Unlock the power of Python dictionaries with integer keys right from the start. This guide will save you from common pitfalls, streamline your coding process, and boost your programming efficiency.
You’ll discover:
- Theoretical foundations of Python dictionaries
- Practical examples with insightful tips
- Solutions to potential challenges
Dive in to elevate your Python skills and become a more proficient programmer.
You will want to see what’s coming next!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
- Python dictionaries with integer keys are efficient, simple, and flexible.
- Keys in Python dictionaries are unique and can be almost any data type.
- You can add, modify, and delete key-value pairs in Python dictionaries.
- Common mistakes include using mutable data types as keys and assuming dictionaries are ordered.
- Troubleshooting involves handling KeyError and TypeError exceptions.
Theoretical Foundations
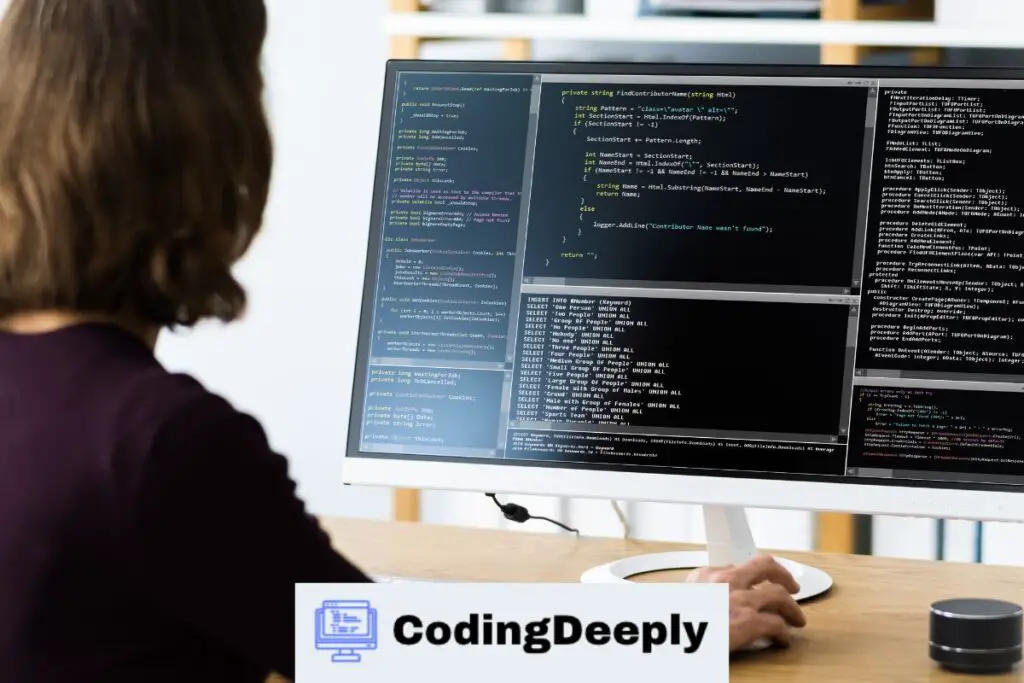
Understanding Python Dictionaries
Python dictionaries, or ‘dicts’ as they’re fondly called, are more than just a data type. They’re a programmer’s best friend. Here’s why:
- Unordered: Dictionaries are like the wild child of Python data types. They don’t care about order; they’re all about key-value pairs.
- Changeable: Dictionaries are mutable. You can add, modify, or delete key-value pairs on the go.
- Indexed: Dictionaries use keys as indexes. No more counting positions!
The Uniqueness of Keys in Python Dictionaries
In Python dictionaries, keys are like fingerprints – unique and distinct. No two keys are the same. This uniqueness is what makes dictionaries so powerful and flexible.
The Flexibility of Python Dictionary Keys
Python dictionary keys are like chameleons. They can be almost any data type:
- Strings: ‘Hello’ could be a key.
- Integers: 123 could be key.
- Tuples: (‘Hello’, 123) could be key.
Python Dictionaries with Integer Keys
Now, let’s talk about Python dictionaries with integer keys. They’re like the secret weapon of Python programming. Here’s why:
- Efficiency: Integer keys can make your code run faster.
- Simplicity: Integer keys can make your code easier to understand.
- Flexibility: Integer keys can make your code more versatile.
Stay tuned as we dive deeper into the practical aspects of Python dictionaries with integer keys in the next section. You’re in for a treat!
Practical Examples with Tips and Best Practices
Ready to create your first Python dictionary with integer keys?
Let’s get our hands dirty with some code.
Here’s a simple dictionary:
my_dict = {1: 'apple', 2: 'banana', 3: 'cherry'} print(my_dict)
Running this code will give you:
{1: 'apple', 2: 'banana', 3: 'cherry'}
Voila! You’ve just created a Python dictionary with integer keys.
Manipulating Python Dictionaries with Integer Keys
Now that you’ve created a dictionary, let’s play around with it. Here’s how you can add, modify, and delete key-value pairs:
- Adding a key-value pair:
my_dict[4] = 'date' print(my_dict)
- Modifying a key-value pair:
my_dict[1] = 'avocado' print(my_dict)
- Deleting a key-value pair:
del my_dict[2] print(my_dict)
Accessing Values in a Python Dictionary with Integer Keys
Accessing values in a Python dictionary with integer keys is a piece of cake.
Here’s how you do it:
print(my_dict[1])
This will print ‘avocado’.
Best Practices when Using Python Dictionaries with Integer Keys
Now that you’re a pro at using Python dictionaries with integer keys, let’s talk about some best practices:
- Use clear, descriptive keys: This makes your code easier to read and understand.
- Avoid using mutable data types as keys: This can lead to unexpected behavior.
- Use the
get()
method to access values: This prevents errors if the key doesn’t exist.
Stay tuned for the next section, where we’ll tackle potential problems and their solutions. You’re doing great, keep going!
Potential Problems and Their Solutions
Common Mistakes and How to Avoid Them
Even the best programmers make mistakes. But don’t worry. We’ve got your back. Here are some common mistakes when using Python dictionaries with integer keys:
- Using mutable data types as keys: Stick to using immutable data types, like integers.
- Forgetting that keys are case sensitive: ‘Key’ and ‘key’ are two different keys.
- Assuming dictionaries are ordered: Remember, dictionaries are unordered.
Troubleshooting Python Dictionaries with Integer Keys
Need help with a problem? Don’t fret. Here’s how you can troubleshoot issues with Python dictionaries with integer keys:
- KeyError: This means the key you’re trying to access doesn’t exist. Use the get() method to avoid this error.
- TypeError: This means you’re using a mutable data type as a key. Switch to an immutable data type.
my_dict = {[1, 2]: 'apple'}
This will give you a TypeError. Instead, use a tuple:
my_dict = {(1, 2): 'apple'}
Frequently Asked Questions
Can I use floating-point numbers as keys in Python dictionaries?
Absolutely! Like integers, floating-point numbers are immutable, so they can be used as keys in Python dictionaries. However, using integers or strings as keys is generally recommended due to the precision issues with floating-point numbers.
What happens if I try to access a key that doesn’t exist in the dictionary?
If you try to access a key that doesn’t exist in the dictionary, Python will raise a KeyError. To avoid this, you can use the dictionary’s get() method, which returns None if the key doesn’t exist, or a default value that you can specify.
Can I use a Python dictionary to implement a graph data structure?
Yes, you can! Python dictionaries are a great way to implement graph data structures, especially with integer keys. The keys can represent the graph nodes, and the values can represent the edges.
How can I merge two Python dictionaries with integer keys?
You can merge two Python dictionaries using the update() method or the ** operator. Both methods will add the key-value pairs from the second dictionary to the first. If there are duplicate keys, the values from the second dictionary will overwrite the values in the first.
Are Python dictionaries thread-safe?
Python dictionaries are not thread-safe by default. Consider using a thread-safe alternative like collections if you need a Python dictionary in a multi-threaded program.ChainMap or collections.OrderedDict, or use a lock to synchronize access to the dictionary.
Conclusion
Congratulations! You’ve just mastered Python dictionaries with integer keys. You’ve learned the theory, seen them in action, and even learned how to troubleshoot common issues.
Remember, practice makes perfect. So, keep coding, keep experimenting, and keep learning. You’re on your way to becoming a Python pro!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.