Welcome to this guide on capturing user input with the enter key in Python. Accepting user input is crucial for creating interactive programs, and capturing input with the enter key is a common method.
In this guide, we will cover the basics of capturing user input with the enter key and more advanced techniques for manipulating and processing user input.
We will also discuss handling user input errors and implementing input validation. By the end of this guide, you will have a solid understanding of capturing and processing user input in your Python programs.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding User Input in Python
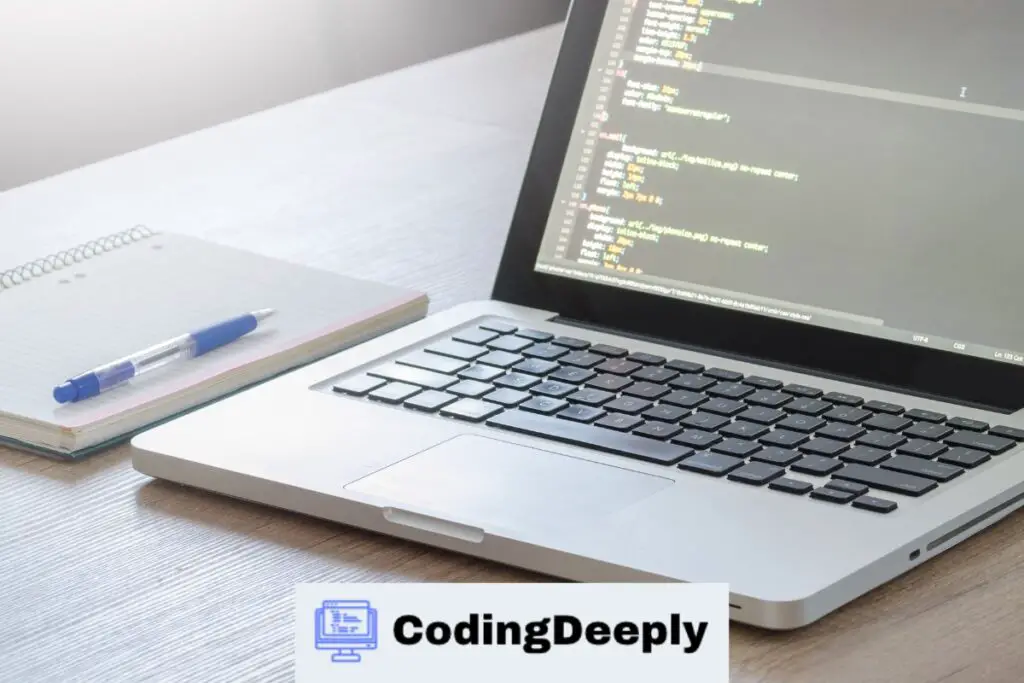
Python is a popular programming language that is widely used for developing all kinds of applications. One of the key features of Python is its ability to accept input from users.
User input can be defined as any data that a user enters into a program through the keyboard, mouse, or other input devices.
This section will discuss the basics of accepting user input in Python and explore the different methods available.
How User Input Works in Python
In Python, user input is typically accepted using the input()
function. This function prompts the user to enter a value, which is then stored in a variable.
The input()
function waits for the user to enter input and press the Enter key. Once the user has entered their input, the function returns the input as a string.
For example, suppose we want to prompt the user to enter their name and store it in a variable called name
. We can use the following code:
name = input("Please enter your name: ")
print("Hello, " + name + "!")
When this code is executed, the user will see the message “Please enter your name:” in the console. They can then enter their name and press Enter. The program will then print the message “Hello, [name]!” where [name] is the name that the user entered.
Other Methods for Accepting User Input
While the input()
function is the most common way to accept user input in Python, there are other methods available.
For example, the sys.stdin.readline()
function can be used to read a line of input from the console. This function is useful when you need to read input that contains spaces or special characters.
In addition, Python provides several libraries that can be used to accept input from sources other than the console. For example, the tkinter
library can be used to create graphical user interfaces that allow users to enter data using text boxes, buttons, and other widgets.
Now that we’ve covered the basics of user input in Python, let’s move on to capturing user input specifically with the enter key.
Capturing User Input with the Enter Key
One of the most common methods for capturing user input in Python is through the use of the `input()` function. This function allows the program to display a prompt to the user and wait for them to enter input.
To capture user input specifically with the enter key, the `input()` function can be used in conjunction with a while loop. The loop will prompt the user for input until the enter key is pressed.
Capturing Input with While Loop
Here’s an example of how to use a while loop to capture user input with the enter key:
while True: user_input = input("Please enter some text and press Enter to submit: ") if user_input: break
In this example, the `while True` loop ensures that the program will continuously prompt the user for input until it receives input and the `break` statement is executed.
The `input()` function displays a prompt message to the user to enter text and waits for the user to enter input. If the user enters input and presses the enter key, the string is assigned to the `user_input` variable and the loop is exited through the `break` statement.
If the user doesn’t enter anything and hits enter, the loop will continue to prompt for input until it receives valid input.
It’s important to note that the `input()` function automatically handles the enter key press as an input string, so there’s no need to capture the enter key press separately explicitly.
Handling User Input with Conditionals
Once user input has been captured, it can be processed further and used to perform various tasks. One of the most common ways to process user input is through conditional statements that perform different actions based on the user input.
Here’s an example:
user_input = input("Please enter 'yes' or 'no' and press Enter: ") if user_input.lower() == "yes": print("You entered 'yes'.") elif user_input.lower() == "no": print("You entered 'no'.") else: print("Invalid input.")
In this example, the program prompts the user to enter “yes” or “no” and waits for input. Once input is entered and the enter key is pressed, the input is assigned to the `user_input` variable.
The program then checks if the user entered “yes” or “no” by using an if/else statement with the `lower()` method. If the user entered “yes”, the program prints “You entered ‘yes’.” If the user entered “no”, the program prints “You entered ‘no’.”
If the user entered anything other than “yes” or “no”, the program prints “Invalid input.”
Using basic conditional statements and user input capture techniques makes it easy to create interactive Python programs that accept and process user input effectively.
Handling User Input Errors
When capturing user input in Python, handling errors is a critical part of the process. Without proper error handling, your program may crash or produce unexpected results.
Input Validation
One technique for handling user input errors is input validation. Input validation involves checking user input to make sure it meets certain criteria.
Empty Input: Using the if statement, you can check if the user has entered any input. For example:
if len(input_string) == 0:
print(“Please enter a value”)
Input Type: You can also validate the type of input by using built-in Python functions. For example, you can use isdigit() to check if the input is a number:
if input_string.isdigit():
number = int(input_string)
else:
print(“Please enter a valid number”)
Error Handling
In addition to input validation, you can also use error handling to catch potential errors and prevent your program from crashing. Python provides a try/except statement for this purpose.
For example, if you are converting user input to a number, you can use a try/except statement to catch any errors that may occur:
try:
number = int(input_string)
except ValueError:
print(“Please enter a valid number”)
By using input validation and error handling, you can ensure that your program is robust and able to handle a variety of user input scenarios.
Processing User Input
Now that we have captured user input with the enter key using the `input()` function, we need to process that input in order to make use of it in our program. Here are some common tasks you may want to perform with user input:
Converting Input to Different Data Types
The user’s input is typically read as a string, but you may need to convert it to a different data type, such as an integer or float, in order to perform calculations or comparisons.
To do this, you can cast the input to the desired data type using the appropriate function:
- Use the `int()` function to convert a string to an integer.
- To convert a string to a floating-point number, use the `float()` function.
- If you’re not sure whether the input can be converted to the desired data type, you can use a `try-except` block to catch any errors that may occur.
Performing Calculations
Once you have converted user input to the desired data type, you can perform calculations on it. Here are some common operators you can use:
- Addition: `+`
- Subtraction: `-`
- Multiplication: `*`
- Division: `/`
- Modulus (remainder): `%`
For example, if you want to add two numbers entered by the user, you could write:
num1 = int(input(“Enter the first number: “))
num2 = int(input(“Enter the second number: “))
result = num1 + num2
print(“The sum is:”, result)
Storing Input in Variables
Once you have processed user input, you may want to store it in a variable for later use. To do this, assign the input to a variable using the `=` operator:
name = input(“What is your name? “)
age = int(input(“What is your age? “))
print(“Your name is”, name, “and you are”, age, “years old.”)
Now you can refer to the `name` and `age` variables later in your program to use the values entered by the user.
Advanced Techniques for User Input
While the basics of capturing and processing user input are essential, there are also more advanced techniques that you can use to manipulate and validate input in Python. Here are some examples:
1. Input Validation Using Regular Expressions
One powerful technique for validating user input is to use regular expressions. This allows you to define specific patterns that the input must match in order to be considered valid. For example, you can use regular expressions to validate email addresses, phone numbers, or credit card numbers.
To use regular expressions in Python, you will need to import the re module. You can then define the regular expression pattern using the re.compile() function and apply it to the input string using the re.match() function.
2. Handling Special Characters
When capturing user input, it’s important to consider how special characters like quotes or backslashes will be handled. One way to do this is by using escape characters, such as \, to represent these characters in the input string.
Another option is to use raw string literals created by adding an r before the opening quotation mark. This tells Python to ignore all escape characters in the string.
3. Implementing Input Prompts
Input prompts are a useful way to guide the user and provide context for the expected input. You can create input prompts using the input() function by including a string argument that describes the input expected from the user.
For example, if you were asking the user to enter their name, you could use the following code:
name = input("Please enter your name: ")
4. Creating Dynamic Prompts
Another advanced technique for user input is to create dynamic input prompts that change based on the user’s previous input. This can be useful for guiding the user towards valid input or for providing additional context based on their previous responses.
To create dynamic input prompts, you can use conditional statements in your code that determine what input prompt to display based on the user’s previous input. You can also use loops to repeatedly ask for input until the user provides valid input.
By using these advanced techniques for manipulating and processing user input, you can create more robust and user-friendly applications in Python.
Capturing User Input with the Enter Key
When capturing user input in Python, the enter key can be useful. The input()
function is the most common method used to capture user input, and it waits for the user to press the enter key before returning the input value.
Using the input()
Function
The input()
function is used to capture user input in Python, and it can be enhanced to capture input specifically using the enter key. Here’s an example:
user_input = input("Please enter your name and press Enter: ")
This code prompts the user to enter their name, and once they press the enter key, the input value is stored in the user_input
variable.
Handling the Enter Key
When using the input()
function, it’s important to handle the enter key to ensure proper capture of user input. Here’s an example:
user_input = input("Please enter your age and press Enter: ")
if user_input == "":
print("You must enter a value for your age.")
else:
age = int(user_input)
print("You entered", age)
This code prompts the user to enter their age, and if they press enter without providing a value, the script will inform them that a value is required. If a value is provided, it will be stored as an integer variable.
Summary
The enter key can be useful when capturing user input in Python, and the input()
function makes it easy to do so.
By handling the enter key and implementing input validation, you can create robust programs that accurately capture and process user input.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.