Do you need help to create a list of consecutive integers in Python?
This simple task can become a real headache if you need to know the right approach.
But don’t worry…
This comprehensive guide will save you from common pitfalls and boost your coding efficiency. Here’s what you’ll discover:
- Theoretical foundations
- Practical examples and tips
- Problem-solving strategies
So, let’s dive in and turn this challenge into a piece of cake!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
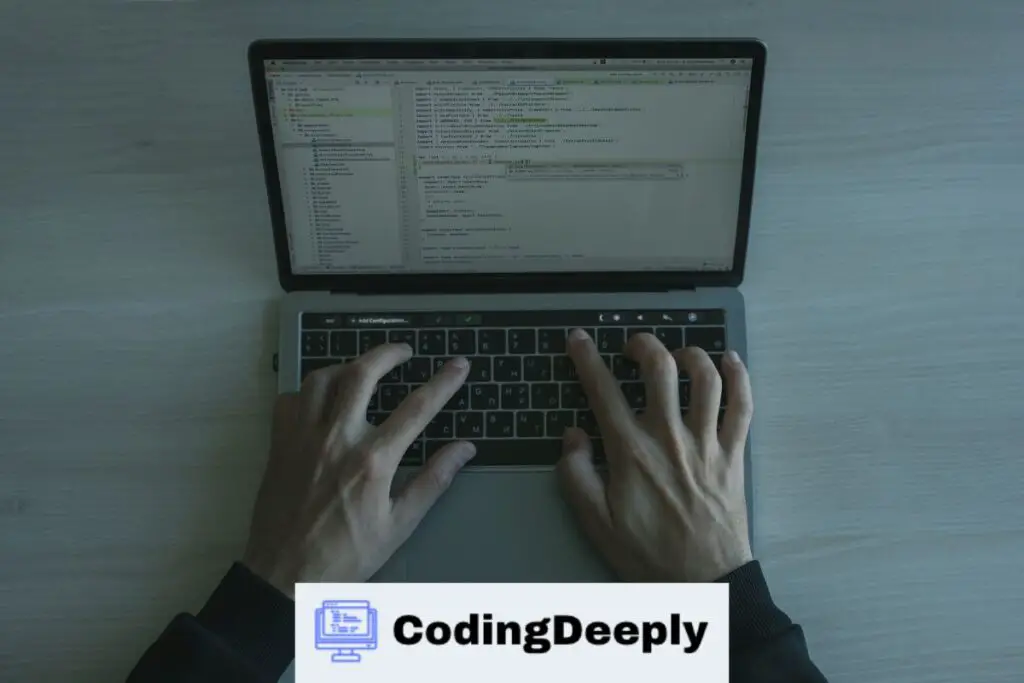
- Python lists and the range() function are essential for creating consecutive integers.
- List comprehension provides a Pythonic and efficient way to create lists.
- Custom step sizes in range() allow for flexible list creation.
- Generators can efficiently handle large lists of consecutive integers.
- Practical strategies can help manage memory errors and control large lists.
Theoretical Foundations
Understanding Python Lists
Let’s kick things off by getting to grips with Python lists. These are more than just a collection of items. They’re a dynamic, versatile tool that can simplify your coding life. Here’s why:
- They’re mutable: You can change them on the fly.
- They’re ordered: The order of items matters.
- They’re flexible: They can hold different types of data.
Understanding Consecutive Integers
Next up, consecutive integers. These are numbers that follow each other in order without any gaps. For example, 1, 2, 3, and so on. They’re a common sight in programming and data analysis, helping you to:
- Iterate over data
- Generate sequences
- Perform calculations
Python’s Built-in Functions for List and Range Manipulation
Python has two built-in functions that will be your best friends when creating lists of consecutive integers: list() and range(). Here’s a quick rundown:
- list(): This function creates a new list. Simple as that.
- range(): This function generates a sequence of numbers, which is perfect for creating consecutive integers.
Practical Examples and Tips
Creating a List of Consecutive Integers Using ‘range()’
Now, let’s put theory into practice. To create a list of consecutive integers using range(), follow these steps:
- Call range() with your start and end numbers.
- Wrap it with list() to convert the range to a list.
Here’s how it looks in the code:
numbers = list(range(1, 11)) print(numbers)
This will print the numbers 1 to 10. Easy.
Creating a List of Consecutive Integers with List Comprehension
But there’s another way: list comprehension. This is a more Pythonic way to create lists and is super efficient. Here’s how you do it:
numbers = [i for i in range(1, 11)] print(numbers)
This will give you the same result as before but with less code. Neat!
Tips for Efficient List Creation and Manipulation
Finally, let’s talk about efficiency. When working with lists in Python, keep these tips in mind:
- Use list comprehension for cleaner, faster code.
- Avoid adding items one by one. It’s slow!
- Use range() for large lists of consecutive integers.
And there you have it! You’re on your way to mastering the creation of lists of consecutive integers in Python. But don’t stop here. Keep reading to discover advanced techniques and how to solve common problems. Let’s keep the momentum going!
Advanced Techniques
Creating Lists of Consecutive Integers with Custom Step Sizes
Ready to level up your Python skills? Let’s talk about custom step sizes. This powerful feature of the range() function lets you control the “step” between consecutive integers. Here’s how it works:
numbers = list(range(1, 20, 2)) print(numbers)
This will print all odd numbers from 1 to 19. The 2 is the step size, which means “skip every second number.” You can set it to any value you like.
Using Generators for Large Lists of Consecutive Integers
When dealing with large lists, memory can become an issue. That’s where generators come in. They’re iterable, like lists, but they generate values on the fly, which saves memory. Here’s how you can use a generator to create a large list of consecutive integers:
numbers = (i for i in range(1, 1000001))
This creates a generator that generates numbers from 1 to 1,000,000. You can loop over it just like a list, but it will take up little memory.
Common Problems and Solutions
Dealing with Memory Errors
Speaking of memory, let’s talk about how to handle memory errors. These can occur when you’re working with very large lists. Here are some strategies to deal with them:
- Use generators instead of lists.
- Use the range() function directly.
- Split your data into smaller chunks.
Handling Large Lists of Consecutive Integers
Working with large lists can be challenging, but don’t let that scare you. Here are some tips for handling large lists of consecutive integers:
- Use efficient algorithms and data structures.
- Use Python’s built-in functions whenever possible.
- Feel free to ask for help. The Python community is full of people who have faced the same challenges and are more than willing to help.
Wrapping Up
And there you have it! You’ve journeyed through the ins and outs of creating lists of consecutive integers in Python. From understanding the basics to tackling advanced techniques, you’ve covered everything.
Remember these key takeaways:
- Python lists and the range() function are your best friends.
- List comprehension is a neat, Pythonic way to create lists.
- Generators are a lifesaver for handling large lists.
But don’t stop here. Keep exploring, coding, and pushing your Python skills to new heights. The programming world is full of exciting challenges; you’re ready to take them on. So, fire up your Python editor, and start creating!
Frequently Asked Questions
1. Can I create a list of consecutive integers in reverse order?
Absolutely! Python’s range() function allows you to create a list of integers in reverse order. You need to specify the start number, end number (typically, this would be zero), and a step size of -1. Here’s an example:
numbers = list(range(10, 0, -1)) print(numbers)
This will print the numbers from 10 to 1 in descending order.
2. How can I add a list of consecutive integers to an existing list?
You can extend an existing list with a list of consecutive integers using the extend() method. Here’s how:
numbers = [0] numbers.extend(range(1, 11)) print(numbers)
This will add the numbers from 1 to 10 to the existing list.
3. Can I create a list of consecutive floating-point numbers?
Python’s range() function only works with integers. However, you can create a list of consecutive floating-point numbers using a combination of the numpy library’s arrange () function and list comprehension. Here’s an example:
import numpy as np numbers = [float(i) for i in np.arange(0.0, 1.0, 0.1)] print(numbers)
This will print the numbers from 0.0 to 0.9 with a step size 0.1.
4. How can I create a list of consecutive integers with a custom function?
You can create a custom function to generate a list of consecutive integers. This can be useful if you add complex logic not covered by the range() function. Here’s a simple example:
def custom_range(start, end): return [i for i in range(start, end+1)] numbers = custom_range(1, 10) print(numbers)
This will print the numbers from 1 to 10.
5. Can I create a list of consecutive negative integers?
You can create a list of consecutive negative integers using the range() function. Just remember to make the start number larger than the end number and use a step size of -1. Here’s an example:
pythonCopy code
numbers = list(range(-1, -11, -1)) print(numbers)
This will print the numbers from -1 to -10.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.