Ever wondered if there’s a simple way to check if an object has a specific method in Python?
Well, you’re in the right place!
In this blog post, we’ll dive into some handy techniques to discover just that. We’ll explore Python’s built-in functions like hasattr() and getattr(), and even create our custom function.
So, let’s level up your Python skills together! 💪
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.
The Key Takeaways
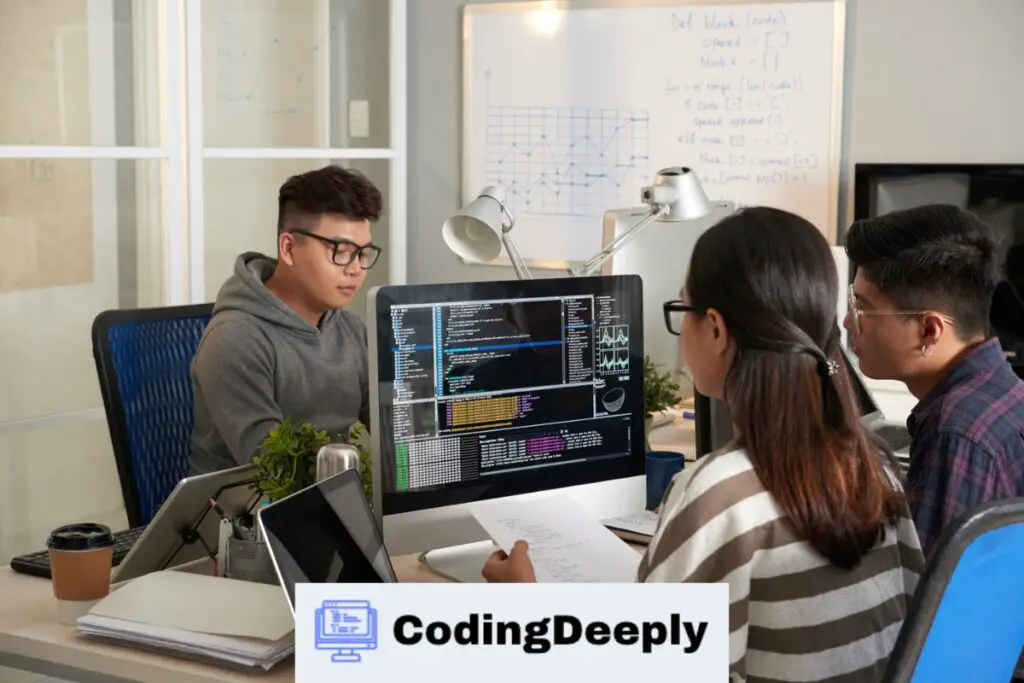
- Python objects and methods: Objects are instances of classes, and methods are functions defined within classes that can be called on objects.
- hasattr(): A built-in Python function that checks if an object has a specific attribute, such as a method, and returns a boolean value.
- getattr(): Another built-in function that checks if an object has a specific attribute and returns the attribute if it exists or a default value if it doesn’t.
- Custom function: You can create a custom function to check if an object has a method, making your code more readable and reusable.
- Practical applications: Method-checking techniques are useful in dynamic objects, third-party libraries, duck typing, plugin systems, and testing/debugging scenarios.
Python Objects: What are They?
First things first, let’s get the basics down!
Python objects are instances of classes containing data and methods that can act on that data.
Everything in Python is an object – from numbers and strings to functions and classes.
Methods: A Python Object’s Best Friend
Now, onto methods.
A method is a function bound to an object and can act on that object’s data.
It’s like a regular function but with a twist: it belongs to a class and can only be called through an instance. This is because methods are typically defined within classes and used to perform specific tasks related to the object.
The Dynamic Duo: Objects and Methods in Python
So, how do objects and methods work together in Python?
Think of objects as the actors in a play and methods as the actions they can perform.
When you create an object, it comes loaded with the methods defined in its class. You can call these methods on the object to modify its data or perform some action.
For example, let’s say we have a Dog class with a bark method:
class Dog: def bark(self): print("Woof! Woof!")
Now, we can create an instance of the Dog class and make it bark:
my_dog = Dog() my_dog.bark() # Output: Woof! Woof!
Here, my_dog is an object of the Dog class, and we call its bark method to make it bark!
That’s the magic of objects and methods in Python: They work together to help you create powerful, reusable code.
So, now that we’ve got the basics down let’s move on to checking if an object has a specific method…
Introducing the hasattr() Function
Let’s talk about the hasattr() function.
This built-in Python function helps you check if an object has a specific attribute, like a method or a property. It’s super useful when figuring out if an object has a certain method or if you’re working with dynamic objects.
Using hasattr() to Check for Methods
hasattr() takes two arguments: the object you’re checking and the name of the attribute (method or property) you’re looking for. It returns True if the object has the attribute and False otherwise.
Here’s an example:
class Dog: def bark(self): print("Woof! Woof!") my_dog = Dog() if hasattr(my_dog, 'bark'): print("This dog can bark!") else: print("This dog can't bark...")
In this example, hasattr() checks if the my_dog object has a bark method. It returns True, so the output will be “This dog can bark!” 🎉
More Examples with hasattr()
Now that you know how hasattr() works, let’s check out a couple more examples:
class Cat: def meow(self): print("Meow!") class Fish: def swim(self): print("I'm swimming!") my_cat = Cat() my_fish = Fish() # Check if my_cat has the meow method print(hasattr(my_cat, 'meow')) # Output: True # Check if my_fish has the swim method print(hasattr(my_fish, 'swim')) # Output: True # Check if my_fish can meow (spoiler: it can't!) print(hasattr(my_fish, 'meow')) # Output: False
And there you have it! hasattr() is your new best friend for checking if an object has a specific method or property. Give it a try, and have Fun experimenting! 🚀
Meet the getattr() Function
Hold tight because we’re introducing another Python gem: the getattr() function!
This handy built-in function checks if an object has a certain attribute and lets you access that attribute.
So, it’s like a super-charged version of hasattr()! 🚀
Checking and Accessing Methods with getattr()
To use getattr(), you’ll need to pass in three arguments: the object you’re checking, the name of the attribute you’re looking for, and a default value to return if the attribute isn’t found.
If the object has the attribute, getattr() will return it; otherwise, it returns the default value.
Here’s a quick example:
class Dog: def bark(self): print("Woof! Woof!") my_dog = Dog() bark_method = getattr(my_dog, 'bark', None) if bark_method: bark_method() # Output: Woof! Woof! else: print("This dog can't bark...")
In this case, getattr() checks if the my_dog object has a bark method. Since it does, getattr() returns the method, which we can call directly with bark_method().
More Fun with getattr()
Want more examples? You got it!
class Cat: def meow(self): print("Meow!") my_cat = Cat() # Check if my_cat has the meow method and call it meow_method = getattr(my_cat, 'meow', None) if meow_method: meow_method() # Output: Meow! # Check if my_cat has the purr method (it doesn't) purr_method = getattr(my_cat, 'purr', None) if purr_method: purr_method() else: print("This cat can't purr...") # Output: This cat can't purr...
And there you go!
Now you know how to use getattr() to check for and access methods in Python objects. Please give it a whirl and enjoy the power it brings to your code!
Why Create a Custom Function?
We’ve covered hasattr() and getattr() functions, but what if you want something more tailored to your needs?
That’s where custom functions come in!
By creating a custom function, you can make your code more readable and reusable, making your life as a programmer easier.
So, let’s build one for checking if an object has a method!
Crafting Your Method-Checking Function
Creating a custom function is a breeze!
Here’s a simple example of a custom function that checks if an object has a specific method:
def has_method(obj, method_name): return callable(getattr(obj, method_name, None))
This has_method() function takes an object and a method name as arguments, and it uses the callable() function to check if the attribute returned by getattr() is a method.
Putting Your Custom Function to Work
Now, let’s see our custom has_method() function in action:
class Dog: def bark(self): print("Woof! Woof!") class Fish: def swim(self): print("I'm swimming!") my_dog = Dog() my_fish = Fish() # Check if my_dog has the bark method print(has_method(my_dog, 'bark')) # Output: True # Check if my_fish has the swim method print(has_method(my_fish, 'swim')) # Output: True # Check if my_fish can bark (nope!) print(has_method(my_fish, 'bark')) # Output: False
And there you have it!
Your very own custom function for checking if an object has a method.
Go ahead, play around with it, and enjoy the added flexibility in your Python projects!
When to Use Method-Checking Techniques
When to use these great method-checking techniques? There are many practical scenarios where checking if an object has a method can be helpful. For example, when you’re working with:
- Dynamic objects whose methods might change at runtime
- Third-party libraries or APIs with evolving features
- Objects from different classes that share some methods
Now, let’s dive into some tips and examples for real-world applications!
Tips for Effective Method-Checking
- Keep it simple: Stick to hasattr() when you need to know if an object has a specific method, and use getattr() when you want to access it.
- Customize when needed: Create a custom function for more specific use cases or when you want to improve code readability.
- Write clean code: Always consider readability and maintainability when using method-checking techniques.
Real-World Examples
- Plugin systems: Method-checking can help you determine if a plugin provides certain functionality before calling its methods in applications that support plugins or extensions.
- Duck typing: Python encourages duck typing, which means you focus on what an object can do rather than its class. Checking if an object has a method can help you implement duck typing effectively.
- Testing and debugging: You can use method-checking to write better test cases or to debug your code by ensuring that objects have expected methods.
With these practical tips and examples, you can use method-checking techniques like a pro in your Python projects!
Wrapping It Up
Now you’ve mastered the art of checking if an object has a method in Python.
You can tackle any coding challenge, from using hasattr() and getattr() to crafting your custom functions.
So go forth and conquer your Python projects with confidence!
Advertising links are marked with *. We receive a small commission on sales, nothing changes for you.