Welcome to our article on sharing global variables across files in Python. As you develop more complex programs, you may need to share data between different modules in your code.
This is where global variables come in handy.
This section will introduce you to global variables and how they can facilitate seamless communication between files in your Python code. We will explore the benefits of using global variables and how they can help simplify your code and avoid redundancy.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Global Variables in Python
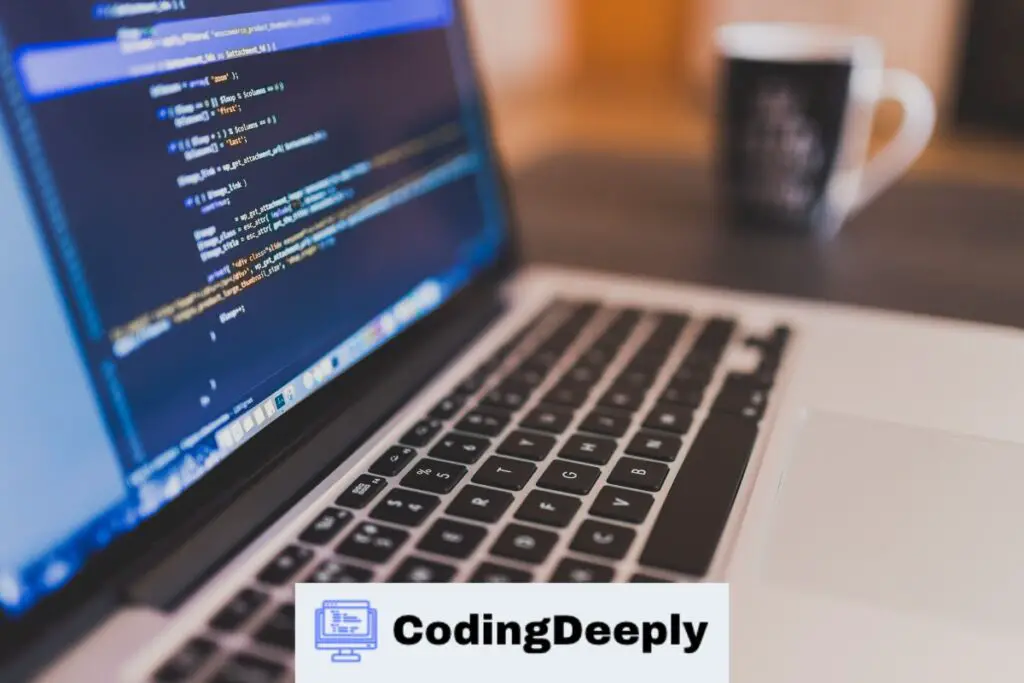
Before we dive into the techniques for sharing global variables across files, let us first understand what global variables are in Python and how they differ from local variables.
In Python, a variable is defined as global if declared outside of a function or class. Global variables can be accessed and modified from any part of the code, making them useful for sharing data between different parts of your program.
However, when working with global variables, it is important to remember that they are accessible from all files and functions within your code. This can lead to conflicts and errors if not used carefully.
One way to mitigate these issues is by using the Python global keyword. When declaring a global variable inside a function, specify it using the global keyword. This tells Python that the variable is global and should be accessed from outside the function.
Here’s an example to illustrate how the global keyword works:
x = 10 # global variable def foo(): global x # declare x as global inside the function x = 5 # modify the global variable foo() print(x) # Output: 5
In the example above, we declare the variable x as global inside the function foo using the global keyword. This allows us to modify the global variable x from within the function and update its value to 5. The output of the print statement confirms that the value of x has been modified globally.
Sharing Global Variables Across Multiple Files
Sharing global variables across multiple files is essential to building complex software applications in Python. When two or more modules need to communicate and share data, global variables can provide a clean and concise solution.
This section will explore different techniques for sharing global variables across files and ensuring smooth communication between modules.
Using Python Modules
One way to share global variables between files is by defining them in one module and accessing them in another module.
This is done by creating a Python module that contains the global variables and using the import
statement to access them in a different module.
Example:
file1.py |
x = 5
|
---|---|
file2.py |
import file1
print(file1.x)
|
In this example, we define a global variable x
in file1.py
and import it into file2.py
using the import
statement. We can then access the value of x
using file1.x
in file2.py
.
Importing Variables
Another way to share global variables between files is by importing specific variables from a module using the from
statement. This allows you to access only the variables you need from a module instead of importing the entire module.
Example:
file1.py x = 5
file2.py from file1 import x
print(x)
In this example, we import the global variable
x
fromfile1.py
intofile2.py
using thefrom
statement. We can then access the value ofx
directly infile2.py
.
It is important to note that global variables can introduce potential risks and challenges in your code. To ensure a smooth and maintainable codebase when sharing global variables, it is essential to follow best practices and guidelines, which we will discuss in the next section.
Best Practices for Sharing Global Variables
While global variables can be useful for communicating between files in your Python code, they can also introduce potential risks and challenges.
Here are some best practices and guidelines to ensure smooth communication and avoid common pitfalls when sharing global variables between modules:
- Avoid overusing global variables: While global variables can simplify communication between modules, excessive use can lead to a lack of clarity and organization in your code. Consider carefully whether a global variable is necessary before implementing it.
- Define global variables in a centralized location: To avoid conflicts or confusion, define your global variables in a separate module dedicated to holding global values. This will make it easier to manage and update them in one place.
- Use appropriate naming conventions: Choose descriptive and unique names for your global variables to avoid naming collisions. Avoid using common or reserved names in Python to prevent unexpected behavior.
- Handle global variables with care: Be cautious when modifying or accessing global variables, especially in a multi-threaded environment, to prevent race conditions or unexpected behavior. Consider using synchronization mechanisms such as locks or semaphores to avoid concurrency issues.
By following these best practices, you can ensure that your use of global variables in Python is clear, organized, and reliable.
FAQ: Common Questions About Global Variables in Python
In this section, we will address some frequently asked questions related to global variables in Python. These FAQs will cover common concerns and provide additional clarity on the topic of sharing data between modules using global variables.
What are the potential risks of using global variables?
Global variables can introduce potential risks in your code, such as naming conflicts, unexpected side effects, and difficulty in debugging. It is important to use global variables with caution and only when necessary.
Can global variables be used across different Python versions?
Yes, global variables can be used across different Python versions as long as the syntax and naming conventions of the code remain the same.
How can I avoid naming conflicts when using global variables?
To avoid naming conflicts, it is recommended to use a naming convention that clearly identifies the purpose and scope of each variable. Additionally, you can use a unique prefix or suffix for each variable to ensure that they do not clash with other variables in your code.
Is it possible to change the value of a global variable in one module and have it reflected in another module?
Yes, it is possible to change the value of a global variable in one module and have it reflected in another module, as long as both modules are accessing the same global variable. However, it is important to use caution when modifying global variables and ensure that the changes do not cause unexpected side effects in other parts of your code.
What is the difference between local and global variables?
Local variables are defined within a function or block of code and can only be accessed within that function or block. Global variables, on the other hand, are defined outside of any function or block and can be accessed from anywhere in the code, including within functions and modules.
When is it appropriate to use global variables?
It is appropriate to use global variables when you need to share data between different parts of your code, and passing the data as arguments or returning values is not practical or efficient.
However, using global variables sparingly and only when necessary is important, as they can introduce complexity and potential risks in your code.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.