Ready to dive in and learn to ensure those pesky URLs are valid?
Let’s get started!
In this blog post, we’ll cover the ins and outs of URL validation, show you how to use Python libraries, and share some killer tips you can immediately put into action.
So, buckle up. We’re about to become URL validation experts together! 💪
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
Key Takeaways
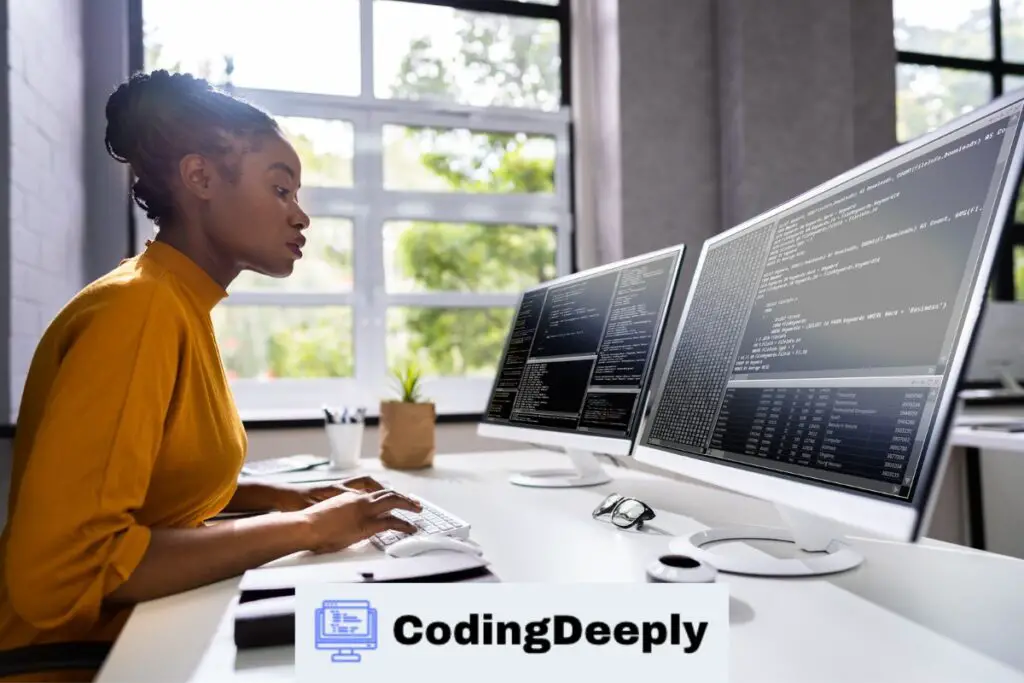
- Python offers built-in libraries like urllib.parse and the re module for URL validation, providing useful functions like urlparse, urlsplit, and regex patterns.
- urlparse and urlsplit in urllib.parse can be used to analyze and validate URLs by breaking them down into components.
- Regular expressions (regex) offer a powerful and flexible approach to URL validation, allowing custom patterns to match valid URLs.
- Third-party libraries like validators and tldextract provide additional options for URL validation with user-friendly functions and advanced analysis capabilities.
- Installing and using third-party libraries is easy with pip and a few lines of code, expanding your toolbox for tackling URL validation tasks.
Python’s Built-in Libraries for URL Validation
You don’t have to reinvent the wheel regarding URL validation in Python.
There are some fantastic built-in libraries just waiting for you to use them!
Let’s explore the two popular ones: urllib.parse and re.
urlparse and urlsplit in the urllib.parse module
The urllib.parse module is a treasure trove of useful URL functions.
Two of these handy tools are urlparse and urlsplit. They break down a URL into its components, making it easier to check if everything’s in the right place. 😎
Here’s a quick rundown of the differences between urlparse and urlsplit:
- urlparse separates a URL into six components: scheme, netloc, path, params, query, and fragment.
- urlsplit is similar but skips the params part, giving you only five components.
Both functions are super useful for URL validation, so it’s a personal preference regarding your choice.
Let’s move on to our next built-in library!
Using re (Regular Expression) module
Sometimes, you need more flexibility when validating URLs. Enter the re-module, Python’s way of handling regular expressions! 🎉
Regular expressions, or regex, are powerful patterns that match, find, and manipulate text. They’re like a secret code language for string manipulation. With the re-module, you can create custom patterns to check URLs, ensuring they fit your desired structure.
Here’s what you’ll love about using regex for URL validation:
- Flexibility: You can create patterns to match the URL format you need.
- Power: Regex can handle complex patterns and edge cases, making it a versatile tool for URL validation.
- Precision: You can fine-tune your regex patterns to capture specific URL parts, giving you more control over the validation process.
So, there you have it! Python’s built-in libraries, urllib.parse, and re, are ready to help you validate URLs like a pro.
It’s time to put these awesome tools to work and create some URL validation magic!
Ready to learn how to use urlparse and urlsplit for URL validation? Let’s jump right in and become masters of these great functions!
Introduction to urlparse and urlsplit functions
As we mentioned earlier, urlparse and urlsplit dissect URLs into their components.
By checking these components, we can determine whether a URL is valid. It’s like having a magnifying glass for URLs!
A step-by-step guide to using urlparse and urlsplit for URL validation
Here’s a simple guide to get you started with urlparse and urlsplit:
Import the functions: First, import the functions from the urllib.parse module:
from urllib.parse import urlparse, urlsplit
Choose your function: Pick urlparse or urlsplit, depending on your preference. Remember, urlparse gives you six components, while urlsplit provides five.
Dissect the URL: Call the chosen function and pass in the URL you want to validate. It’ll return an object with the URL components:
parsed_url = urlparse("https://www.example.com/path?query=value#fragment")
Check the components: Now, it’s time to examine the URL components. At a minimum, a valid URL should have a scheme (e.g., “http”) and a netloc (e.g., “www.example.com“). You can check these by accessing the object’s attributes:
if parsed_url.scheme and parsed_url.netloc: print("Valid URL!") else: print("Invalid URL!")
Code examples
Here’s a complete example using urlparse:
from urllib.parse import urlparse def is_valid_url(url): parsed_url = urlparse(url) return bool(parsed_url.scheme and parsed_url.netloc) url = "https://www.example.com/path?query=value#fragment" print("Valid URL!" if is_valid_url(url) else "Invalid URL!")
And here’s the same example using urlsplit:
from urllib.parse import urlsplit def is_valid_url(url): split_url = urlsplit(url) return bool(split_url.scheme and split_url.netloc) url = "https://www.example.com/path?query=value#fragment" print("Valid URL!" if is_valid_url(url) else "Invalid URL!")
Now you’re ready to conquer URL validation with urlparse and urlsplit! Keep practicing, and soon you’ll be a URL-validation superhero!
URL Validation with Regular Expressions
Regular expressions (regex) are like a superpower for text manipulation.
They’re a set of patterns that can match, search, or replace text in strings. Python’s re module lets you use regex like a pro.
Crafting a regex pattern for URL validation
To use regex for URL validation, you’ll need to create a pattern that matches valid URLs.
This pattern should include the different components of a URL, like a scheme, netloc, and path.
Don’t worry.
We’ve got you covered with a simple regex pattern for URL validation:
pattern = re.compile( r'^(?:http|ftp)s?://' # Scheme r'(?:(?:[A-Z0-9](?:[A-Z0-9-]{0,61}[A-Z0-9])?\.)+(?:[A-Z]{2,6}\.?|[A-Z0-9-]{2,}\.?)|' # Domain r'localhost|' # Localhost r'\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3})' # IP address r'(?::\d+)?' # Port (optional) r'(?:/?|[/?]\S+)$', re.IGNORECASE) # Path and query (optional)
A step-by-step guide to using regex for URL validation
Here’s how to use the re module and our regex pattern for URL validation:
Import the module: First, import the re module:
import re
Compile the pattern: Use re.compile() to compile the regex pattern. This helps improve performance when using the pattern multiple times:
pattern = re.compile("your_regex_pattern_here", re.IGNORECASE)
Match the URL: Use the match() method to check if the URL matches the regex pattern. It returns a match object if the URL is valid or None if it’s not:
if pattern.match("https://www.example.com"): print("Valid URL!") else: print("Invalid URL!")
Code examples
Here’s a complete example of URL validation with regex:
import re def is_valid_url(url): pattern = re.compile( r'^(?:http|ftp)s?://' r'(?:(?:[A-Z0-9](?:[A-Z0-9-]{0,61}[A-Z0-9])?\.)+(?:[A-Z]{2,6}\.?|[A-Z0-9-]{2,}\.?)|' r'localhost|' r'\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3})' r'(?::\d+)?' r'(?:/?|[/?]\S+)$', re.IGNORECASE) return bool(pattern.match(url)) url = "https://www.example.com/path?query=value#fragment" print("Valid URL!" if is_valid_url(url) else "Invalid URL!")
Congratulations, you’re now a regex wizard for URL validation!
Third-Party Libraries for URL Validation
Need more than built-in libraries?
No problem!
Let’s explore two great third-party libraries for URL validation: validators and tldextract.
They’ll make validating URLs as easy as pie!
validators library
validators is a super user-friendly library with an easy-to-use url() function. It returns True if the URL is valid and False if not.
Simple.
tldextract library
If you need more advanced URL validation, tldextract is here to save the day!
This library accurately extracts subdomain, domain, and suffix from URLs. You can use it to analyze and validate URLs with more granularity.
How to install and use third-party libraries
Installing and using these libraries is a piece of cake!
Follow these steps:
Install the library: Use pip to install the library:
pip install validators
or
pip install tldextract
Import the library: Import the library into your Python script:
import validators
or
import tldextract
Use the library: Call the library’s functions to validate URLs:
print(validators.url("https://www.example.com"))
or
extracted = tldextract.extract("https://www.example.com") print(extracted.domain) # Outputs 'example'
Conclusion
You’ve now mastered the art of URL validation in Python!
With built-in libraries, regex, and third-party libraries at your disposal, you’re all set to tackle any URL validation challenge. So, go ahead, put your newfound skills into action, and make your code more robust and error-free!
Happy coding! 😎
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.