Hey there! Have you ever encountered an error message in Python that says “NoneType object has no attribute”?
If you have, don’t worry, you’re not alone! This error message can be quite confusing and frustrating, especially for beginners in programming.
But fear not, in this article, we’ll explore what the NoneType object is, what the AttributeError means, and how to troubleshoot and fix it.
Understanding the error message is crucial because it can help you pinpoint the problem in your code and make the necessary adjustments.
So, let’s dive in and explore this error message in more detail.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
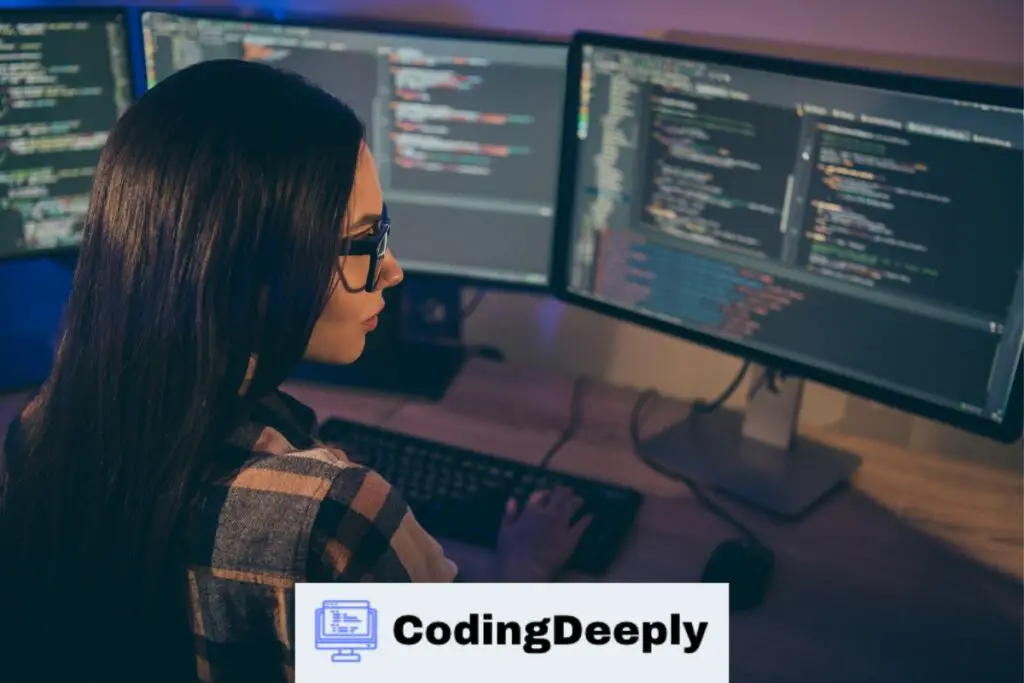
- The AttributeError is raised when you try to access a method or attribute of an object that does not exist in Python.
- The NoneType object is commonly associated with the AttributeError.
- Double-checking the attribute name, checking that the object is the correct type, and using a conditional statement to check if the object has the attribute before accessing it are some tips for troubleshooting the AttributeError.
- Using the documentation or source code of the object to ensure that the attribute exists and is being used correctly can help fix the AttributeError.
- Troubleshooting and fixing errors like the AttributeError is an essential part of programming in Python.
What is the NoneType object?
So, what exactly is the NoneType object? In Python, None is a built-in constant that represents the absence of a value. The NoneType object is the data type of the None value.
Here are a few things to keep in mind about the NoneType object and None value:
- None is commonly used to indicate the absence of a value or the end of a sequence.
- None is a singleton object in Python, which means that there is only one instance of it.
- The NoneType object is a special type in Python that represents the type of the None value.
- None is often used as a default value for function arguments or variables that may not have a value initially.
In Python, the None value can be assigned to any variable, but it is most commonly used in functions and conditional statements. Now that we have a better understanding of the NoneType object, let’s explore the AttributeError in more detail.
Understanding the AttributeError
The AttributeError is an error message that you might encounter when working with Python. This error message is raised when an object does not have the attribute that you are trying to access. In other words, the AttributeError is raised when you try to access a method or attribute of an object that does not exist.
Some common causes of the AttributeError include:
- A typo in the attribute name
- The object is not the type you expected it to be
- The object has not been initialized correctly
- The object does not have the attribute you are trying to access
Here are a few examples of code that could raise an AttributeError:
Example 1:
my_list = [1, 2, 3]
my_list.append(4)
my_list.sort()
# This will not raise an AttributeError because my_list is a list object and it has the methods 'append' and 'sort'
Example 2:
my_string = "hello"
my_string.append("world")
# This will raise an AttributeError because the string object does not have the 'append' method.
Example 3:
my_dict = {"key": "value"}
my_dict.popitem()
my_dict.sort()
# This will raise an AttributeError because the dictionary object does not have the 'sort' method.
Now that we have a better understanding of what causes the AttributeError, let’s explore some strategies for troubleshooting and fixing it.
Troubleshooting AttributeError
Encountering an AttributeError can be frustrating, but there are several tips and strategies you can use to troubleshoot and fix the error. Here are a few:
Tips for debugging AttributeError
- Double-check the attribute name for typos or errors.
- Check that the object you are trying to access the attribute from is the correct type.
- Make sure that the object has been initialized correctly and that the attribute exists.
Common strategies for fixing AttributeError
- Use a conditional statement to check if the object has the attribute before accessing it.
- Check the documentation or source code of the object to ensure that the attribute exists and is being used correctly.
- Reinitialize the object to ensure that it has the correct attributes.
Here are a few examples of how you could fix an AttributeError:
Example 1:
my_string = "hello"
if hasattr(my_string, "append"):
my_string.append("world")
# This will not raise an AttributeError because the conditional statement checks if the string object has the 'append' method before accessing it.
Example 2:
my_dict = {"key": "value"}
if hasattr(my_dict, "sort"):
my_dict["key2"] = "value2"
sorted_dict = dict(sorted(my_dict.items()))
# This will not raise an AttributeError because the conditional statement checks if the dictionary object has the 'sort' method before trying to use it. If it doesn't have the method, the code inside the conditional statement will not be executed.
Example 3:
my_list = [3, 2, 1]
my_list.sort()
# This will fix the AttributeError because we are calling the 'sort' method on a list object, which has the 'sort' method.
Remember, troubleshooting and fixing errors like AttributeError is an essential part of programming, so don’t get discouraged if you encounter them!
Conclusion
In this article, we’ve explored the AttributeError in Python, which is raised when an object does not have the attribute you are trying to access. We’ve defined the NoneType object, which is commonly associated with the AttributeError, and provided examples of code that could raise this error. We also discussed tips for debugging AttributeError and common strategies for fixing it.
To recap, here are some key points to remember about handling AttributeError in Python:
- The AttributeError is raised when you try to access a method or attribute of an object that does not exist.
- Some common causes of the AttributeError include typos in the attribute name, incorrect object initialization, or the object not being the type you expected it to be.
- Tips for troubleshooting and fixing the AttributeError include double-checking the attribute name, checking that the object is the correct type, and using a conditional statement to check if the object has the attribute before accessing it.
In conclusion, encountering errors like the AttributeError is a common part of programming. By using the strategies and tips we’ve discussed in this article, you can effectively troubleshoot and fix these errors to create more robust and efficient code in Python.
Frequently Asked Questions
What can cause the AttributeError to occur in Python?
The AttributeError can occur when you try to access a method or attribute of an object that does not exist. Some common causes of this error are typos in the attribute name, incorrect initialization of the object, or the object is not the expected type.
How can I fix the AttributeError in my Python code?
To fix the AttributeError, you can try to fix the problem by checking the attribute name, checking the object type, and using a conditional statement to verify that the object has the attribute before accessing it.
Additionally, using the object’s documentation or source code can be helpful to ensure that the attribute exists and is being used correctly.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.