Eval vs. exec: These two built-in functions are essential for executing code dynamically in Python, but it’s crucial to understand their differences to use them effectively.
This article explores the key features and distinctions between eval and exec, highlighting their usage and limitations.
Whether you’re a novice Python coder or an experienced developer, this article will provide valuable insights into using eval and exec more efficiently in your programs. So, let’s begin our journey into Python eval and exec!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
What is Python eval?
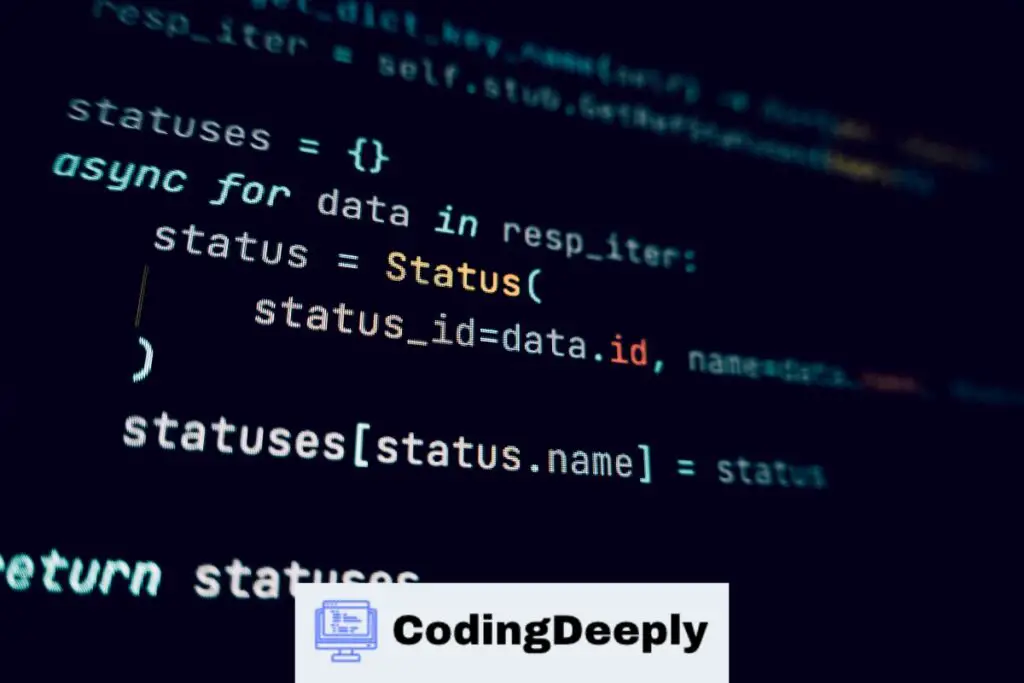
Python eval is a built-in function in the Python language that allows you to execute a single Python expression or statement dynamically. This means that you can use eval to run code that has been dynamically generated or created at runtime.
The syntax for using eval is quite simple. All you need to do is pass a string containing the Python expression or statement you want to execute to the eval function. The function will then evaluate the expression or statement and return the result.
Here is an example:
Code: | x = 3 |
---|---|
Output: | 5 |
In this example, we define a variable x
and set its value to 3
. We then use eval to execute the expression 'x + 2'
, which adds 2
to the value of x
and returns the result 5
. Finally, we print the result to the console.
It is important to note that while eval can be a powerful tool, it can also be risky to use in certain situations. We will discuss these considerations in more detail in later sections of this article.
What is Python exec?
Python exec is a built-in function in Python that is used to execute a block of dynamically created code. Unlike Python eval, which can only evaluate a single expression, Python exec can execute more complex code blocks, such as loops and conditional statements.
This makes it a powerful tool for dynamic execution of Python code, particularly in cases where the code is generated programmatically.
Python exec takes a single argument, a string containing one or more lines of Python code.
It then compiles and executes the code contained in the string, within the program’s current global and local namespace. This allows the executed code to interact with and modify the program’s current state.
It is important to note that while Python exec can be a useful tool, it can also pose a security risk if used improperly. Since it can execute arbitrary code, it is important to ensure that the code being executed is trusted and does not allow for unauthorized access or manipulation of sensitive data.
Key Differences between eval and exec
Python eval and exec are two functions that allow for dynamic execution of code. While they may seem similar, there are several key differences between the two:
Function | Differences |
---|---|
eval | – Evaluates a single expression or statement – Returns the result of the evaluation – Can only evaluate one expression or statement at a time – Cannot modify the environment in which it is called – Should be used for simple and safe evaluations |
exec | – Executes a block of code as a whole – Does not return anything – Can execute multiple expressions and statements – Can modify the environment in which it is called – Should be used with caution due to potential security risks |
One important distinction to note is that eval evaluates a single expression or statement, while exec executes an entire block of code. Additionally, eval cannot modify the environment in which it is called, whereas exec has the ability to make changes to the environment.
Another consideration is the potential security risks associated with using exec. Since it can execute multiple expressions and statements and modify the environment, it can potentially cause significant harm if used improperly. As a result, it should be used with caution and only in cases where its usage is necessary or advantageous.
Understanding the differences between eval and exec is crucial for selecting the appropriate function for different programming tasks, ensuring that code is executed safely and effectively.
When to use eval and when to use exec
Choosing between eval and exec can depend on multiple factors, including the code requirements, security concerns and performance considerations. Here are some guidelines to help you determine when to use eval and when to use exec:
When to use eval
Eval is best used for evaluating and executing single expressions or statements that are relatively simple. It is particularly useful for working with user input or dynamically generated code.
However, it is important to note that eval can pose a security risk, as it can execute arbitrary code.
As such, it is recommended that it be used only with trusted input.
When to use exec
Exec is a more powerful function than eval, as it can execute blocks of code with multiple statements or complex expressions. It is ideal for handling larger, more complex scripts.
However, it is also a potential source of security vulnerabilities, especially when untrusted code is being executed. As such, it is recommended that exec be used with caution and only when necessary.
When deciding whether to use eval or exec, it is important to consider the specific requirements of the code and to evaluate any potential security or performance concerns thoroughly.
In general, it is a good practice to avoid using either function unless absolutely necessary and to limit their use to trusted or controlled input.
FAQ
Here are some frequently asked questions about Python eval and exec:
1. What is the difference between Python eval and exec?
Python eval is used to execute a single expression or statement dynamically, while Python exec is used to execute larger blocks of code dynamically. Eval returns a value, while exec doesn’t return anything.
2. Is it safe to use eval and exec?
Both eval and exec can be potentially dangerous if used improperly. It’s important to be careful when using them to avoid any security issues. It’s recommended to only use them with trusted code and to avoid user input whenever possible.
3. When should I use eval?
Eval should be used when you want to evaluate and execute a single expression or statement. It’s useful for quick computations or for evaluating user input that you trust.
4. When should I use exec?
Exec should be used when you need to execute larger blocks of code dynamically. It’s useful for creating dynamic code, for example, when constructing functions or classes on-the-fly.
5. Can eval or exec improve the performance of my program?
Using eval or exec can be faster than writing equivalent code using normal Python syntax, but it’s important to consider the potential security risks and to use them sparingly. In general, it’s better to write code using standard Python syntax whenever possible.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.