As a developer, you know the importance of using the right tools and techniques to make your code efficient and effective.
One of the most powerful features of Python programming is its ability to work with dictionaries. Dictionaries allow storing data in key-value pairs, making it easy to organize and manipulate complex data sets.
In this article, we will explore how to master the Python dictionary dot notation for easy access and manipulation of data.
Whether you’re a new or experienced Python developer, learning about dot notation can greatly enhance your coding skills and make your work more efficient.
Let’s dive in and discover the power of Python dictionaries!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Understanding Python Dictionaries
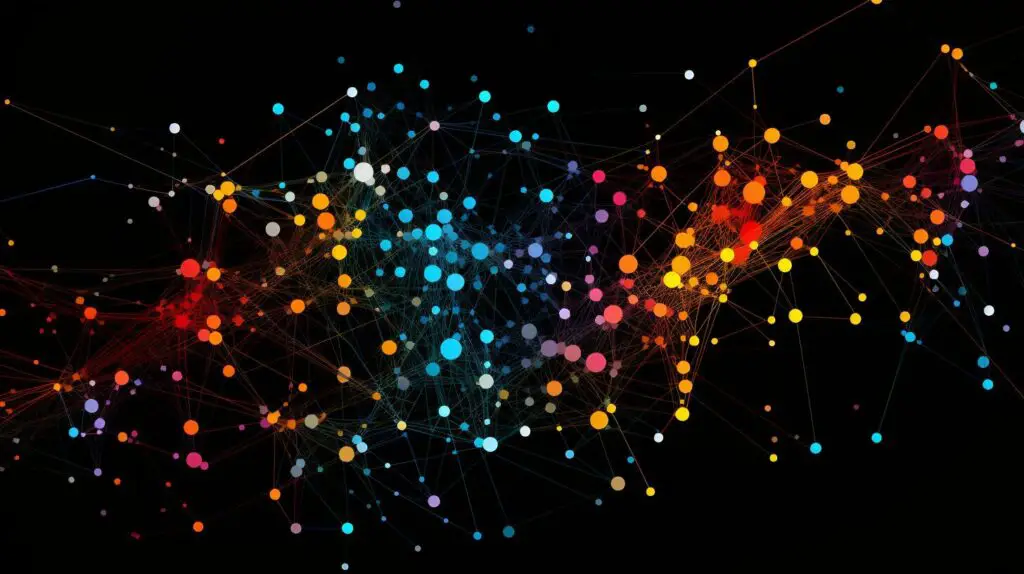
If you’re new to Python, you may wonder what a dictionary is and how it differs from other data types.
In simple terms, a dictionary is an unordered collection of related data in the form of key-value pairs. Each key in a dictionary is unique and maps to a specific data type value, such as integers, strings, or even other dictionaries.
Dictionaries are a powerful and versatile tool in Python, allowing you to store and manipulate data highly efficiently.
They offer a fast lookup time for values based on their associated keys, making them ideal for use in situations where you need to access data quickly and efficiently.
Traditional Dictionary Access
Before we dive into using the dot notation for Python dictionaries, let’s briefly review traditional dictionary access.
We use square brackets to access dictionary keys and their corresponding values in traditional dictionary access. For example, if we have a dictionary named ‘my_dict’ with the key-value pairs {‘name’: ‘John’, ‘age’: 30}, we would access the value associated with the ‘name’ key like this:
my_dict[‘name’]
The above code would return the string value ‘John’.
Similarly, we can set a new value for a key in the dictionary using:
my_dict[‘age’] = 31
This would change the value associated with the ‘age’ key from 30 to 31.
While traditional dictionary access is useful, it can become cumbersome when dealing with deeply nested dictionaries or when we want to access a key that doesn’t exist in the dictionary.
In the next section, we’ll introduce the dot notation as a more efficient way to access and manipulate Python dictionaries.
Introducing Dot Notation
Python dictionaries are a powerful and versatile data type, but traditional dictionary access can become tedious when dealing with nested structures. This is where dot notation comes in.
With dot notation, Python developers can access and manipulate nested dictionary values using a simple dot syntax, instead of using multiple bracket pairs for each level of nesting.
How Dot Notation Works
Dot notation replaces multiple bracket pairs with dots in a nested dictionary access. For example, instead of using:
my_dict['first_level']['second_level']['third_level']
you can use dot notation:
my_dict.first_level.second_level.third_level
Not only does this syntax make accessing and manipulating nested dictionary values more intuitive, but it also makes the code cleaner and easier to read.
Manipulating Dictionary Values with Dot Notation
Now that you understand how to access dictionary values using dot notation, it’s essential to learn how to manipulate these values to make changes to your dictionary. Let’s explore some ways to make changes to dictionary values using dot notation.
Changing Dictionary Values
If you want to change the value of a key in your dictionary, you can use dot notation to reassign the value. For example, let’s say you have a dictionary of employee information:
Key | Value |
---|---|
name | John |
age | 25 |
department | HR |
If you want to change John’s age to 26, you can do so using dot notation:
employee.age = 26
This will update the age value for the “employee” dictionary to 26.
Adding Dictionary Values
You can also use dot notation to add new key-value pairs to your dictionary. For example, let’s say you want to add an “email” key to the employee dictionary:
employee.email = “[email protected]”
This will add a new key-value pair to the employee dictionary:
Key | Value |
---|---|
name | John |
age | 26 |
department | HR |
[email protected] |
Deleting Dictionary Values
Finally, you can also delete key-value pairs from your dictionary using dot notation. For example, let’s say you want to delete the “department” key from the employee dictionary:
del employee.department
This will remove the “department” key and its corresponding value from the employee dictionary:
Key | Value |
---|---|
name | John |
age | 26 |
[email protected] |
As you can see, dot notation provides a simple and intuitive way to manipulate dictionary values in Python. With these skills, you can easily access, modify, and remove data from your dictionaries to suit your needs.
Best Practices for Using Dot Notation with Python Dictionaries
If you want to master the art of using Python dictionary dot notation for easy access and manipulation, it is essential to follow best practices. Here are some tips to help you use dot notation effectively:
- Use it only when necessary: Although dot notation is a powerful tool, it is crucial to use it only when it simplifies your code. If the dot notation makes your code unnecessarily complex, it is better to stick to traditional dictionary access.
- Ensure dictionary keys are valid: When using dot notation, make sure that the dictionary keys you are trying to access are valid Python identifiers. Otherwise, you’ll get a syntax error.
- Keep your code readable: To make your code more readable, use parentheses to group dot notation. This ensures that the order of operations is clear, and prevents confusion.
- Be mindful of performance: Although dot notation can make your code more readable, it may not always be the most efficient way to access dictionary values. In some cases, traditional dictionary access may be faster. Therefore, it’s important to balance readability and performance.
- Protect against key errors: As with traditional dictionary access, it’s crucial to protect against key errors when using dot notation. Use the ‘get’ method or try/except statements to handle missing keys.
Example:
The following example demonstrates how to use dot notation with Python dictionaries correctly:
Dictionary | Code | Output |
---|---|---|
{“name”: “John”, “age”: 30, “gender”: “male”} | name = person.get(‘name’) age = person.get(‘age’) gender = person.get(‘gender’, ‘unknown’) | John 30 male |
{“name”: “John”, “age”: 30, “gender”: “male”} | name = person.name age = person.age gender = person.gender | Error: ‘dict’ object has no attribute ‘name’ |
Note: In the first example, dot notation simplifies the code and makes it more readable. In the second example, dot notation results in an error because the keys are not valid Python identifiers.
By following these best practices, you can use Python dictionary dot notation effectively to make your code more readable and maintainable. However, remember to use it only when necessary, protect against key errors, and balance readability and performance.
Implementing Python Dictionary Dot Notation in Real-World Scenarios
Python dictionary dot notation can be applied in a wide range of real-world scenarios to improve the readability and efficiency of code. Let’s take a look at some examples:
“Using the dot notation has reduced the amount of time I spend looking up keys in my Python code. It’s also improved the readability of my code by making it easier to see which values I’m accessing.”
– Sarah, software developer
Here are some examples of how Python dictionary dot notation can be used:
Scenario | Example Code |
---|---|
Accessing nested values | person_info = {'name': {'first': 'John', 'last': 'Doe'}, 'age': 35} |
Updating dictionary values | person_info = {'name': {'first': 'John', 'last': 'Doe'}, 'age': 35} |
Creating new dictionary keys and values | person_info = {'name': {'first': 'John', 'last': 'Doe'}} |
As shown in the above examples, Python dictionary dot notation can greatly improve the readability and efficiency of code. However, it’s important to keep in mind some best practices for using dot notation with Python dictionaries.
Frequently Asked Questions about Python Dictionary Dot Notation
Here are some frequently asked questions about Python dictionary dot notation:
Q: What is Python dictionary dot notation?
A: Python dictionary dot notation is a way to access and manipulate values in a nested dictionary by using period “.” as a separator instead of square brackets “[]”.
Q: Why use dot notation instead of traditional dictionary access?
A: Dot notation provides a more concise and intuitive way to access and manipulate values in a nested dictionary. It also makes the code more readable and easier to maintain.
Q: Can dot notation be used with all types of dictionaries?
A: Dot notation can only be used with dictionaries that have keys which can be accessed using dot notation. For example, if a dictionary has keys that contain spaces or special characters, dot notation cannot be used.
Q: Are there any limitations to using dot notation?
A: Yes, there are some limitations to using dot notation. If the key contains a number or starts with a number, dot notation cannot be used. Additionally, if the key contains a hyphen or other special character, dot notation cannot be used.
Q: Is dot notation faster than traditional dictionary access?
A: The performance of dot notation and traditional dictionary access is similar. However, dot notation can improve code readability and reduce the likelihood of errors, which can ultimately save time in the long run.
Q: Can dot notation be used for nested dictionaries?
A: Yes, dot notation can be used for nested dictionaries. It provides an easy way to access and manipulate values in a nested dictionary without having to use multiple square brackets.
Q: What are some best practices for using dot notation with Python dictionaries?
A: Some best practices for using dot notation include using descriptive variable names, following a consistent naming convention, and using try-except blocks to handle possible errors. It is also important to ensure that the key names are valid and do not contain any special characters or spaces that cannot be used with dot notation.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.