Are you experiencing issues with Python files closing immediately? This problem can be frustrating and hinder your productivity. However, there are several solutions to this problem that we can explore.
In this section, we will guide you through common causes of Python file closure issues and provide troubleshooting steps to fix them. We’ve got you covered whether it’s due to coding errors, system resources, or runtime errors.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding the issue
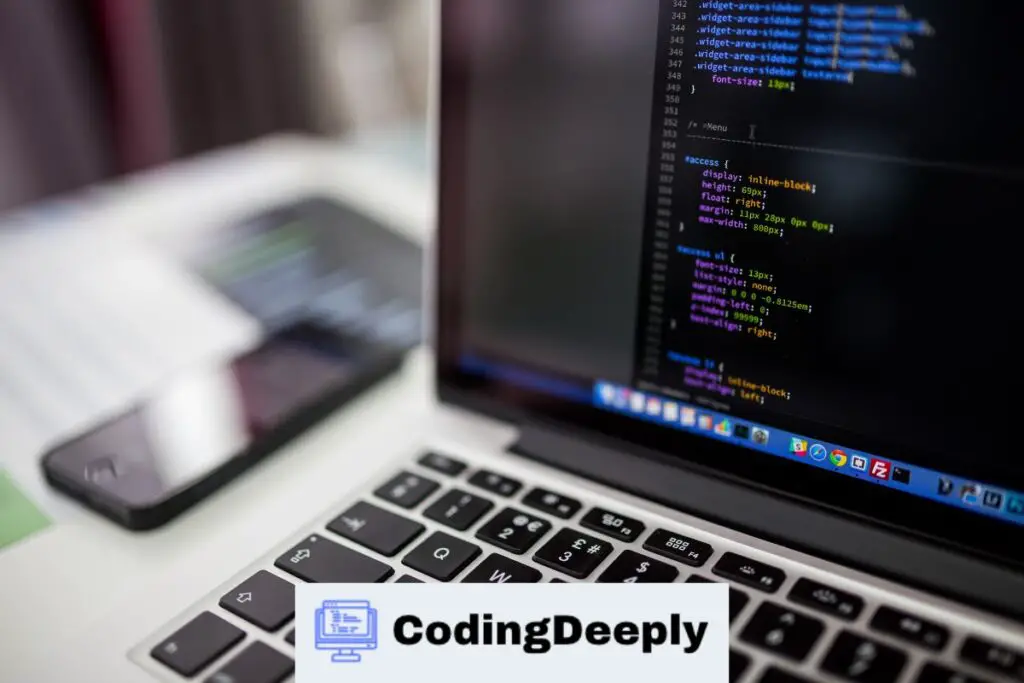
Before diving into the solutions, it’s important to understand why Python files may close immediately.
Various factors can cause this issue.
- Errors in the code: Python files may close abruptly if there are errors in the code that cause the program to terminate unexpectedly.
- Inadequate system resources: If the system does not meet the requirements of the program, it can lead to immediate closure of the file.
- Runtime errors: Some errors may happen during the execution of the program that can cause the file to close without warning.
- External factors: Antivirus software, firewalls, or other software can interfere with the execution of Python files, leading to unexpected closures.
By understanding these potential causes, you can troubleshoot your Python files more effectively.
Check for Errors in the Code
Errors in the code can be a common cause for Python files closing immediately. Debugging your code will help identify and eliminate any errors that may be causing your files to close unexpectedly.
Identifying Errors
When your Python file closes immediately, there is likely an error in your code. The error could be related to syntax, logical errors, or runtime errors. Use a debugger to identify and locate the errors in your code.
Several debugging tools are available for Python, including pdb and PyCharm. These tools enable you to review your code line-by-line, inspect variables and data structures, and identify syntax and runtime errors.
Fixing Errors
Once you have identified the errors in your code, the next step is to fix them. Depending on the type and complexity of the error, there may be several approaches to fixing it.
- Fix syntax errors: Syntax errors can typically be fixed by correcting the syntax. Review your code to identify any missing or misplaced brackets, parentheses, or quotation marks.
- Fix logical errors: Logical errors are often caused by incorrect logic or algorithm. Carefully analyze your code for logical errors and consider alternative approaches to resolve them.
- Handle runtime errors: Runtime errors can be handled using exception handling techniques. Use try-except blocks to handle exceptions and prevent your Python file from closing immediately.
After fixing the errors, re-run your Python file to ensure it runs without closing immediately.
Verify system resources
Another possible cause for Python files closing immediately is inadequate system resources. Checking and optimizing system resources is important to ensure smooth execution of your Python files. Below are some steps you can take:
Check CPU and memory usage
Check your computer’s CPU and memory usage while running the Python file. If your system usage is high, try closing other applications that are not essential to running the Python file.
Update drivers
Ensure that all your drivers are up to date. Outdated drivers can cause system instability, resulting in abrupt file closures.
Allocate more memory
Python files can require a significant amount of memory to run smoothly. If your file crashes due to lack of memory, try allocating more memory to your Python environment or optimizing your code to reduce memory usage.
Check your system requirements
Ensure that your system meets the minimum requirements for running your version of Python. Check the Python documentation for system requirements.
Disable antivirus and firewalls temporarily
Antivirus software and firewalls can sometimes interfere with the execution of Python files. Temporarily disabling them can help to determine whether they are causing the issue. If they are, try adding exceptions or disabling specific settings that may be causing conflicts.
Handling runtime errors
Runtime errors can be frustrating and lead to unexpected closures of Python files. However, there are techniques for handling them and allowing your code to continue running.
Exception handling
One approach to handling runtime errors is through the use of exception handling. This involves anticipating potential errors and writing code to handle them gracefully, rather than letting the program crash.
To use exception handling, you must wrap the code that may cause an error in a try-except block. The try block contains the code that may raise an exception, while the except block contains the code that handles the exception. Here’s an example:
try: # code that may raise an exception except: # code that handles the exception
You can also specify the type of exception to handle by including the name of the exception after except. For example:
try: # code that may raise a NameError exception except NameError: # code that handles the NameError exception
Logging
Another useful technique for handling runtime errors is logging. Logging allows you to track the execution of your program and record any errors that occur. This can be especially useful for identifying and fixing bugs that may be causing your Python files to close unexpectedly.
To use logging, you can import the logging module and then use the various logging functions to write messages to a log file. Here’s an example:
import logging logging.basicConfig(filename='example.log', level=logging.DEBUG) try: # code that may raise an exception except Exception as e: logging.error(e)
This code sets up basic logging to a file named example.log and sets the logging level to DEBUG. Then, any exceptions that occur within the try block will be logged to the file using the logging.error function.
By using these techniques, you can handle runtime errors in your Python files and prevent them from closing unexpectedly.
Updating Python and dependencies
If your Python files are still closing immediately after following the troubleshooting steps in the previous sections, it’s possible that outdated Python versions or dependencies are the root cause. It’s important to keep your Python environment up to date to ensure smooth execution of your scripts. Follow the steps below to update your Python and dependencies.
Updating Python
To check your current Python version, open a command prompt or terminal window and type:
- python –version
If your version is outdated, you can download the latest version of Python from the official website and install it.
Alternatively, if you’re using a Python version manager, such as pyenv or Anaconda, you can use the manager to update your Python version.
Updating dependencies
Dependencies are external packages that your Python script relies on. It’s important to keep your dependencies up to date to ensure your Python environment runs smoothly. To update your dependencies, follow the steps below:
- Identify the dependencies used in your project and their versions by analyzing your requirements.txt file or by running pip freeze command in command prompt or terminal:
- pip freeze > requirements.txt
- Update individual dependencies by running:
- pip install –upgrade [dependency_name]
- To update all dependencies at once, run:
- pip install –upgrade -r requirements.txt
Following these steps should help ensure that your Python files run smoothly and don’t close immediately due to outdated Python versions or dependencies.
Troubleshooting External Factors
Even with proper coding practices and system resources, external factors can still interfere with the execution of Python files and lead to unexpected closures. Let’s explore potential conflicts and provide troubleshooting steps to resolve them.
Antivirus Software
Antivirus software can sometimes mistake Python scripts for malicious software and prevent them from running correctly. To resolve this, try temporarily disabling your antivirus software and re-run the script. If the issue is resolved, you may need to add your Python directory or script as an exception to your antivirus software.
Firewalls
Firewalls can also interfere with the execution of Python scripts. If you suspect the firewall is causing issues, try temporarily disabling it and re-run the script. If the script runs correctly, you may need to add a rule to your firewall allowing Python scripts to run. Consult your firewall’s documentation for instructions on how to do this.
Permissions
Sometimes, user permissions can prevent Python scripts from running correctly. Make sure the user running the script has appropriate permissions to access the directory and files required for the script.
Other Programs
Other programs running on your computer can sometimes interfere with the execution of Python scripts. Try closing unnecessary programs and restarting your computer before running the script again.
With these troubleshooting techniques, you should be able to identify and resolve external factors causing unexpected closures of your Python files.
Preventing future closure issues
While troubleshooting and fixing the immediate closure of Python files is critical, it’s also important to take steps to prevent this issue from reoccurring in the future. Here are some best practices for file management:
- Organize files: Keep your files organized in a clear and logical directory structure. This will make it easier to find and manage your files.
- Use version control: Version control software like Git can help you keep track of changes to your code and revert to previous versions if necessary.
- Check for compatibility: Before integrating new code or libraries, make sure they are compatible with your existing code.
- Test code: Before running code on a large scale, test it with a small dataset to check for errors and ensure that it runs smoothly.
- Use exception handling: Implement exception handling in your code to handle runtime errors in a controlled manner, preventing abrupt closures.
- Maintain dependencies: Keep your Python and dependencies up to date. Outdated versions can cause compatibility issues and unexpected closures.
By following these best practices, you can minimize the risk of immediate closure of Python files and maintain a more stable and efficient coding environment.
FAQ
Here are some frequently asked questions about Python file closure:
Q: Why does my Python file keep closing immediately?
A: There can be several reasons why your Python file is closing immediately, including errors in the code, inadequate system resources, runtime errors, outdated Python versions or dependencies, and external factors like antivirus software or firewalls.
Q: How can I troubleshoot my Python file?
A: To troubleshoot your Python file, you can try several steps, including checking for coding errors, verifying system resources, handling runtime errors using exception handling techniques, updating Python and dependencies to the latest versions, and troubleshooting external factors.
Q: How can I prevent my Python file from closing unexpectedly in the future?
A: To prevent future instances of Python files closing immediately, you can follow file management best practices, such as organizing and structuring your files effectively. It’s also essential to stay up to date with the latest versions of Python and dependencies.
Q: Can antivirus software or firewalls interfere with the execution of Python files?
A: Yes, external factors like antivirus software or firewalls can interfere with the execution of Python files and lead to unexpected closures. If you suspect that such factors are causing the issue, you can troubleshoot by modifying your antivirus or firewall settings, or disabling them temporarily to see if the Python file runs smoothly.
Q: Why is it important to keep Python and dependencies up to date?
A: Updating to the latest Python and dependency versions can fix known bugs and security vulnerabilities, improve performance, and provide access to new features and functionalities. By keeping your software up to date, you can avoid many potential issues like files closing abruptly.
Q: What is exception handling?
A: Exception handling is a technique used in software development to handle runtime errors that could cause a program or file to crash. This technique allows the software to continue to run when an error occurs, instead of shutting down abruptly, by catching and handling the error gracefully.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.