Hey there, Python enthusiast! Feel like you’re coding in circles, your programs running slower than a snail on vacation?
Time to put the brakes on those bad habits and turbo-charge your Python skills.
This guide will keep you from stumbling into the common pitfalls of Python’s Counter, and by the end, you’ll be writing code that’s quicker than Usain Bolt. Ready to dive in?
Expect to discover:
- The magic of Python Counter.
- Unveiling time complexity.
- Boosting code efficiency.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
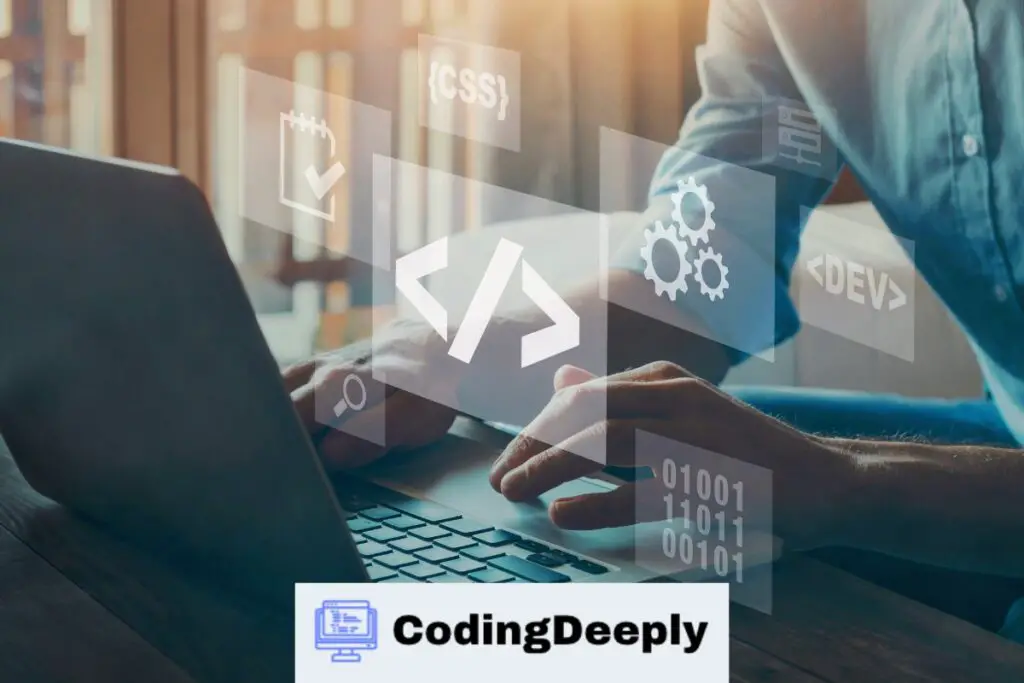
Here are the key takeaways from our exploration of Python Counter:
- Python Counter is a powerful tool for counting hashable objects efficiently.
- Understanding time complexity helps optimize code performance when using Python Counter.
- Python Counter has a time complexity of O(1) for element access and O(n) for creation.
- Python Counter finds applications in data analysis, text processing, and building efficient programs.
- Advanced features like the
elements()
andsubtract()
methods provide additional functionality.
Remember these points as you harness the power of Python Counter in your coding endeavors!
Dive into the World of Python Counter
Welcome aboard, fellow coder! Let’s start this journey by diving deep into the heart of the Python Counter.
What is Python Counter, anyway?
What’s this Python Counter, and why should you care? It’s a neat little tool in Python’s collections module.
Picture it as a smart dictionary that can help you count hashable objects or, in simple words, stuff that you can’t change once they’re created.
- Keep track of words in a text
- Tally scores in a game
- Count items in a list
See? It’s more useful than you thought!
Python Counter in Action
So, how does Python Counter get down to business? Let’s take a simple example. Say, you’ve got a basket full of fruits, and you want to know how many of each fruit type you have. All you need to do is feed your list of fruits to Python Counter, and voila! You have your tally.
from collections import Counter fruits = ['apple', 'banana', 'apple', 'orange', 'banana', 'banana'] fruit_counter = Counter(fruits) print(fruit_counter)
And there you have it! The script spits out a dictionary with each type of fruit and its count.
Look, Python Counter is your friend in need. When you’re scratching your head counting elements in a list, tuple, or sentence,
Python Counter saves the day. It’s a handy tool that takes your raw data and transforms it into something meaningful.
Now that you’re pals with Python Counter let’s dive deeper into its time complexity in the next chapter.
Tick-Tock on the Clock: Understanding Time Complexity
Right on! You’ve grasped Python Counter but ever wondered about the ticking clock behind it? Let’s pull back the curtain and look at time complexity.
Unraveling Time Complexity
Time complexity sounds like a buzzword from a sci-fi movie, but it’s more intimidating than it sounds. In a nutshell, it’s a way to measure how efficient your code is. It’s about how your code’s run-time increases with the input size.
Think of it this way:
- Got a small task? The code runs swiftly.
- Got a big job? The code might take its sweet time.
Understanding time complexity helps us write better, faster code, avoiding those awkward pauses when waiting for a result.
The ABCs of Time Complexity
Let’s break it down a bit more. In algorithms, the time complexity is often represented by big O notation. Don’t let those fancy terms scare you off; it’s just a way to describe how well an algorithm scales as the number of data increases.
- O(1): It doesn’t matter how big your task is; the time taken is constant. Now, that’s efficient!
- O(n): The time taken increases linearly with the task size.
- O(n²): The time taken multiplies for every new element in your task. Watch out!
Knowing these basics of time complexity, you’ll code like a pro, making smart choices that keep your programs swift and smooth.
Next, we’ll delve into the time complexity of our good old friend, Python Counter.
The Clock Ticks: Time Complexity of Python Counter
Nice! You’ve got Python Counter and time complexity down. Now, it’s time for the big reveal—how do these two play together?
How Quick is Python Counter?
So, you’re ready to race with Python Counter. But what’s the time complexity here? The good news is that creating a Python Counter from a list is an O(n) operation. That means the time taken is proportionate for every new element in your inventory.
And are you accessing the elements? It’s a dream run with an O(1) operation!
Here’s a quick recap:
- Creating a Counter: O(n)
- Accessing elements: O(1)
Isn’t that impressive?
Why Does Time Complexity Matter in Python Counter?
Great, Python Counter is fast. But why does this matter? Well, the time complexity is a deal-breaker in large-scale data analysis.
Whether counting votes in an election or analyzing sentiments on social media, Python Counter helps you handle heaps of data in a snap!
Here’s the kicker. The time complexity doesn’t just make your programs faster; it also helps to save resources. Remember, efficient code is the first step towards sustainable coding.
Ready to apply all this knowledge to real-life problems? Don’t worry; we’ve got you covered. Up next, we’re talking about how to use Python Counter to make your coding life easier. Gear up, coding wizards!
Conquer with Counter: Python Counter in Your Next Project
Alright, you’re almost a Python Counter pro now. But how do you implement it in your next project?
Let’s dive into the practical world of coding!
Data Analysis with Python Counter
Data is the new oil, and Python Counter is your drilling rig! It’s time to unearth insights from your data. With its swift performance and low time complexity, Python Counter can easily handle big data tasks.
Remember, counting occurrences is often the first step in data analysis. So, whether it’s social media analytics or market research, Python Counter has your back!
Text Processing Like a Pro
Have you ever wondered how text-based recommendation systems work? Or how do spam emails get detected?
Well, they all start with counting words. And there’s no better tool for this job than Python Counter. Count the frequency of words in a document, and use this data to feed your machine learning models.
Cool, right?
Building Fast and Efficient Programs
We’ve said it before, and I’ll say it again—time complexity matters!
With Python Counter’s stellar time complexity, you can write faster and more efficient programs. Say goodbye to nested loops and manual counting. Python Counter is here to save the day!
That’s it, folks! Python Counter is a mighty tool in your coding arsenal. Fast, efficient, and practical—it ticks all the boxes. So, what are you waiting for?
Jump in, start coding, and let Python Counter make your life easier!
Unleashing the Power: The Mighty Python Counter Library
Now that you’ve grown fond of the Python Counter library and its workings let’s put that knowledge to practice. It’s time to harness the untapped potential of this library, and here’s how you do it.
Use Cases of the Python Counter
You’ve learned the theory, but theory without practice is like a car without fuel. Let’s fuel up your programming skills with these practical applications:
- Frequency Count
- Top-N Items
- Arithmetic Operations
Frequency Count
In data science, counting the frequency of items in a list is a common operation. With the Python Counter, it’s as easy as pie! You won’t need to write elaborate loops, just a simple, one-liner command: Counter(your_list). Neat.
Top-N Items
Want to find the most common items in your data? Python Counter has got your back! The most common () method is your buddy here, saving you time and lines of code.
Arithmetic Operations
Bet you didn’t know this! The Python Counter can perform arithmetic operations like addition, subtraction, intersection, and union on two counter objects. It’s not just a counter; it’s a Swiss Army knife in the Python world!
The Hidden Gems: Advanced Uses of Python Counter
In the previous sections, you have been introduced to the fundamentals and some practical applications of the Python Counter class. Now, let’s delve into the more advanced uses.
A Glimpse into the Toolbox: Counter Methods
The Python Counter comes loaded with numerous methods to ease your tasks:
- elements()
- subtract()
The elements() method
The elements()
method, a lesser-known feature, provides an iterator over elements repeating each as many times as its count. To illustrate this, consider you have a Counter c = Counter(a=3, b=2)
. Calling list(c.elements())
would give you ['a', 'a', 'a', 'b', 'b']
.
The subtract() method
The subtract()
method, another useful tool, can deduct elements from Counter. It’s like your personal calculator for data. If c = Counter(a=3, b=2)
and d = Counter(a=1, b=2)
, running c.subtract(d)
modifies c
to Counter({'a': 2})
.
Conclusion and Key Takeaways
The Python Counter, though simple at first glance, harbors many advanced features that can help optimize your coding routines. It’s more than just a counting tool; it’s a powerful ally in your data manipulation tasks.
From basic frequency counting to performing arithmetic operations, from retrieving the most common elements to iterating over elements based on their counts, the Python Counter has got you covered.
It’s like your personal toolbox for handling collections.
Remember to keep exploring, experimenting, and learning as you continue your coding journey. Who knows what other coding gems you might uncover? Remember, practice is the key to mastery.
So, why wait? Get started, apply these concepts, and watch your coding prowess grow.
Conclusion and Parting Thoughts
By now, you must have realized that Python’s Counter is a powerful tool that can help you easily tackle complex problems.
The Counter class’s time complexity is a boon for large datasets, enabling you to process data faster and more efficiently. Remember, the key to mastering any tool is practice. So, flex your coding muscles, and let Python Counter make your life easier!
And hey, remember to share this knowledge with your peers. After all, learning is better when it’s shared.
Happy coding 😎.
Frequently Asked Questions (FAQ)
Can I use Python Counter for non-hashable objects?
No, Python Counter is designed to work with hashable objects. Hashable objects have a hash value that remains constant throughout their lifetime integer strings and tuples. However, if you have non-hashable objects like lists or sets, you can convert them to hashable objects before using Python Counter.
For example, you can convert a list to a tuple using the tuple() function and then pass it to the Counter. Remember that the conversion process might affect the uniqueness and order of the elements.
What is the difference between Python Counter and a regular dictionary?
Python Counter and a regular dictionary seem similar but have distinct purposes. The Counter class is a specialized subclass of the dictionary class, primarily used for counting hashable objects.
While dictionaries store key-value pairs, Counters store elements as keys and their counts as values. Counters also provide additional methods specifically tailored for counting, such as most_common() for retrieving the most common elements.
So, if you need to count the frequency of elements or perform counting-related operations efficiently, Python Counter is the way to go.
Can I modify the count of an element in a Python Counter?
Yes, you can modify the count of an element in a Python Counter by directly assigning a new value to it.
For example, if you have a Counter c with elements {‘apple’: 2, ‘banana’: 3}, and you want to change the count of ‘apple’ to 5, you can do c[‘apple’] = 5. This will update the count of ‘apple’ to 5 on the Counter.
Keep in mind that if you assign a count of 0 to an element, it will effectively remove that element from the Counter.
Are there any limitations to the data size that Python Counter can handle?
Python Counter can handle large amounts of data efficiently. However, remember that the available memory on your system can limit the size of data you can work with.
You might encounter memory-related issues when working with large datasets if you need more memory resources. In such cases, you can consider using alternative techniques like streaming or batching your data to process it in smaller chunks.
Optimizing your code and minimizing unnecessary memory usage can improve performance when working with large data sets.
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.