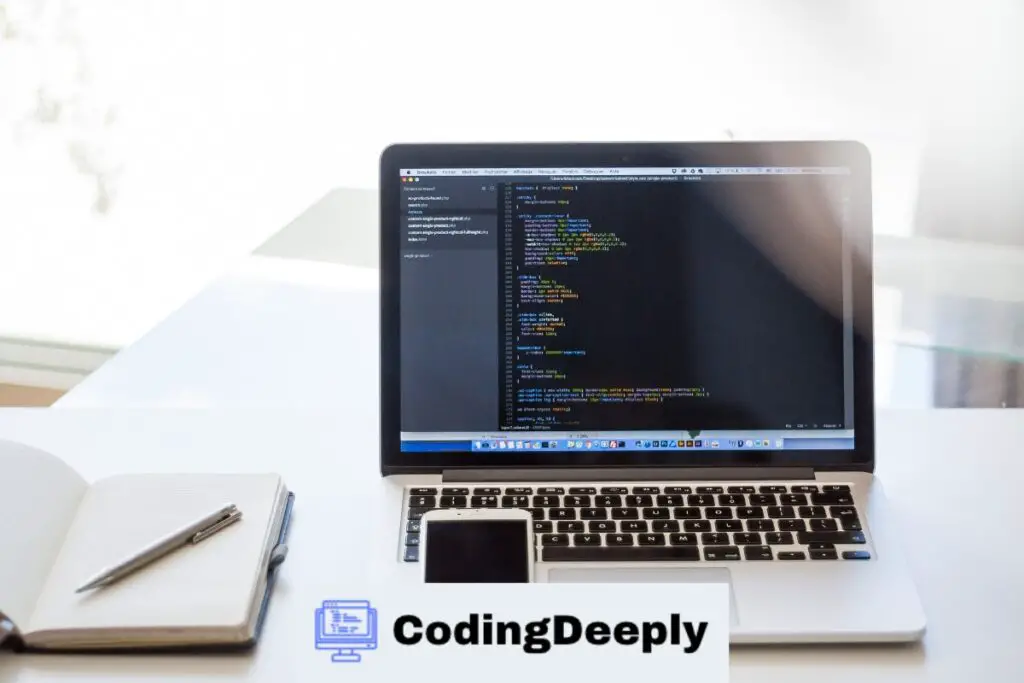
You must use the “open” function to perform file operations in Python. The open function returns a file object that can be used to read from or write to a file.
Here is the basic syntax for opening a file:
file = open(“filename”, “mode”)
The first argument to open is the file name you want to open. The second argument is the mode you want to open the file.
Several modes are available in Python, each providing different access levels to the file. Here are some of the most commonly used modes:
Mode | Description |
---|---|
r | Read-only mode. The file is opened for reading, and the file pointer is placed at the beginning of the file. |
w | Write-only mode. If the file exists, it is truncated to zero length. If the file does not exist, it is created. The file pointer is placed at the beginning of the file. |
a | Write-only mode. If the file exists, the file pointer is placed at the end of the file. If the file does not exist, it is created. |
In addition to these basic modes, some modes allow you to read and write to the same file. These include:
Mode | Description |
---|---|
r+ | Read and write mode. The file is opened for both reading and writing, and the file pointer is placed at the beginning of the file. |
w+ | Read and write mode. If the file exists, it is truncated to zero length. If the file does not exist, it is created. The file pointer is placed at the beginning of the file. |
a+ | Read and write mode. If the file exists, the file pointer is placed at the end of the file. If the file does not exist, it is created. |
Understanding the modes
Your chosen mode will depend on what you want to do with the file. If you only need to read from the file, use “r” mode. If you only need to write to the file, use “w” mode. If you need to read from and write to the file, use “r+” or “w+” mode.
It’s important to note that when you open a file in “w” mode, it is truncated to zero length. This means that any data previously in the file will be deleted. If you want to add data to an existing file, use “a” mode instead.
The w+ mode in Python open function
One of the most useful modes available in the Python open function is the w+ mode, which allows you to write content to a file and read from it.
This mode is particularly handy when creating a new file or modifying an existing one.
When you open a file in w+ mode, the contents of the file are erased immediately. However, you can write new content to the file using various methods such as writing a single line, multiple lines, or even appending to an existing line.
At the same time, you can also read the file’s contents using read or readline functions.
In the following sections, we will explore the w+ mode in more detail, including how to create a file using this mode, how to write content to it, and how to read from it.
Creating a file using the w+ mode
Creating a new file using the w+ mode in Python open function is straightforward.
Here are the steps:
- Open the file using the open() function and specifying ‘w+’ as the mode:
Parameter | Description |
---|---|
filename | The name of the file to be opened. If the file does not exist, it will be created. |
mode | The file mode to be used. In this case, we are using ‘w+’ to open the file for reading and writing. |
Example:
f = open("example.txt", "w+")
- Write content to the file using the write() method:
The write() method allows you to write content to the file. Here’s an example:
f.write("Hello World!")
- Close the file:
It’s important to close the file when you’re done working with it. This ensures that any data you’ve written to the file is saved and that system resources are freed up for other applications.
f.close()
That’s it! You’ve now successfully created a file using the w+ mode in Python.
Writing content to a file using the w+ mode
Now that we have successfully created a file using w+ mode, let’s move on to writing content to it. There are several ways to write content to a file, and we will explore some of the most common methods below:
Method 1: Writing a single line
The simplest way to write content to a file is to use the write()
method to write a single line of text. The syntax for writing a single line is as follows:
Code | Description |
---|---|
file.write("This is a single line") | Writes the string “This is a single line” to the file |
In the above code, we are using the write()
method to write the string “This is a single line” to the file. Note that the write()
method does not automatically add a newline character at the end of the line, so if you want to start the next line on a new line, you will need to add a newline character yourself.
Method 2: Writing multiple lines
If you want to write multiple lines to a file, you can use a loop to iterate through a list of strings and write each string to a new line. Here is an example:
Code | Description |
---|---|
| Writes each string in the lines list to a new line in the file |
In the above code, we are using a for
loop to iterate through the lines
list and write each string to a new line in the file. Note that we are adding a newline character at the end of each line using the \n
escape character.
Method 3: Appending to an existing file
If you want to add new content to an existing file without overwriting the existing content, you can use the append()
mode instead of the write()
mode. Here is an example:
Code | Description |
---|---|
file = open("example.txt", "a") | Opens the file “example.txt” in append mode, writes the string “New line of content” to the end of the file, and closes the file |
In the above code, we are using the open()
function with the "a"
mode to open the file in append mode. This allows us to add new content to the end of the file without overwriting the existing content. Note that we are still using the write()
method to write the new content to the file.
Reading content from a file using the w+ mode
After writing content to a file using the w+ mode in Python, it’s common also to want to read the contents of that file. Fortunately, the w+ mode allows reading and writing, making it a versatile choice for file handling.
To read content from a file opened in w+ mode, you’ll need to ensure that the file pointer is at the beginning of the file. This is because writing operations will move the pointer to the end of the file, so if you attempt to read immediately after writing, you’ll only see the written content.
To move the file pointer to the beginning of the file, you can use the seek()
function. This function takes two arguments: the offset (in bytes) from the beginning of the file, and the position to seek from (which should be set to 0 for the beginning of the file).
Here’s an example:
file = open("example.txt", "w+") file.write("This is some sample text.") file.seek(0, 0) content = file.read() print(content) file.close()
After creating and writing to the file in w+ mode, this code uses seek()
to move the file pointer to the beginning of the file, and then uses read()
to read all of the content in the file. The output of this code would be:
This is some sample text.
It’s important to note that when you’re done reading from a file, you should close it using the close()
function to ensure that any changes you made are saved and the resources used by the file are freed up. If you forget to close the file, you may experience unexpected behavior or errors in your program.
Frequently Asked Questions (FAQ)
What is the difference between the w and w+ modes in Python open function?
The w mode in the Python open function allows for writing to a file but overwrites any existing content.
The w+ mode, on the other hand, allows for both writing and reading to the file, and it does not overwrite existing content. Instead, it adds new content to the end of the file while still allowing access to the existing content.
Can I use the w+ mode to open non-existent files?
Yes, you can use the w+ mode to open non-existent files. If the specified file does not exist, it will be created automatically in this mode.
How do I write multiple lines of content to a file using the w+ mode?
To write multiple lines of content to a file using the w+ mode, you can use the write() function followed by the newline character (\n) to separate each line. For example, you can use the following code to write three lines to a file:
with open('example.txt', 'w+') as file: file.write('Line 1\n') file.write('Line 2\n') file.write('Line 3\n')
How can I read specific lines from a file opened in the w+ mode?
To read specific lines from a file opened in the w+ mode, you can use the seek() function to set the file pointer to the beginning of the desired line, and then use the readline() function to read the content of that line. For example, you can use the following code to read the third line of a file:
with open('example.txt', 'w+') as file: file.write('Line 1\n') file.write('Line 2\n') file.write('Line 3\n') file.seek(0) third_line = file.readline()
Can I use the w+ mode to append content to a file instead of writing to the end?
No, the w+ mode always writes to the end of the file, regardless of the file pointer’s position. If you want to append content to a file, you should use the a or a+ modes instead.
How can I ensure that my file is properly closed after using the w+ mode in Python?
To ensure that your file is properly closed after using the w+ mode in Python, you should use the with statement to open and close the file. This will automatically close the file when the code block is finished executing, even if an exception is raised. For example:
with open('example.txt', 'w+') as file: file.write('Some content') # file is automatically closed outside of the with block
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.